Intro
Discover how to save Excel files as XLSX using VBA with our step-by-step guide. Learn three efficient methods to export Excel files in XLSX format, including using the SaveAs method, Workbook.SaveAs method, and FileDialog object. Master VBA programming and boost productivity with these expert tips and tricks.
Excel is a powerful tool used by millions of people around the world for data analysis, visualization, and management. One of the most common tasks in Excel is saving files in different formats, such as the widely-used XLSX format. While saving an Excel file as XLSX can be done manually through the "Save As" option, using VBA (Visual Basic for Applications) can automate this process, making it more efficient and less prone to errors. In this article, we will explore three ways to save an Excel file as XLSX using VBA.
Why Use VBA to Save Excel Files as XLSX?
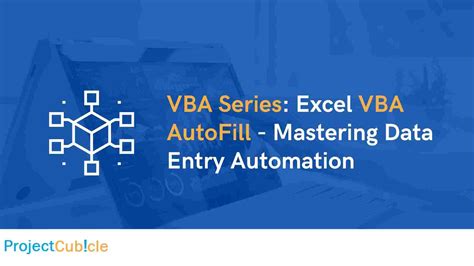
Before we dive into the methods, it's essential to understand the benefits of using VBA to save Excel files as XLSX. VBA is a powerful programming language that allows you to automate repetitive tasks, including file management. By using VBA to save Excel files as XLSX, you can:
- Automate the file-saving process, reducing manual errors and increasing efficiency.
- Save files in bulk, making it ideal for large datasets.
- Customize the file-saving process to meet specific requirements, such as saving files in a specific location or with a specific filename.
Method 1: Using the SaveAs Method
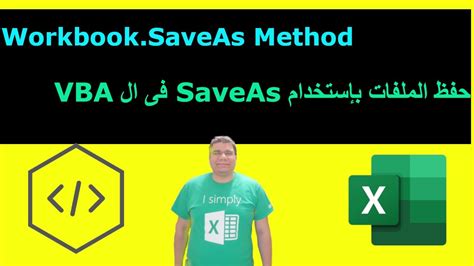
The SaveAs method is the most common way to save an Excel file as XLSX using VBA. This method allows you to specify the file format, filename, and location. Here's an example code snippet that demonstrates how to use the SaveAs method:
Sub SaveAsXLSX()
ThisWorkbook.SaveAs "C:\Example\example.xlsx", xlOpenXMLWorkbook
End Sub
In this code, ThisWorkbook
refers to the active workbook, and SaveAs
is used to save the file as an XLSX file. The file path and name are specified as "C:\Example\example.xlsx", and the file format is set to xlOpenXMLWorkbook
, which corresponds to the XLSX format.
Method 2: Using the SaveAs2 Method
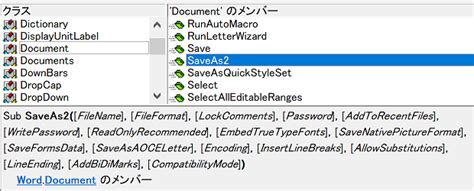
The SaveAs2 method is an updated version of the SaveAs method, introduced in Excel 2007. This method provides more flexibility and allows you to specify additional parameters, such as the file format, filename, and location. Here's an example code snippet that demonstrates how to use the SaveAs2 method:
Sub SaveAsXLSX2()
ThisWorkbook.SaveAs2 "C:\Example\example.xlsx", xlOpenXMLWorkbook,,,,,,,,,,, msoFalse
End Sub
In this code, the SaveAs2
method is used to save the file as an XLSX file. The file path and name are specified as "C:\Example\example.xlsx", and the file format is set to xlOpenXMLWorkbook
. The additional parameters are set to their default values.
Method 3: Using the ExportAsFixedFormat Method
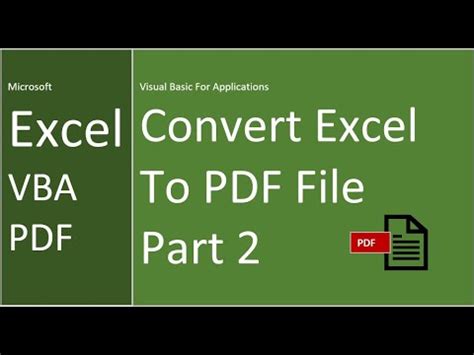
The ExportAsFixedFormat method is a less common way to save an Excel file as XLSX using VBA. This method allows you to export the file in a fixed format, such as XLSX, without modifying the original file. Here's an example code snippet that demonstrates how to use the ExportAsFixedFormat method:
Sub ExportAsXLSX()
ThisWorkbook.ExportAsFixedFormat xlTypeXLSX, "C:\Example\example.xlsx"
End Sub
In this code, the ExportAsFixedFormat
method is used to export the file as an XLSX file. The file path and name are specified as "C:\Example\example.xlsx", and the file format is set to xlTypeXLSX
.
Conclusion and Best Practices
Saving an Excel file as XLSX using VBA can be done in various ways, depending on your specific requirements. When choosing a method, consider the following best practices:
- Use the SaveAs method for simple file-saving tasks.
- Use the SaveAs2 method for more complex file-saving tasks that require additional parameters.
- Use the ExportAsFixedFormat method for exporting files in a fixed format without modifying the original file.
Remember to always test your VBA code in a controlled environment before deploying it in production.
FAQs
- What is the difference between the SaveAs and SaveAs2 methods?
- The SaveAs method is an older method that provides basic file-saving functionality. The SaveAs2 method is an updated version that provides more flexibility and additional parameters.
- Can I use the ExportAsFixedFormat method to save files in other formats?
- Yes, the ExportAsFixedFormat method can be used to export files in various formats, including PDF, XPS, and CSV.
Excel VBA Automation Gallery
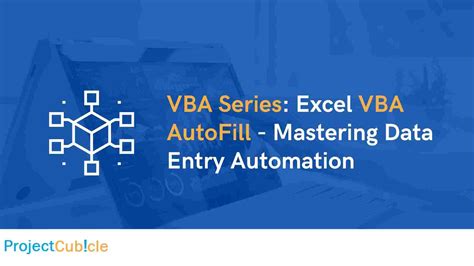
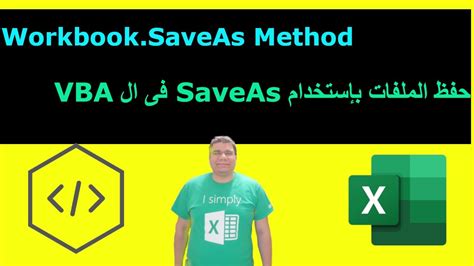
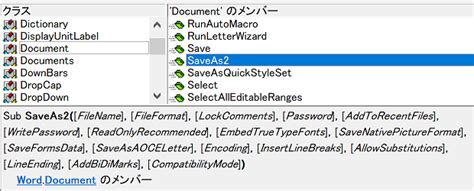
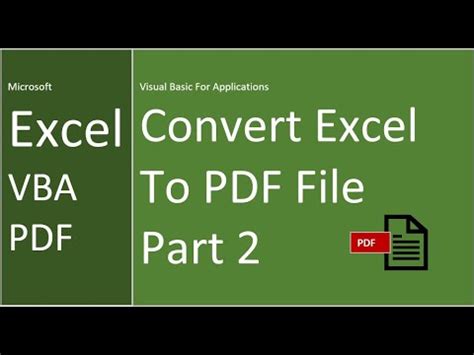
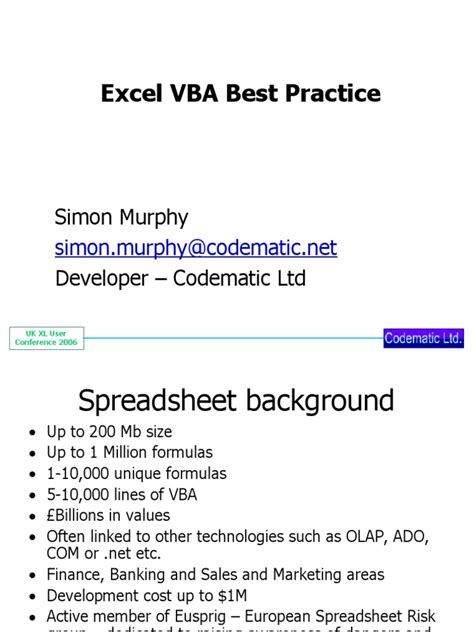
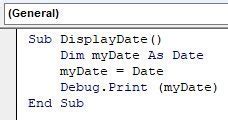
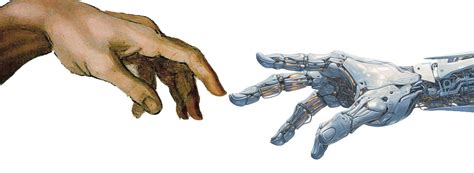
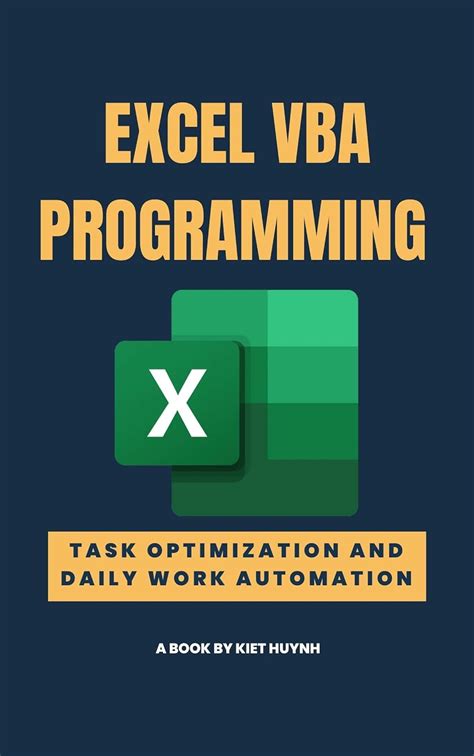
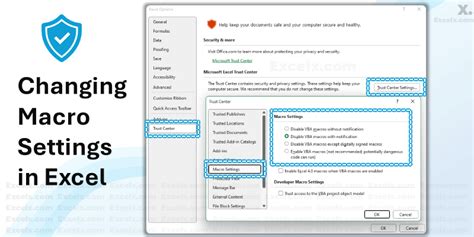
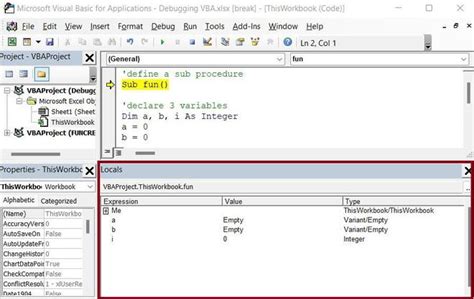
We hope this article has provided you with a comprehensive understanding of how to save an Excel file as XLSX using VBA. If you have any further questions or would like to share your experiences, please leave a comment below.