Intro
Master Excel VBA with our step-by-step guide on how to copy a range to another worksheet. Learn the easy way to duplicate data, formulas, and formatting using VBA code. Discover the power of worksheet loops, range objects, and paste special methods to streamline your workflow. Boost productivity and accuracy with our expert tips.
Copying a range from one worksheet to another is a fundamental operation in Excel VBA. Whether you're automating tasks, building reports, or creating dashboards, you'll frequently need to transfer data between worksheets. In this article, we'll delve into the world of Excel VBA and explore how to copy ranges between worksheets with ease.
Understanding the Basics
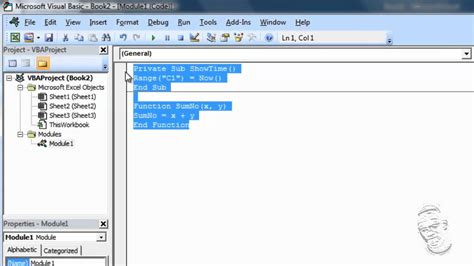
Before we dive into the nitty-gritty of copying ranges, it's essential to understand the basics of Excel VBA. VBA (Visual Basic for Applications) is a programming language used to create and automate tasks in Microsoft Office applications, including Excel. Excel VBA is a powerful tool that allows you to automate repetitive tasks, create custom functions, and interact with other Office applications.
Declaring Variables and Worksheets
To copy a range from one worksheet to another, you'll need to declare variables and worksheets. In VBA, you can declare variables using the Dim
statement. For example:
Dim sourceWorksheet As Worksheet
Dim targetWorksheet As Worksheet
You can then set the sourceWorksheet
and targetWorksheet
variables to the desired worksheets using the Set
statement:
Set sourceWorksheet = ThisWorkbook.Worksheets("Sheet1")
Set targetWorksheet = ThisWorkbook.Worksheets("Sheet2")
Copying a Range using the `Range` Object
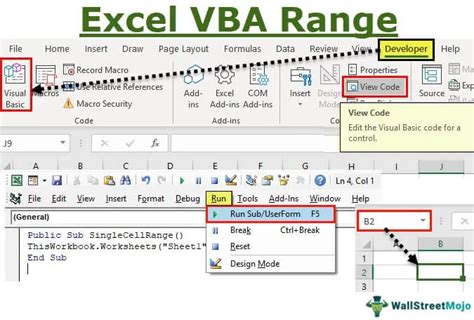
The Range
object is a fundamental object in Excel VBA that represents a cell or a range of cells. You can use the Range
object to copy a range from one worksheet to another using the Copy
method. Here's an example:
sourceWorksheet.Range("A1:B2").Copy targetWorksheet.Range("C3:D4")
This code copies the range A1:B2
from the sourceWorksheet
to the range C3:D4
on the targetWorksheet
.
Copying a Range using the `Cells` Property
Alternatively, you can use the Cells
property to copy a range. The Cells
property returns a Range
object that represents a single cell or a range of cells. Here's an example:
sourceWorksheet.Cells(1, 1).Resize(2, 2).Copy targetWorksheet.Cells(3, 3).Resize(2, 2)
This code copies the range A1:B2
from the sourceWorksheet
to the range C3:D4
on the targetWorksheet
.
Coping with Errors and Exceptions
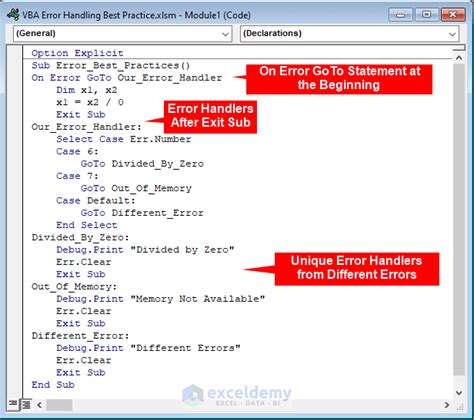
When copying ranges between worksheets, you may encounter errors or exceptions. For example, the target worksheet may not exist, or the range may be invalid. To handle these errors, you can use VBA's built-in error handling mechanisms, such as On Error Resume Next
or On Error GoTo 0
.
Here's an example of error handling:
On Error Resume Next
sourceWorksheet.Range("A1:B2").Copy targetWorksheet.Range("C3:D4")
If Err.Number <> 0 Then
MsgBox "Error copying range: " & Err.Description
End If
On Error GoTo 0
This code attempts to copy the range and displays an error message if an error occurs.
Best Practices and Optimization
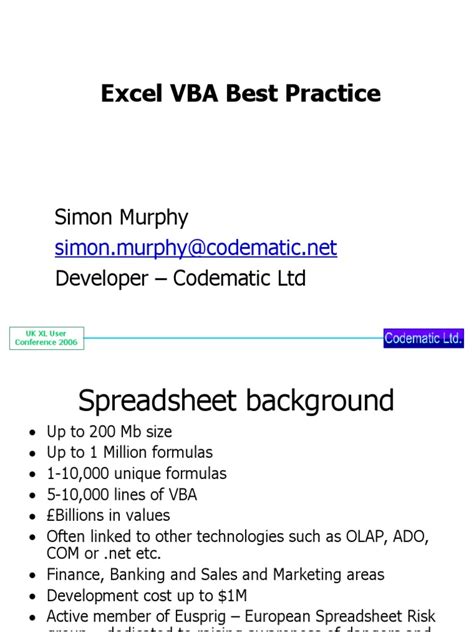
To optimize your VBA code and follow best practices, consider the following tips:
- Use meaningful variable names and comments to explain your code.
- Avoid using
Select
andActivate
methods, which can slow down your code. - Use
Range
objects instead ofCells
property to improve performance. - Handle errors and exceptions using VBA's built-in mechanisms.
- Test your code thoroughly to ensure it works as expected.
By following these tips and best practices, you can write efficient and effective VBA code to copy ranges between worksheets.
Conclusion
Copying a range from one worksheet to another is a fundamental operation in Excel VBA. By understanding the basics of VBA, declaring variables and worksheets, and using the Range
object or Cells
property, you can write efficient and effective code to copy ranges. Remember to handle errors and exceptions, follow best practices, and optimize your code for performance.
Excel VBA Image Gallery
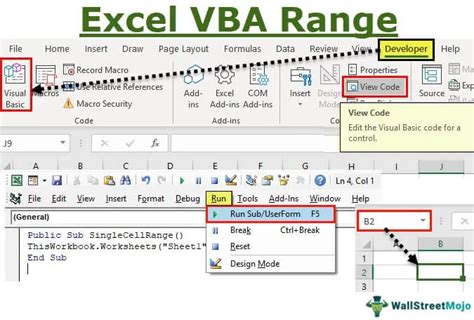
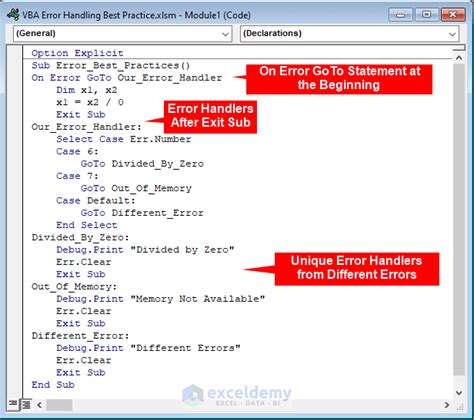
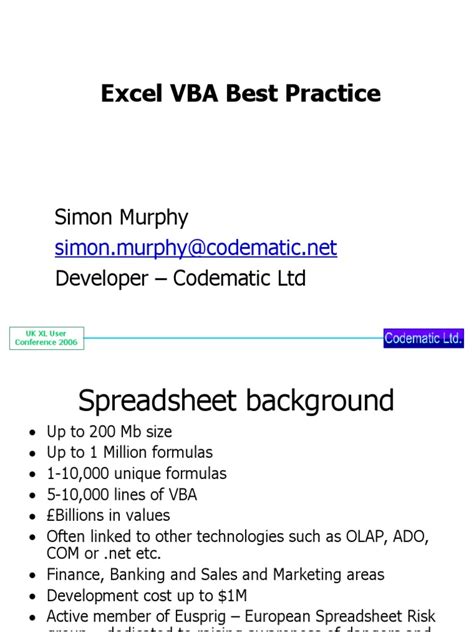
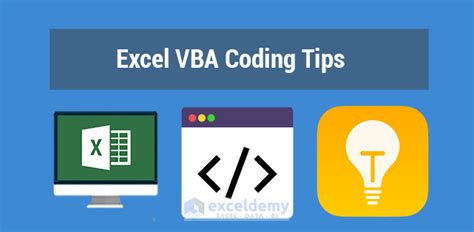
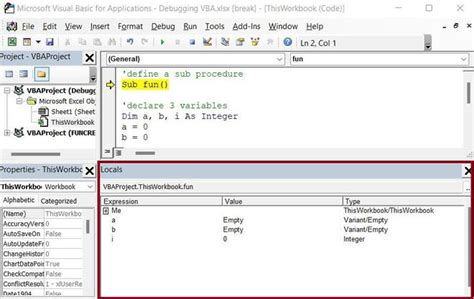
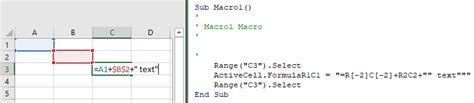
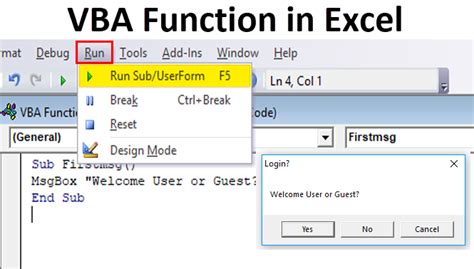
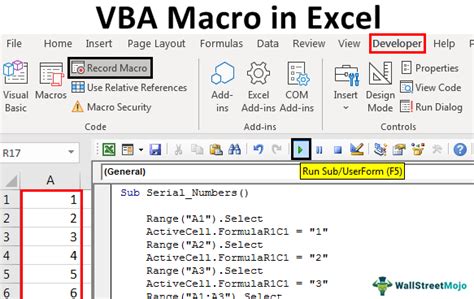
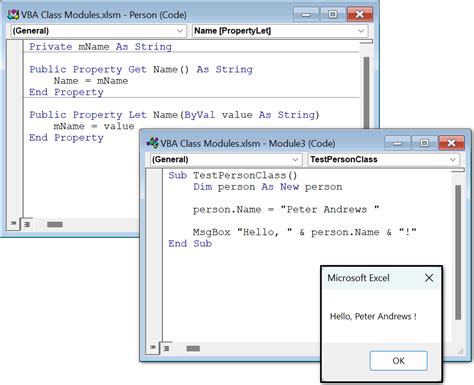
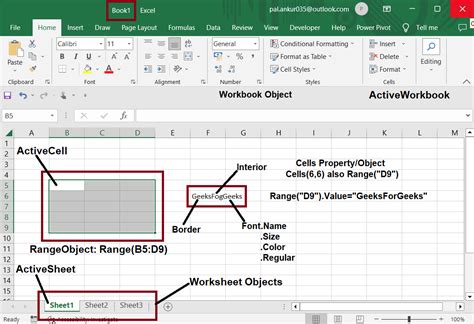
We hope this article has helped you learn how to copy ranges between worksheets in Excel VBA. If you have any questions or need further assistance, please don't hesitate to ask. Share your thoughts and experiences in the comments below, and don't forget to follow us for more Excel VBA tutorials and tips!