Intro
Optimize your VBA workflow by learning how to efficiently cycle through worksheets. Discover expert techniques and best practices for looping through worksheets, including using Worksheet Index, Worksheet Names, and Workbook loop methods. Improve code performance, reduce errors, and streamline your Excel VBA projects with these actionable tips and examples.
For many Excel users, working with multiple worksheets within a single workbook is a common task. However, manually switching between these worksheets can be time-consuming and inefficient. This is where VBA (Visual Basic for Applications) comes into play, allowing you to automate tasks and significantly improve your productivity. In this article, we'll delve into how to cycle through worksheets efficiently using VBA, exploring the benefits, working mechanisms, and practical examples.
Why Cycle Through Worksheets Efficiently?
When working on a project that involves multiple worksheets, you might find yourself frequently switching between them to perform various tasks, such as data entry, calculations, or formatting. This repetitive process can be not only time-consuming but also prone to errors. By using VBA to cycle through worksheets, you can automate these tasks, reducing the time spent on repetitive actions and minimizing the risk of errors.
Benefits of Using VBA to Cycle Through Worksheets
- Time-saving: Automating the process of cycling through worksheets can save you a significant amount of time, allowing you to focus on more critical aspects of your project.
- Error reduction: By minimizing manual intervention, you reduce the likelihood of errors, ensuring that your tasks are performed accurately and consistently.
- Increased productivity: With the ability to automate tasks, you can work more efficiently, completing projects faster and achieving your goals sooner.
How to Cycle Through Worksheets Using VBA
Cycling through worksheets using VBA involves looping through each worksheet in a workbook and performing a specific action. Here's a basic example to get you started:
Sub CycleThroughWorksheets()
Dim ws As Worksheet
' Loop through each worksheet in the active workbook
For Each ws In ThisWorkbook.Worksheets
' Perform an action on each worksheet
ws.Range("A1").Value = "Hello, World!"
Next ws
End Sub
In this example, we're looping through each worksheet in the active workbook and setting the value of cell A1 to "Hello, World!".
Tips and Variations
- Specify a workbook: Instead of using
ThisWorkbook
, you can specify a particular workbook by its name, likeWorkbooks("MyWorkbook.xlsx")
. - Exclude certain worksheets: You can exclude specific worksheets from the loop by checking their names, like
If ws.Name <> "Sheet1" Then
. - Perform different actions: Depending on your needs, you can perform different actions on each worksheet, such as formatting, data manipulation, or calculations.
- Use a more efficient loop: If you're working with a large number of worksheets, you might want to consider using a
For
loop instead of aFor Each
loop, which can be more efficient.
Practical Examples
- Data consolidation: You can use VBA to cycle through worksheets and consolidate data from each sheet into a single summary sheet.
- Formatting: You can automate the formatting of each worksheet, applying a consistent layout and design throughout the workbook.
- Data validation: You can use VBA to cycle through worksheets and apply data validation rules to ensure consistency and accuracy across the workbook.
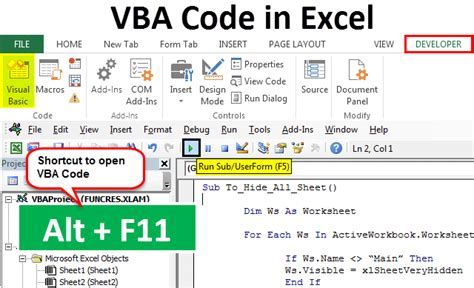
Best Practices
- Keep your code organized: Use clear and descriptive variable names, and consider breaking down large scripts into smaller, more manageable modules.
- Test your code: Before deploying your VBA script, make sure to test it thoroughly to ensure it works as expected.
- Use error handling: Implement error handling mechanisms to catch and handle any errors that may occur during execution.
Conclusion
Cycling through worksheets efficiently using VBA can significantly improve your productivity and reduce errors. By following the tips and best practices outlined in this article, you can create robust and efficient VBA scripts to automate tasks and streamline your workflow.
Gallery of VBA Cycle Through Worksheets
VBA Cycle Through Worksheets Image Gallery
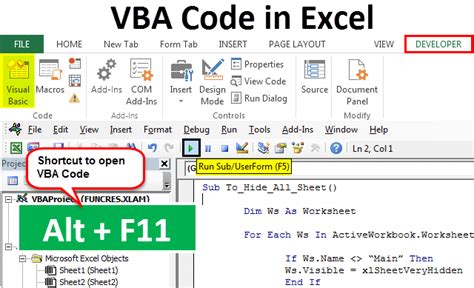
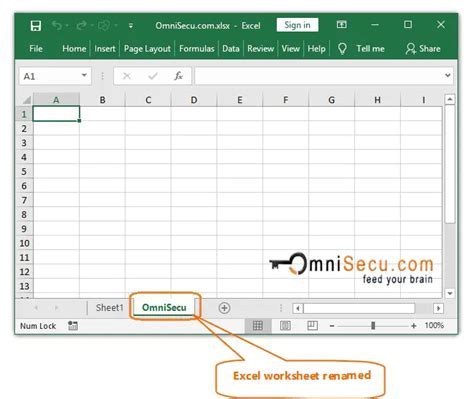
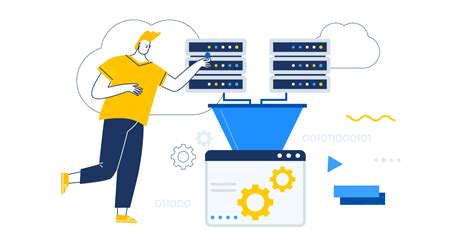
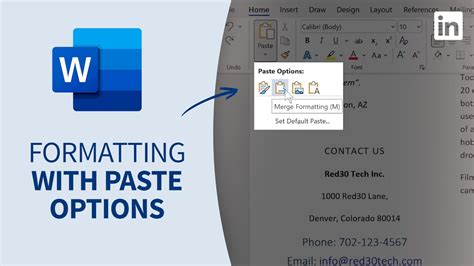
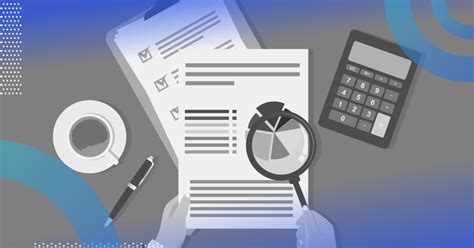
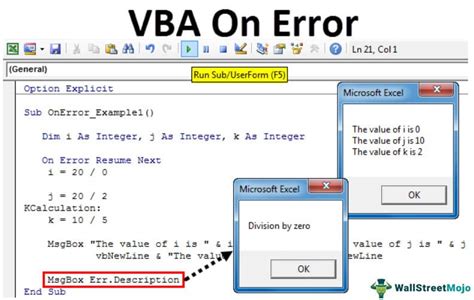
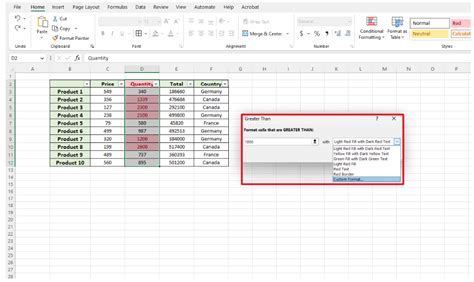
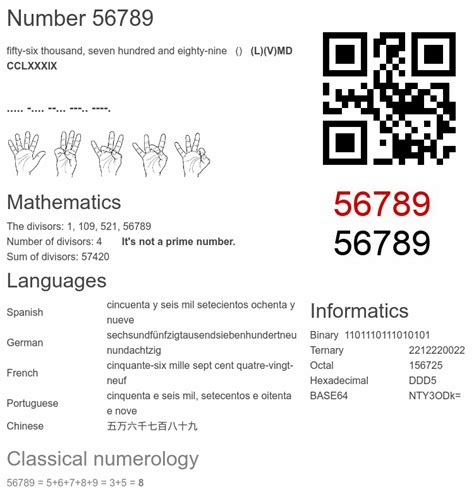
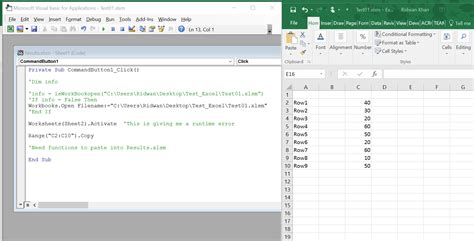
We hope this article has helped you understand how to cycle through worksheets efficiently using VBA. If you have any questions or need further assistance, please don't hesitate to ask.