Intro
Master wrapping text in VBA with ease. Learn how to automatically wrap text in Excel cells using VBA macros, formulas, and techniques. Discover how to resize cells, format text, and optimize data presentation. Improve your VBA skills and streamline your workflow with these expert tips and tricks for wrapping text in VBA.
Wrapping text in VBA can be a bit tricky, but with the right techniques, it can be done easily. In this article, we will explore the different methods of wrapping text in VBA, including using the WrapText
property, the TextWrap
property, and the ShapeRange
object.
Why Wrap Text in VBA?
Wrapping text in VBA is useful when you want to display a large amount of text in a small area, such as a cell or a shape. By wrapping the text, you can make it more readable and prevent it from spilling over into adjacent cells or shapes.
Method 1: Using the WrapText
Property
The WrapText
property is a built-in property in VBA that allows you to wrap text in a cell or range of cells. To use this property, you can simply set it to True
or False
depending on whether you want to wrap the text or not.
Sub WrapTextInCell()
Range("A1").WrapText = True
End Sub
This code will wrap the text in cell A1.
Method 2: Using the TextWrap
Property
The TextWrap
property is similar to the WrapText
property, but it is used for shapes and text boxes instead of cells. To use this property, you can simply set it to True
or False
depending on whether you want to wrap the text or not.
Sub WrapTextInShape()
ActiveSheet.Shapes("Shape1").TextFrame.TextRange.TextWrap = True
End Sub
This code will wrap the text in the shape named "Shape1".
Method 3: Using the ShapeRange
Object
The ShapeRange
object is a powerful object in VBA that allows you to manipulate shapes and text boxes. To wrap text using the ShapeRange
object, you can use the WrapText
method.
Sub WrapTextInShapeRange()
Dim shp As Shape
Set shp = ActiveSheet.Shapes("Shape1")
shp.TextFrame.TextRange.WrapText True
End Sub
This code will wrap the text in the shape named "Shape1".
Example Use Cases
Here are some example use cases for wrapping text in VBA:
- Wrapping text in a cell or range of cells to make it more readable.
- Wrapping text in a shape or text box to prevent it from spilling over into adjacent cells or shapes.
- Creating a table of contents with wrapped text to make it more readable.
Best Practices
Here are some best practices for wrapping text in VBA:
- Use the
WrapText
property for cells and ranges, and theTextWrap
property for shapes and text boxes. - Use the
ShapeRange
object for more complex text wrapping tasks. - Test your code thoroughly to ensure that the text is wrapping correctly.
Common Errors
Here are some common errors that you may encounter when wrapping text in VBA:
- Error 1004: "Method 'WrapText' of object 'Range' failed". This error occurs when you try to wrap text in a range that does not support text wrapping.
- Error 438: "Object doesn't support this property or method". This error occurs when you try to use the
WrapText
property orTextWrap
property on an object that does not support it.
Conclusion
Wrapping text in VBA is a useful technique that can make your text more readable and prevent it from spilling over into adjacent cells or shapes. By using the WrapText
property, the TextWrap
property, or the ShapeRange
object, you can easily wrap text in VBA. Remember to test your code thoroughly and follow best practices to avoid common errors.
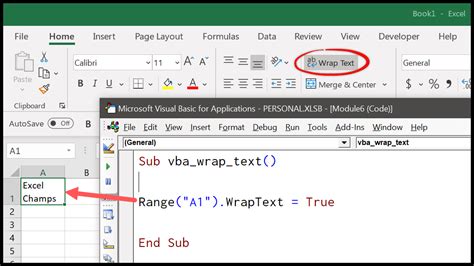
Gallery of VBA Text Wrapping
VBA Text Wrapping Image Gallery
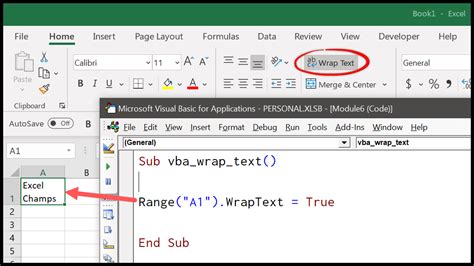
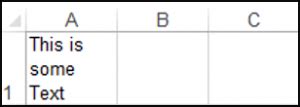
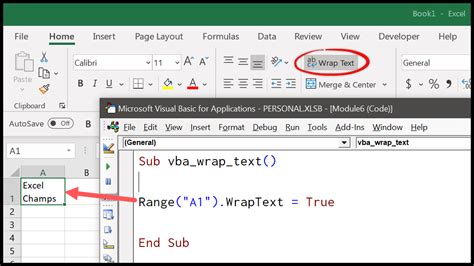
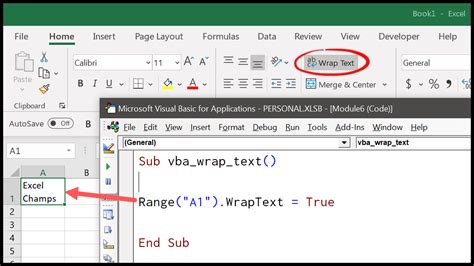
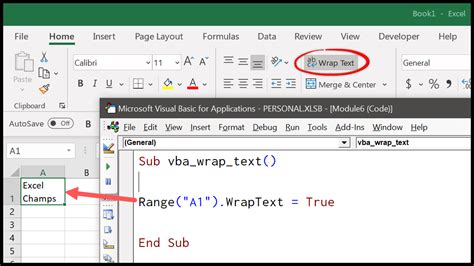
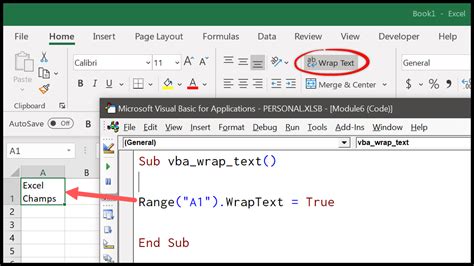
FAQ
- Q: How do I wrap text in VBA?
A: You can wrap text in VBA using the
WrapText
property, theTextWrap
property, or theShapeRange
object. - Q: What is the difference between the
WrapText
property and theTextWrap
property? A: TheWrapText
property is used for cells and ranges, while theTextWrap
property is used for shapes and text boxes. - Q: How do I use the
ShapeRange
object to wrap text? A: You can use theShapeRange
object to wrap text by setting theWrapText
method toTrue
.