Intro
Master VBAs Replace command with 5 powerful techniques. Learn how to manipulate text, update data, and refine your code with Find and Replace methods, including Regular Expressions and loops. Discover the best practices for error handling and optimization, and boost your VBA skills with this comprehensive guide.
The Replace command is a versatile tool in VBA (Visual Basic for Applications) that allows you to search for and replace specific text within a string. This command is particularly useful when working with text data, such as parsing text files, manipulating strings, or performing data cleaning tasks. In this article, we will explore five ways to use the Replace command in VBA, highlighting its syntax, usage, and practical examples.
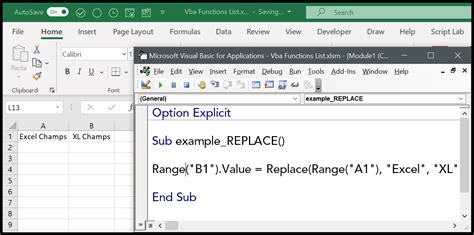
Why Use the Replace Command in VBA?
Before diving into the examples, it's essential to understand the benefits of using the Replace command in VBA. This command allows you to:
- Perform text manipulation tasks efficiently
- Automate data cleaning and processing tasks
- Work with large datasets and text files
- Write more readable and maintainable code
1. Basic Syntax and Usage
The basic syntax of the Replace command in VBA is as follows:
Replace(expression, find, replace, [start, [count, [compare]]])
expression
: The string you want to search and replace text in.find
: The text you want to find and replace.replace
: The text you want to replace the found text with.start
: Optional. The position in the string where you want to start searching.count
: Optional. The number of replacements to make.compare
: Optional. The comparison method to use (e.g., vbBinaryCompare, vbTextCompare).
Here's an example of using the Replace command to replace all occurrences of "old text" with "new text" in a string:
Dim myString As String
myString = "This is old text, and this is old text again."
myString = Replace(myString, "old text", "new text")
Debug.Print myString
2. Replacing Text in a Range of Cells
You can use the Replace command to replace text in a range of cells in a worksheet. This is particularly useful when working with large datasets.
Sub ReplaceTextInRange()
Dim rng As Range
Set rng = Range("A1:A10")
rng.Replace "old text", "new text"
End Sub
In this example, the Replace command is used to replace all occurrences of "old text" with "new text" in the range A1:A10.
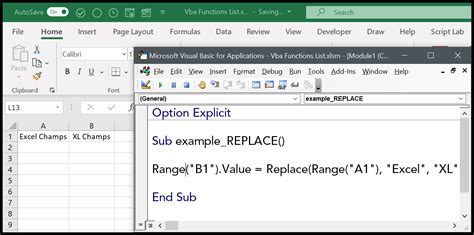
3. Using the Replace Command with Regular Expressions
Regular expressions (regex) are a powerful tool for pattern matching and text manipulation. You can use the Replace command with regex to replace text in a string.
Sub ReplaceTextWithRegex()
Dim myString As String
myString = "Hello, my email address is example@example.com"
myString = Replace(myString, "\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b", "new_email@example.com")
Debug.Print myString
End Sub
In this example, the Replace command is used with a regex pattern to replace the email address in the string with a new email address.
4. Replacing Text in a Text File
You can use the Replace command to replace text in a text file.
Sub ReplaceTextInFile()
Dim filePath As String
filePath = "C:\example.txt"
Dim fileContent As String
fileContent = ReadFile(filePath)
fileContent = Replace(fileContent, "old text", "new text")
WriteFile filePath, fileContent
End Sub
Function ReadFile(filePath As String) As String
Dim fileNumber As Integer
fileNumber = FreeFile
Open filePath For Input As #fileNumber
ReadFile = Input$(LOF(fileNumber), #fileNumber)
Close #fileNumber
End Function
Sub WriteFile(filePath As String, fileContent As String)
Dim fileNumber As Integer
fileNumber = FreeFile
Open filePath For Output As #fileNumber
Write #fileNumber, fileContent
Close #fileNumber
End Sub
In this example, the Replace command is used to replace all occurrences of "old text" with "new text" in a text file.
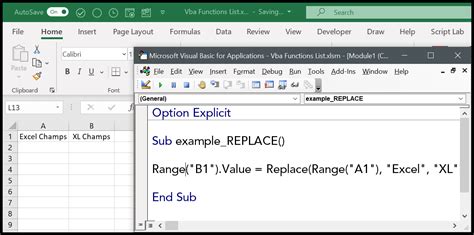
5. Advanced Techniques with the Replace Command
The Replace command can be used in combination with other VBA functions and techniques to achieve advanced text manipulation tasks. For example, you can use the Replace command with the Split function to split a string into an array of substrings and then replace text in each substring.
Sub AdvancedReplace()
Dim myString As String
myString = "apple,banana,orange,grape"
Dim substrings() As String
substrings = Split(myString, ",")
For i = LBound(substrings) To UBound(substrings)
substrings(i) = Replace(substrings(i), "a", "A")
Next i
myString = Join(substrings, ",")
Debug.Print myString
End Sub
In this example, the Replace command is used with the Split function to split a string into an array of substrings and then replace all occurrences of "a" with "A" in each substring.
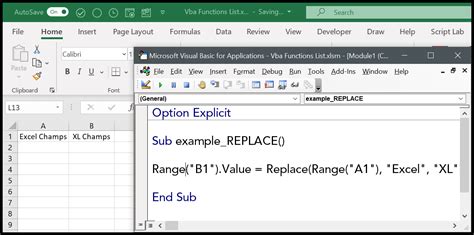
Gallery of VBA Replace Command Examples
VBA Replace Command Examples
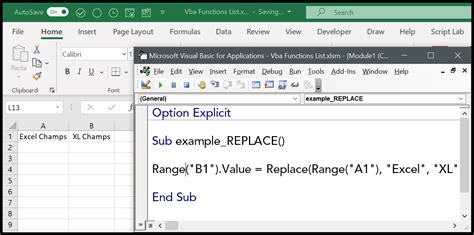
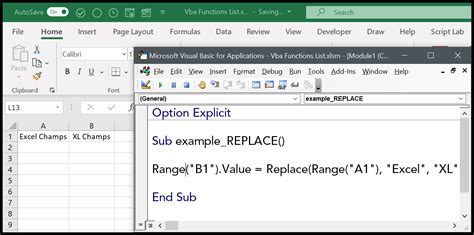
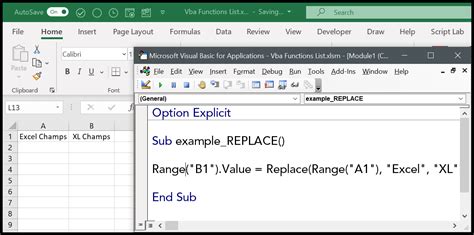
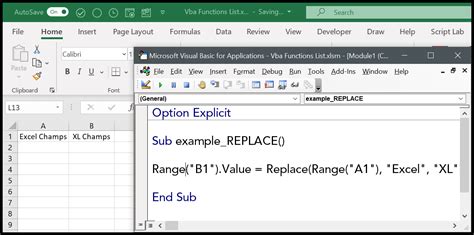
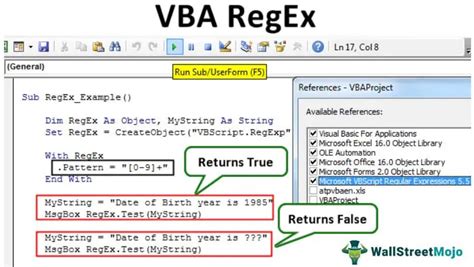
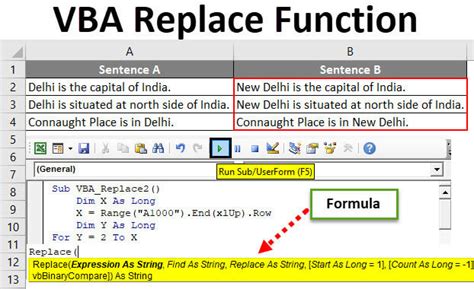
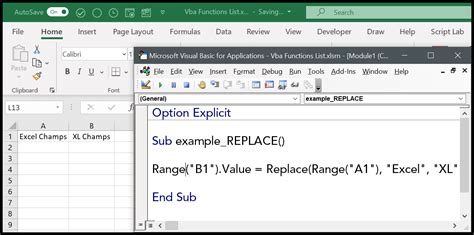
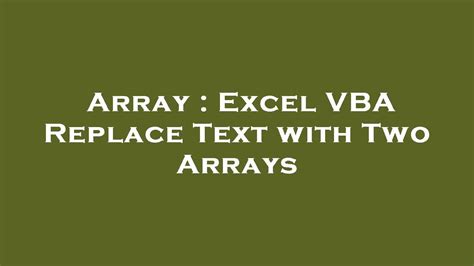
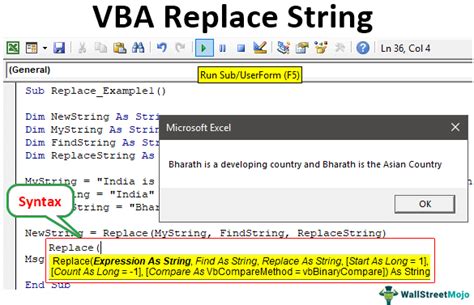
We hope this article has provided you with a comprehensive understanding of the Replace command in VBA and its various uses. Whether you're a beginner or an advanced VBA user, this command is an essential tool to have in your toolkit. If you have any questions or need further assistance, please don't hesitate to ask.