Intro
Master paste values in Excel VBA with ease! Learn how to efficiently paste values without formulas or formatting using VBA codes. Discover techniques for range copying, worksheet looping, and error handling. Optimize your Excel automation with expert tips and tricks, and streamline your workflow with VBA paste values made simple.
In the world of Excel VBA, working with data is a fundamental task. One of the most common operations is pasting values into a worksheet. While it may seem straightforward, there are several ways to achieve this in VBA, and the approach you take can significantly impact performance and accuracy. In this article, we will explore the different methods for pasting values in Excel VBA, discuss their pros and cons, and provide examples to make your coding experience smoother.
Understanding the Basics of Pasting Values in Excel VBA
Before diving into the methods, it's essential to understand how VBA interacts with Excel. When you record a macro, Excel generates code that replicates your actions. However, this code might not always be the most efficient or reliable way to achieve the desired result. In the case of pasting values, the default recorded macro code often uses the PasteSpecial
method, which can be slow and may not work as expected in all situations.
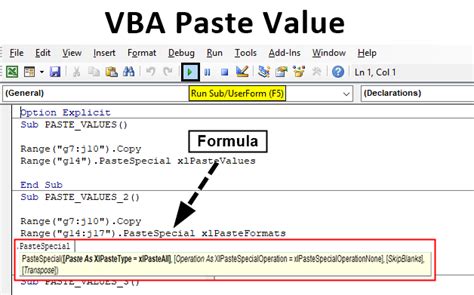
Method 1: Using the PasteSpecial
Method
The most common approach to pasting values in Excel VBA is using the PasteSpecial
method. This method allows you to specify the paste type, such as values, formulas, or formatting. Here's an example:
Sub PasteValuesUsingPasteSpecial()
Range("A1").Copy
Range("B1").PasteSpecial xlPasteValues
Application.CutCopyMode = False
End Sub
While this method works, it has some drawbacks. It can be slow, especially when working with large datasets, and it may not preserve the original formatting.
Method 2: Assigning Values Directly
A more efficient way to paste values in Excel VBA is by assigning the values directly. This approach eliminates the need for copying and pasting, making it faster and more reliable. Here's an example:
Sub PasteValuesByAssignment()
Range("B1").Value = Range("A1").Value
End Sub
This method is not only faster but also preserves the original formatting.
Method 3: Using the Range.Value
Property
Another approach is to use the Range.Value
property to assign values. This method is similar to the previous one but offers more flexibility. Here's an example:
Sub PasteValuesUsingRangeValue()
Range("B1").Resize(10, 1).Value = Range("A1").Resize(10, 1).Value
End Sub
This method allows you to specify the range size, making it useful for pasting values into a larger range.
Method 4: Using Arrays
When working with large datasets, using arrays can significantly improve performance. Here's an example:
Sub PasteValuesUsingArrays()
Dim arr As Variant
arr = Range("A1:A10").Value
Range("B1").Resize(UBound(arr, 1), UBound(arr, 2)).Value = arr
End Sub
This method is the fastest and most efficient way to paste values in Excel VBA.
Conclusion
Pasting values in Excel VBA is a common task that can be achieved using different methods. While the PasteSpecial
method is the most common approach, it may not always be the most efficient or reliable way to achieve the desired result. By using the methods outlined in this article, you can improve your coding experience and make your VBA code more efficient.
Pasting Values in Excel VBA Image Gallery
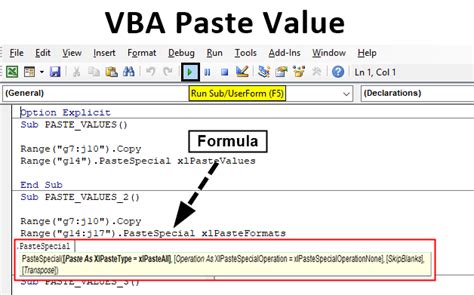
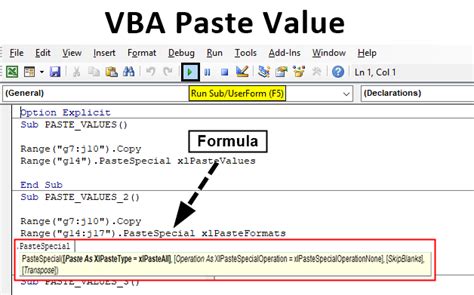
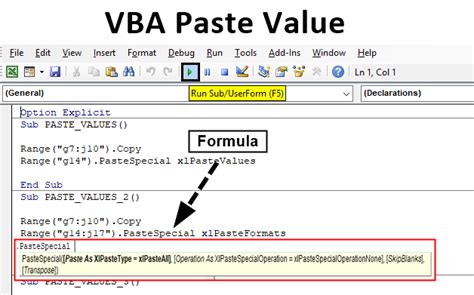
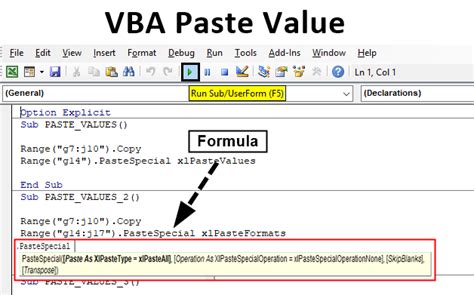
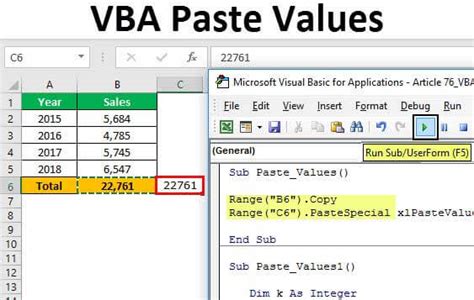
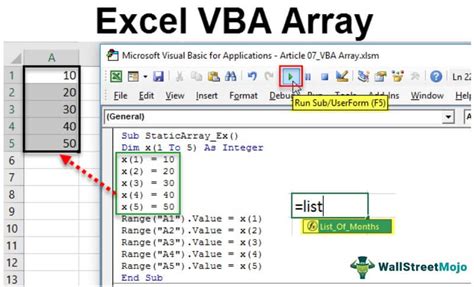
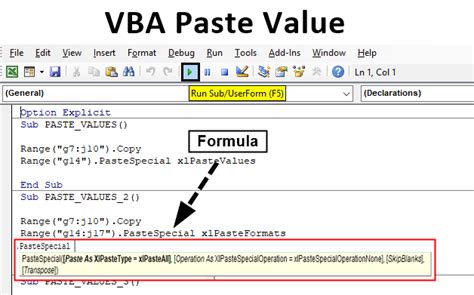
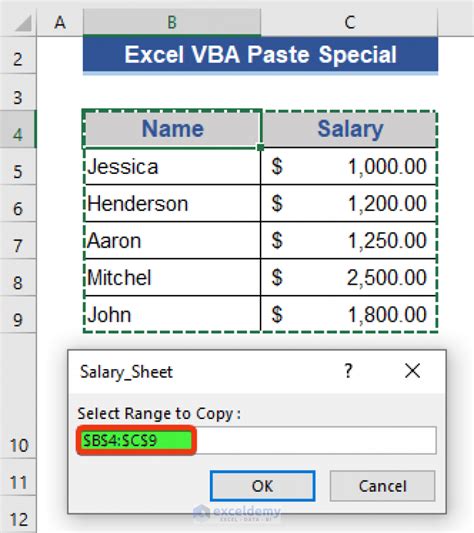
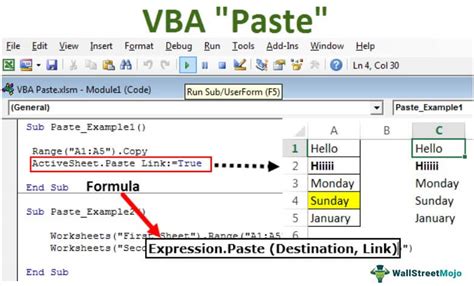
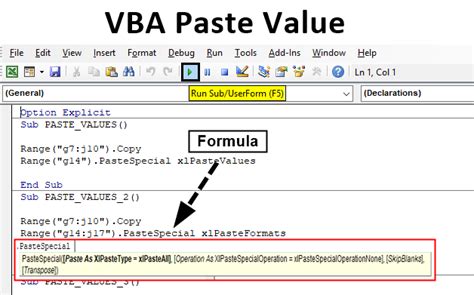
By following the tips and methods outlined in this article, you can improve your Excel VBA skills and make your coding experience more efficient. Remember to experiment with different approaches to find the best solution for your specific needs. Happy coding!