Intro
Master Excel VBA with 5 efficient ways to hide columns. Learn how to auto-hide columns based on conditions, use VBA code to hide multiple columns, and toggle column visibility with a button. Improve your spreadsheet organization and security with these expert VBA techniques, including column indexing and worksheet events.
Excel VBA is a powerful tool that allows users to automate tasks, manipulate data, and create custom interfaces within Excel. One common task that users often need to perform is hiding columns in a worksheet. In this article, we will explore five different ways to hide columns using Excel VBA.
Why Hide Columns?
Before we dive into the different methods, let's briefly discuss why hiding columns might be useful. Hiding columns can help declutter a worksheet, making it easier to focus on the data that matters. It can also help protect sensitive information by hiding columns that contain confidential data. Additionally, hiding columns can make a worksheet more visually appealing by removing unnecessary data.
Method 1: Hide a Single Column
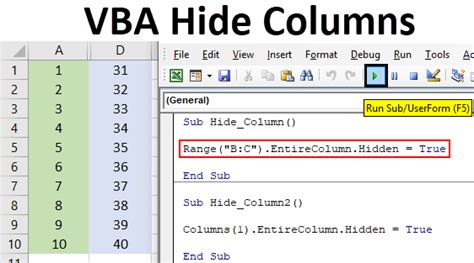
To hide a single column using VBA, you can use the following code:
Sub HideSingleColumn()
Columns("A").Hidden = True
End Sub
In this code, Columns("A")
refers to the column you want to hide, which in this case is column A. The Hidden
property is set to True
to hide the column.
Method 2: Hide Multiple Columns
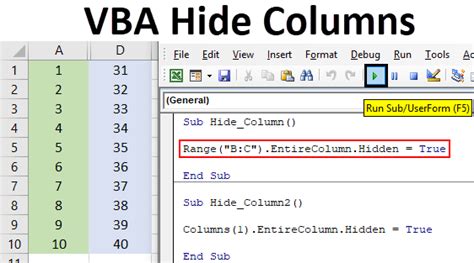
To hide multiple columns, you can use the following code:
Sub HideMultipleColumns()
Columns("A:C").Hidden = True
End Sub
In this code, Columns("A:C")
refers to the range of columns you want to hide, which in this case is columns A to C.
Method 3: Hide Columns Based on a Condition
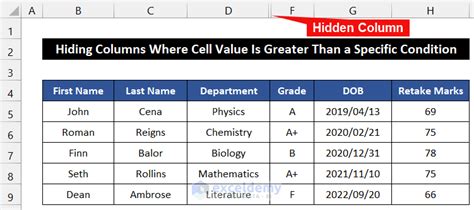
To hide columns based on a condition, you can use the following code:
Sub HideColumnsCondition()
If Range("A1").Value = "Hide" Then
Columns("A").Hidden = True
Else
Columns("A").Hidden = False
End If
End Sub
In this code, the value in cell A1 is checked. If the value is "Hide", then column A is hidden. Otherwise, column A is visible.
Method 4: Hide Columns Using a Loop
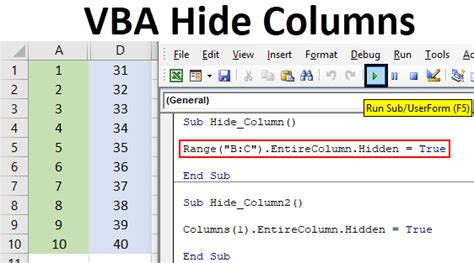
To hide columns using a loop, you can use the following code:
Sub HideColumnsLoop()
For i = 1 To 10
Columns(i).Hidden = True
Next i
End Sub
In this code, a loop is used to iterate through the first 10 columns. Each column is hidden using the Hidden
property.
Method 5: Hide Columns Using a Range
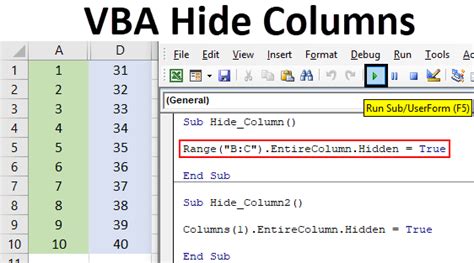
To hide columns using a range, you can use the following code:
Sub HideColumnsRange()
Range("A:E").Columns.Hidden = True
End Sub
In this code, the range A:E is selected, and then the Columns
property is used to hide the columns.
Gallery of Excel VBA Column Hiding Examples
Excel VBA Column Hiding Examples
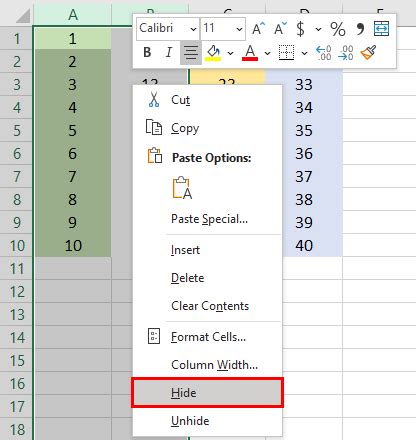
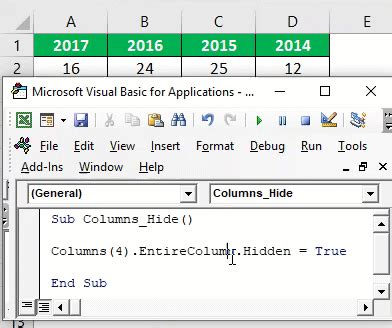
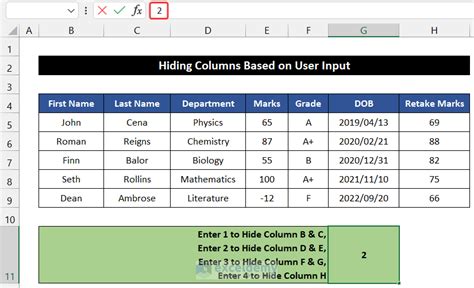
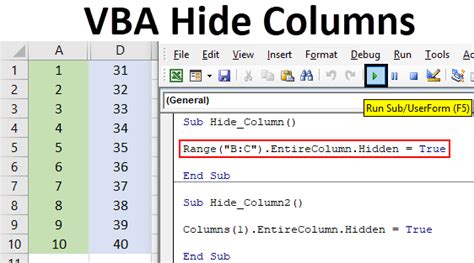
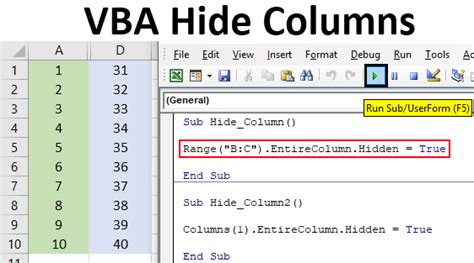
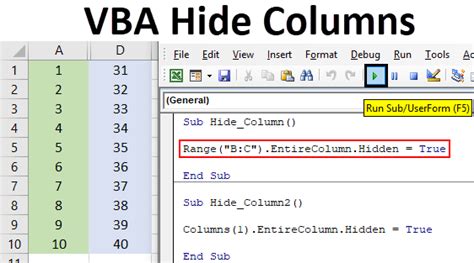
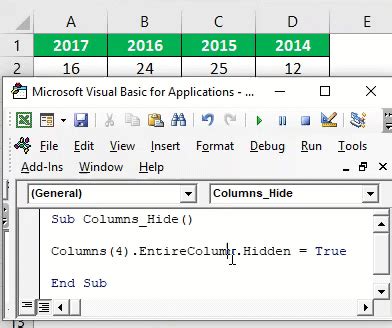
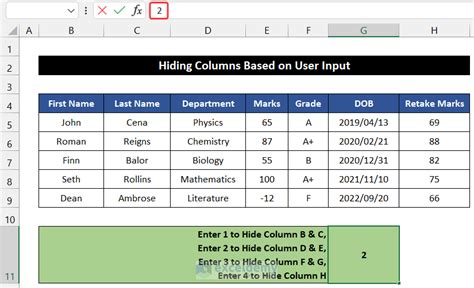
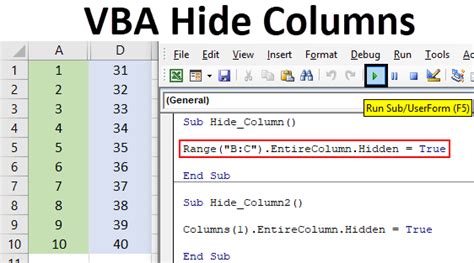
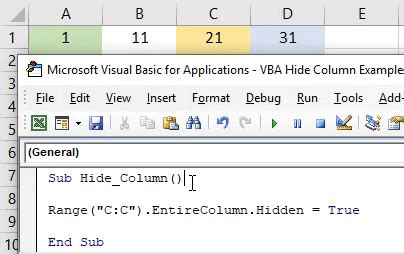
We hope this article has provided you with the knowledge and examples you need to hide columns using Excel VBA. Whether you need to hide a single column, multiple columns, or columns based on a condition, we have shown you five different methods to achieve this. Remember to always test your code and use error handling to ensure that your macros run smoothly.
Share Your Thoughts!
Have you ever needed to hide columns in Excel? What method do you prefer? Share your thoughts and experiences in the comments below!