Intro
Master Excel automation with VBA! Discover 3 efficient ways to save as XLS using VBA programming, including file conversion, worksheet manipulation, and Excel object library integration. Learn how to streamline your workflow, optimize file formats, and boost productivity with these expert VBA tips and techniques.
The ability to save files in various formats is a crucial aspect of working with data, especially when it comes to sharing and compatibility. One of the most common file formats used in data analysis and manipulation is XLS, which is associated with Microsoft Excel. When working with VBA (Visual Basic for Applications), saving files as XLS can be particularly useful for creating, editing, and sharing spreadsheet data. In this article, we'll explore three different ways to save a file as XLS using VBA.
The Importance of Saving as XLS
Before diving into the methods, let's quickly discuss why saving as XLS might be important. The XLS format is widely supported by various spreadsheet software, making it a versatile choice for sharing data. Moreover, it can be particularly useful when working with legacy systems or when the recipient doesn't have the latest version of Excel.
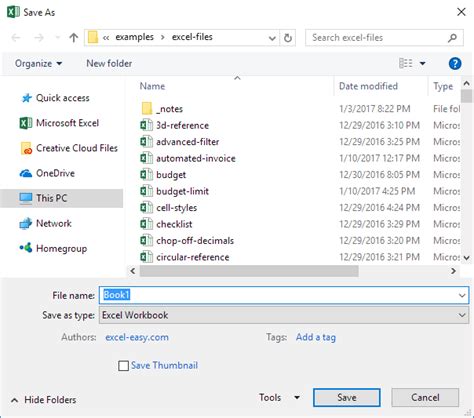
Method 1: Using the SaveAs
Method
One of the most straightforward methods to save a file as XLS using VBA is by utilizing the SaveAs
method. This method allows you to specify the file format, among other parameters, to save your workbook in the desired format.
Sub SaveAsXLS()
ThisWorkbook.SaveAs Filename:="example.xls", FileFormat:=xlExcel8
End Sub
In this example, ThisWorkbook
refers to the active workbook, and SaveAs
is used to save it as "example.xls" with the file format specified as xlExcel8
, which corresponds to the XLS format.
Method 2: Using the Workbook.SaveAs
Method with a Different Approach
Another way to achieve the same result is by using the Workbook.SaveAs
method in a slightly different manner. This approach involves specifying the file format through a numeric value instead of using the xlExcel8
constant.
Sub SaveAsXLSAlternative()
Dim wb As Workbook
Set wb = ThisWorkbook
wb.SaveAs Filename:="example.xls", FileFormat:=56
End Sub
Here, 56
corresponds to the XLS file format, and ThisWorkbook
is used to set the wb
variable as the active workbook.
Method 3: Utilizing Late Binding and the SaveAs
Method
The third method involves using late binding to achieve the same result. Late binding means the object type is determined at runtime rather than at compile time. This method is particularly useful when working with different versions of Excel or when the exact object model is not known in advance.
Sub SaveAsXLSLateBinding()
Dim objExcel As Object
Dim wb As Object
Set objExcel = CreateObject("Excel.Application")
Set wb = objExcel.Workbooks.Add
wb.SaveAs Filename:="example.xls", FileFormat:=56
End Sub
In this example, CreateObject
is used to create an Excel application object late-bound, and then a new workbook is added to this application. The SaveAs
method is then used to save this workbook as XLS.
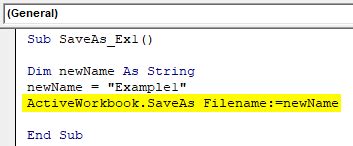
Conclusion and Recommendations
Each of the methods outlined above can be effective in saving a file as XLS using VBA, depending on your specific needs and the context of your project. Here are some recommendations:
- Use Method 1 when you are working within the Excel VBA environment and want a straightforward, early-bound solution.
- Choose Method 2 if you prefer or need to specify file formats using numeric values.
- Opt for Method 3 when working with multiple Excel versions or in scenarios requiring late binding.
Remember, the key to successfully implementing these methods is understanding your project's specific requirements and the limitations of each approach.
VBA Save As XLS Gallery
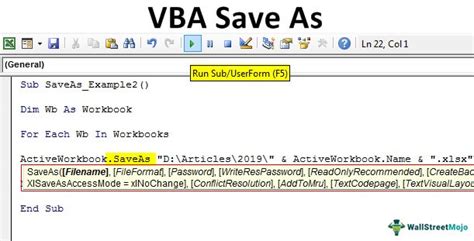
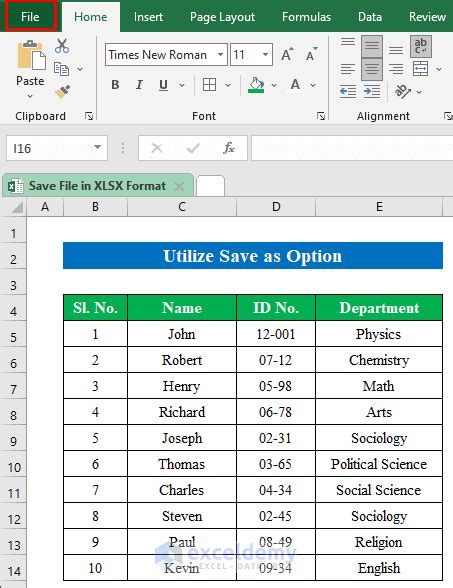
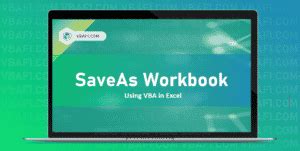
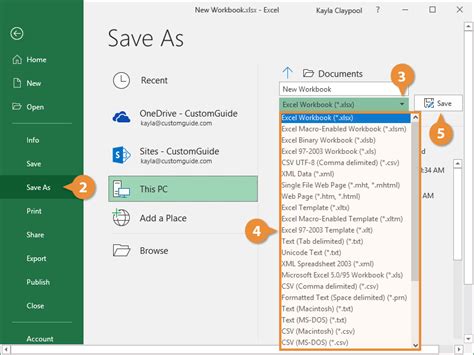
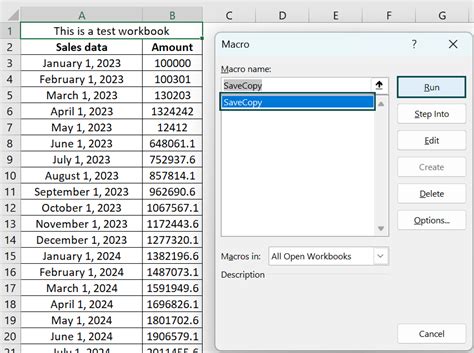
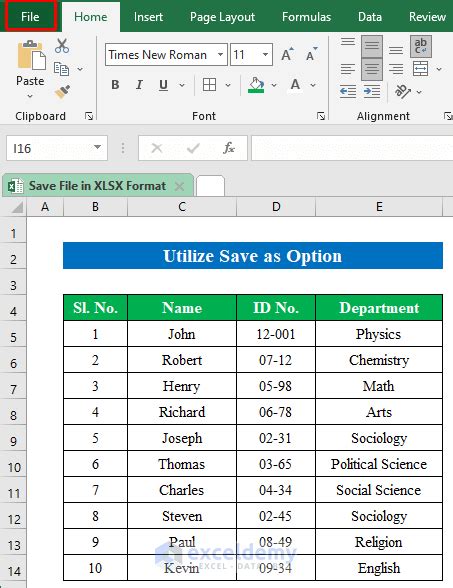
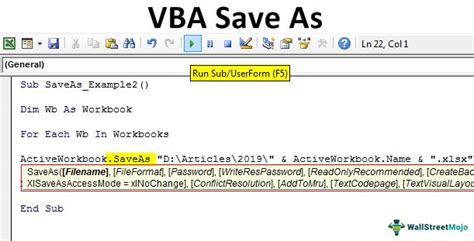
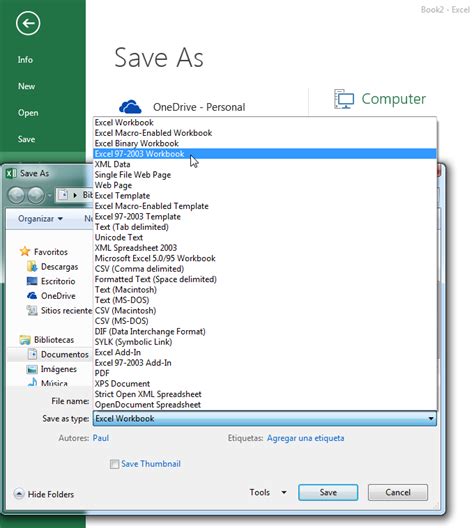
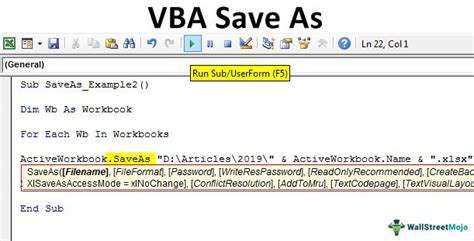
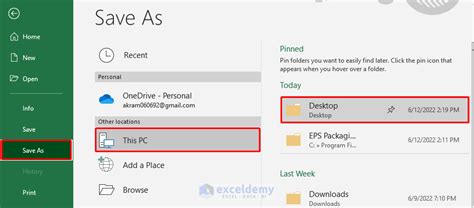
Engage with the Community
We hope this comprehensive guide has been helpful in your journey to learn how to save files as XLS using VBA. If you have any questions, need further clarification, or would like to share your experiences, please don't hesitate to comment below. Your input is invaluable in creating a supportive community for learning and growth. Share this article with others who might find it useful and let's keep the conversation going!