Intro
Discover the top 5 methods to find the last row in VBA, streamlining your Excel automation. Learn how to use the Cells.Find, Range.Find, UsedRange, SpecialCells, and Do...Loop methods to efficiently identify the last row with data. Master VBAs last row detection techniques and boost your spreadsheet productivity.
Finding the last row in a worksheet is a common task in VBA (Visual Basic for Applications) programming. Whether you're working with Excel, Word, or another application, identifying the last row of data is crucial for tasks like data manipulation, formatting, and analysis. In this article, we will explore five different methods to find the last row in VBA.
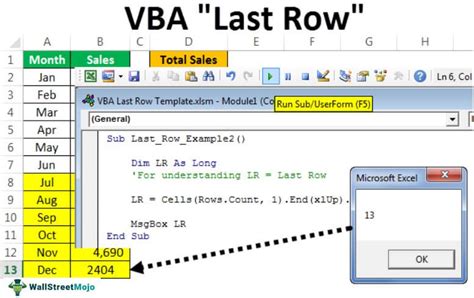
Method 1: Using the End
Property
One of the simplest ways to find the last row is by using the End
property of the Range
object. This property returns a Range
object that represents the cell at the end of the region that contains data.
Sub FindLastRowEndProperty()
Dim lastRow As Long
lastRow = Cells.Find(What:="*", SearchOrder:=xlRows, SearchDirection:=xlPrevious, LookIn:=xlValues).Row
MsgBox "Last row: " & lastRow
End Sub
Method 2: Using the UsedRange
Property
The UsedRange
property returns a Range
object that represents the range of cells that have been used in the worksheet. By finding the last row of the UsedRange
, you can determine the last row of data.
Sub FindLastRowUsedRange()
Dim lastRow As Long
lastRow = ActiveSheet.UsedRange.Rows.Count
MsgBox "Last row: " & lastRow
End Sub
Method 3: Using the Range
Object with xlDown
This method involves using the Range
object with the xlDown
parameter to find the last row of data.
Sub FindLastRowRangeXLDown()
Dim lastRow As Long
lastRow = Range("A1").End(xlDown).Row
MsgBox "Last row: " & lastRow
End Sub
Method 4: Using the Range
Object with xlUp
Similar to the previous method, this approach uses the Range
object with the xlUp
parameter to find the last row of data.
Sub FindLastRowRangeXLUp()
Dim lastRow As Long
lastRow = Range("A" & Rows.Count).End(xlUp).Row
MsgBox "Last row: " & lastRow
End Sub
Method 5: Using the Cells
Collection
This method involves using the Cells
collection to find the last row of data.
Sub FindLastRowCellsCollection()
Dim lastRow As Long
lastRow = Cells.Find(What:="*", SearchOrder:=xlByRows, SearchDirection:=xlPrevious).Row
MsgBox "Last row: " & lastRow
End Sub
Choosing the Right Method
Each method has its strengths and weaknesses. When choosing the right method for your specific needs, consider the following factors:
- Speed: Methods 1 and 5 are generally the fastest, as they use the
Find
method, which is optimized for performance. - Accuracy: Methods 2 and 3 may not always return the correct last row, especially if there are blank cells or formatting issues in the worksheet.
- Flexibility: Method 4 is the most flexible, as it allows you to specify the starting cell and direction of the search.
By understanding the pros and cons of each method, you can choose the best approach for your specific VBA project.
Best Practices
When working with VBA, it's essential to follow best practices to ensure your code is efficient, readable, and maintainable. Here are some tips:
- Use meaningful variable names: Avoid using generic variable names like
x
ory
. Instead, use descriptive names that indicate the purpose of the variable. - Use comments: Comments help explain the purpose of your code and make it easier for others to understand.
- Use error handling: Error handling is crucial in VBA, as it helps you anticipate and manage errors that may occur during execution.
By following these best practices and choosing the right method for finding the last row, you can write more efficient and effective VBA code.
Conclusion
Finding the last row in VBA is a common task that can be accomplished using various methods. By understanding the strengths and weaknesses of each approach, you can choose the best method for your specific needs. Remember to follow best practices, such as using meaningful variable names, comments, and error handling, to ensure your code is efficient, readable, and maintainable.
VBA Last Row Image Gallery
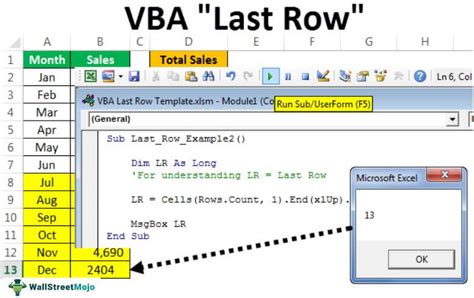
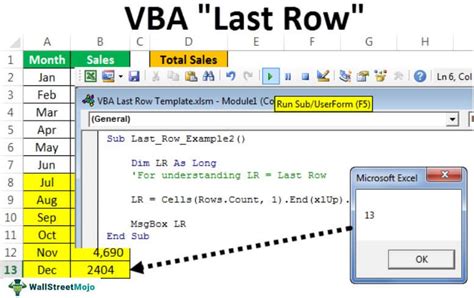
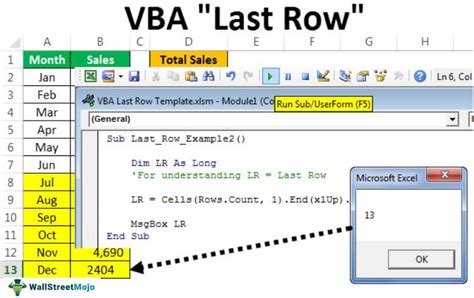
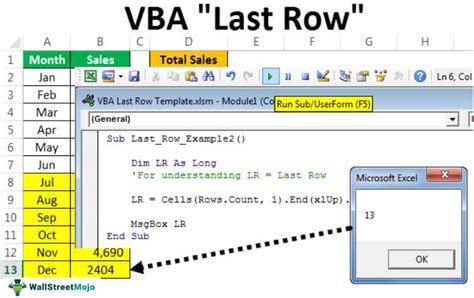
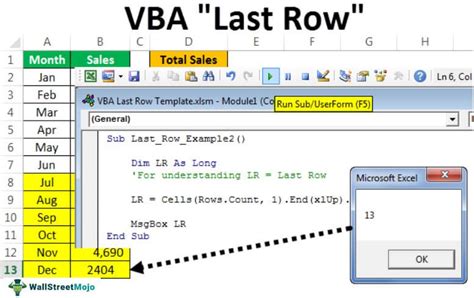
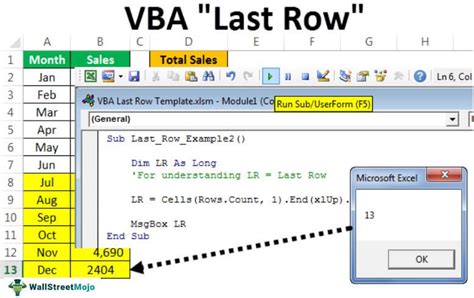
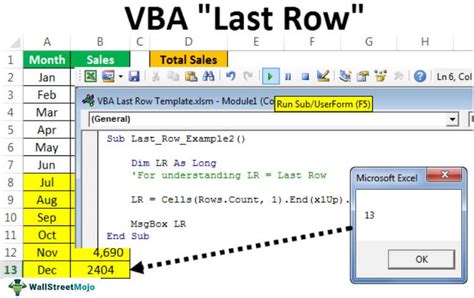
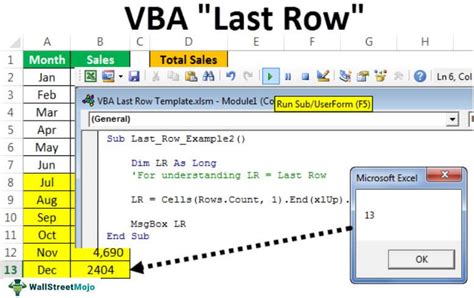
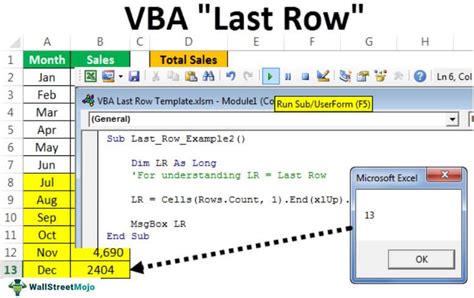
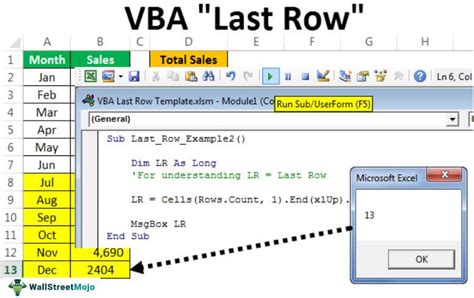
We hope this article has provided you with valuable insights and practical tips for finding the last row in VBA. If you have any questions or need further assistance, please don't hesitate to ask.