Intro
Master data manipulation with VBA by learning how to split a string into an array. This article provides an in-depth guide on using VBAs string splitting functions, including syntax, examples, and best practices for efficient data processing. Boost your Excel skills and unlock the power of array manipulation.
Manipulating data is an essential part of working with Excel, and one of the most common tasks is splitting a string into an array. VBA (Visual Basic for Applications) provides a powerful toolset for achieving this, making it easier to work with complex data sets. In this article, we will explore the world of VBA string manipulation, focusing on the Split function and its applications.
Why Split Strings in VBA?
Before diving into the technical aspects, let's understand why splitting strings is crucial in VBA. When working with text data, it's common to encounter strings that contain multiple values separated by delimiters such as commas, spaces, or tabs. By splitting these strings into arrays, you can easily access and manipulate individual elements, making it easier to perform data analysis, validation, and formatting.
The VBA Split Function
The Split function is a built-in VBA method that splits a string into an array of substrings based on a specified delimiter. The syntax is as follows:
Split(expression, [delimiter], [limit], [compare])
expression
: The input string to be split.delimiter
: The character or string that separates the substrings. If omitted, the default delimiter is a space.limit
: The maximum number of substrings to return. If omitted, all substrings are returned.compare
: The comparison method to use when evaluating the delimiter. Possible values arevbBinaryCompare
(default) orvbTextCompare
.
Basic Examples of Split Function
Let's start with a simple example:
Sub SplitString()
Dim str As String
str = "apple,banana,orange"
Dim arr As Variant
arr = Split(str, ",")
Debug.Print arr(0) ' prints "apple"
Debug.Print arr(1) ' prints "banana"
Debug.Print arr(2) ' prints "orange"
End Sub
In this example, we split the string "apple,banana,orange" into an array using the comma as the delimiter.
Using the Split Function with Multiple Delimiters
Sometimes, you may encounter strings with multiple delimiters. In such cases, you can use the Split function with an array of delimiters. Here's an example:
Sub SplitStringWithMultipleDelimiters()
Dim str As String
str = "apple,banana;orange-grape"
Dim arr As Variant
arr = Split(str, Array(",", ";", "-"))
Debug.Print arr(0) ' prints "apple"
Debug.Print arr(1) ' prints "banana"
Debug.Print arr(2) ' prints "orange"
Debug.Print arr(3) ' prints "grape"
End Sub
In this example, we split the string using an array of delimiters: comma, semicolon, and hyphen.
Handling Missing Values
When working with real-world data, you may encounter strings with missing values or inconsistent formatting. To handle such cases, you can use the IsMissing
function to check if an element in the array is missing.
Sub HandleMissingValues()
Dim str As String
str = "apple,,orange"
Dim arr As Variant
arr = Split(str, ",")
For i = LBound(arr) To UBound(arr)
If IsMissing(arr(i)) Then
Debug.Print "Missing value at position " & i
Else
Debug.Print arr(i)
End If
Next i
End Sub
In this example, we split the string and iterate through the array to check for missing values using the IsMissing
function.
Real-World Applications
Now that we've explored the basics of the Split function, let's look at some real-world applications:
- Data validation: Splitting strings can help you validate data by checking for inconsistencies in formatting or values.
- Data transformation: By splitting strings, you can transform data into a more usable format, such as converting a comma-separated list into a table.
- Data analysis: Splitting strings can facilitate data analysis by allowing you to access and manipulate individual elements.
Gallery of VBA String Manipulation
VBA String Manipulation Image Gallery
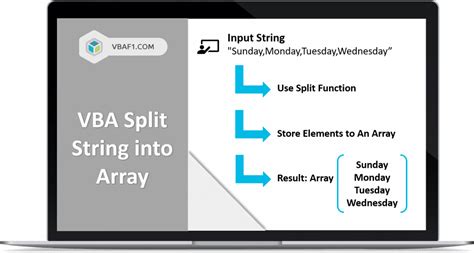
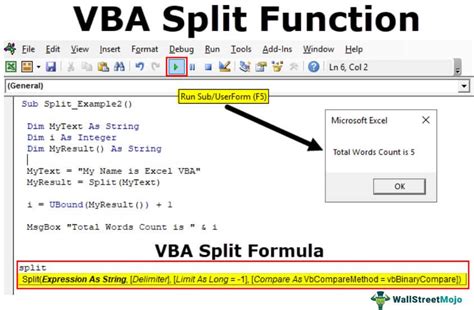
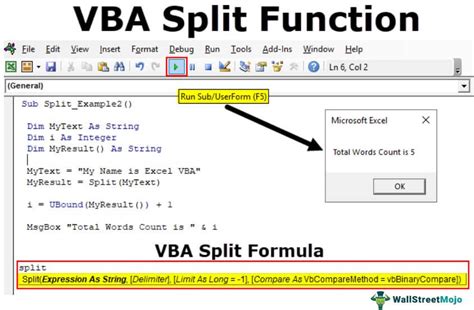
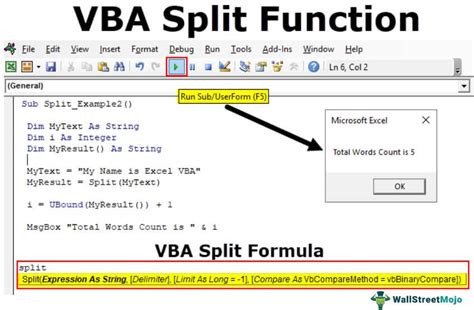
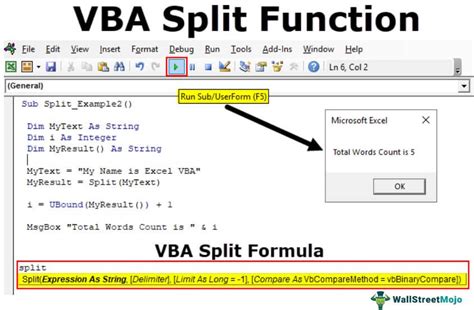
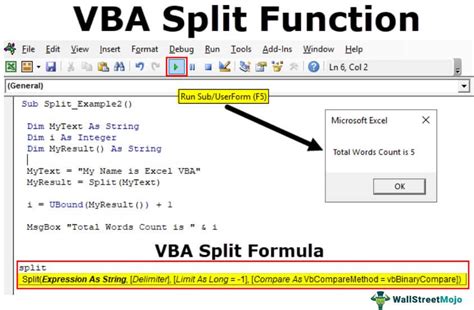
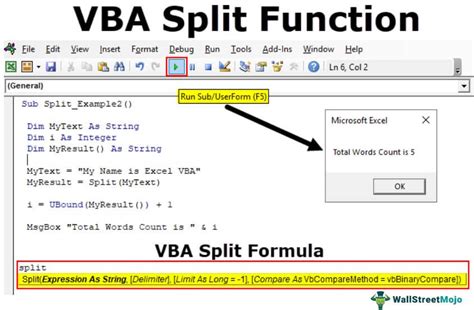
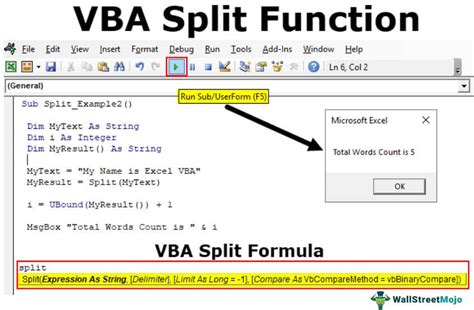
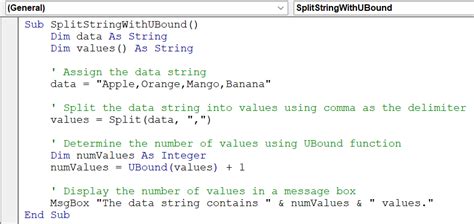
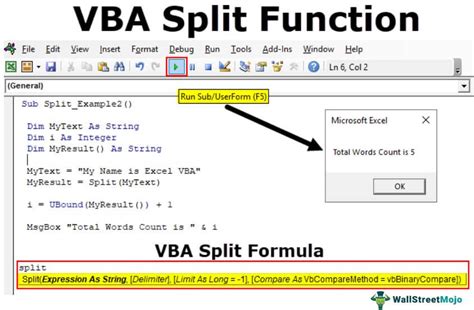
Conclusion
In this article, we explored the world of VBA string manipulation, focusing on the Split function and its applications. By mastering the Split function, you can unlock a wide range of data manipulation possibilities, from simple string splitting to complex data analysis and validation. Whether you're a beginner or an experienced VBA developer, this article has provided you with the knowledge and inspiration to take your string manipulation skills to the next level.