Intro
Master the art of copying current workbooks in Excel VBA with ease. Learn how to automate duplicate workbooks, copy Excel worksheets, and replicate entire workbooks using VBA macros. Discover simple code snippets and expert tips to streamline your workflow and boost productivity. Perfect for Excel power users and VBA beginners alike.
Copying a current workbook in Excel VBA can be a useful skill to have, especially when working on multiple projects or collaborating with others. In this article, we will explore the different ways to copy a current workbook in Excel VBA, along with practical examples and code snippets.
Why Copy a Workbook in Excel VBA?
There are several reasons why you might want to copy a workbook in Excel VBA. Some common scenarios include:
- Creating a backup of your workbook before making significant changes
- Sharing a workbook with others while keeping the original intact
- Creating multiple versions of a workbook for different projects or clients
- Archiving a workbook for future reference
Using the Workbook.SaveCopyAs
Method
One of the simplest ways to copy a workbook in Excel VBA is by using the Workbook.SaveCopyAs
method. This method allows you to save a copy of the current workbook to a new file location.
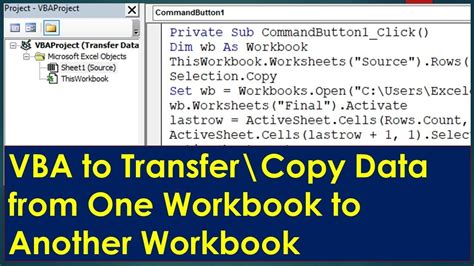
Here is an example code snippet that demonstrates how to use the Workbook.SaveCopyAs
method:
Sub CopyWorkbook()
Dim filePath As String
filePath = "C:\Users\Username\Documents\CopyOfWorkbook.xlsx"
ThisWorkbook.SaveCopyAs filePath
End Sub
Using the Workbook.SaveAs
Method
Another way to copy a workbook in Excel VBA is by using the Workbook.SaveAs
method. This method allows you to save the workbook with a new file name or location.
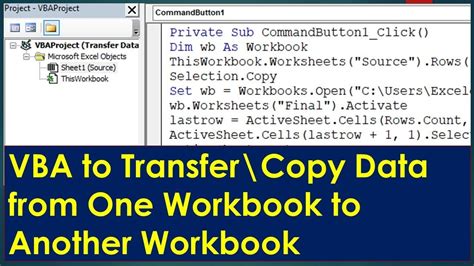
Here is an example code snippet that demonstrates how to use the Workbook.SaveAs
method:
Sub CopyWorkbookAlternative()
Dim filePath As String
filePath = "C:\Users\Username\Documents\CopyOfWorkbookAlternative.xlsx"
ThisWorkbook.SaveAs filePath
End Sub
Using the Workbooks.Add
Method
You can also copy a workbook in Excel VBA by using the Workbooks.Add
method. This method allows you to create a new workbook and then copy the contents of the current workbook to the new one.
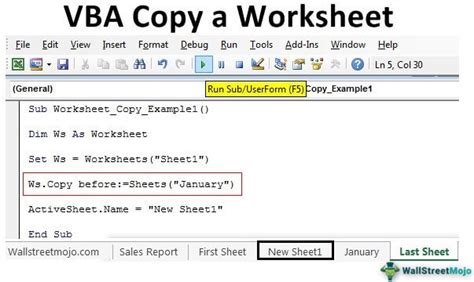
Here is an example code snippet that demonstrates how to use the Workbooks.Add
method:
Sub CopyWorkbookAddNew()
Dim newWorkbook As Workbook
Set newWorkbook = Workbooks.Add
ThisWorkbook.Sheets.Copy Before:=newWorkbook.Sheets(1)
newWorkbook.SaveAs "C:\Users\Username\Documents\CopyOfWorkbookAddNew.xlsx"
End Sub
Using the Workbooks.Open
Method
Finally, you can also copy a workbook in Excel VBA by using the Workbooks.Open
method. This method allows you to open a new instance of the current workbook and then save it with a new file name or location.
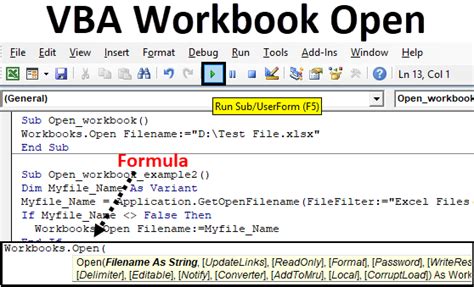
Here is an example code snippet that demonstrates how to use the Workbooks.Open
method:
Sub CopyWorkbookOpenNew()
Dim newWorkbook As Workbook
Set newWorkbook = Workbooks.Open(ThisWorkbook.FullName)
newWorkbook.SaveAs "C:\Users\Username\Documents\CopyOfWorkbookOpenNew.xlsx"
End Sub
Conclusion
In this article, we explored different ways to copy a current workbook in Excel VBA. We covered the Workbook.SaveCopyAs
, Workbook.SaveAs
, Workbooks.Add
, and Workbooks.Open
methods, along with practical examples and code snippets. By using these methods, you can easily copy a workbook in Excel VBA and use it for various purposes, such as creating backups, sharing with others, or archiving for future reference.
Gallery of Copy Workbook Excel VBA
Copy Workbook Excel VBA Image Gallery
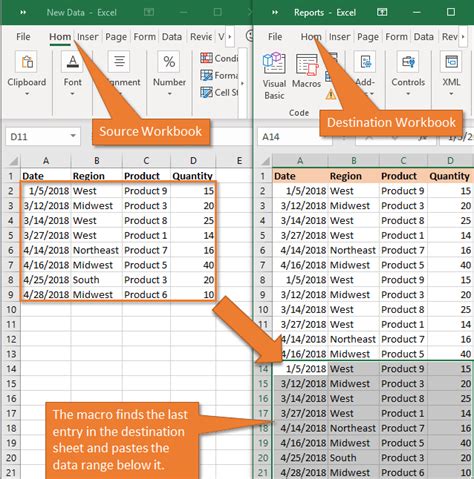
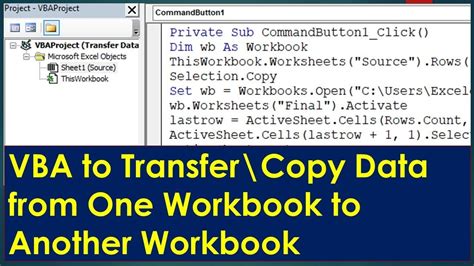
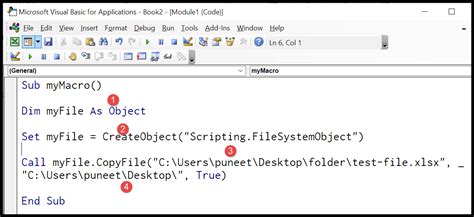
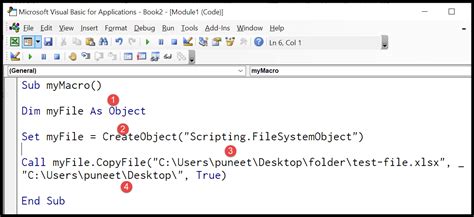
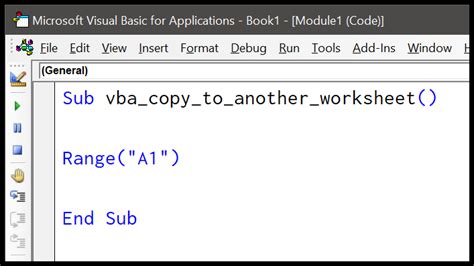
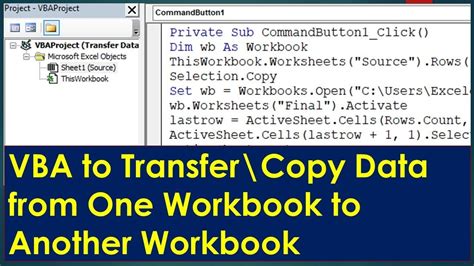
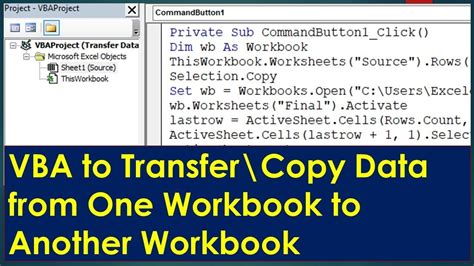
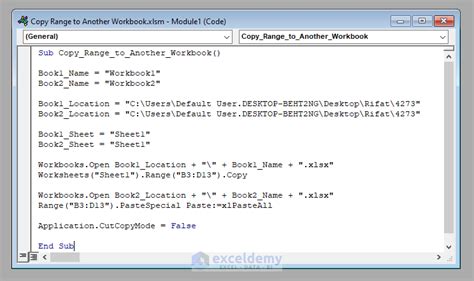
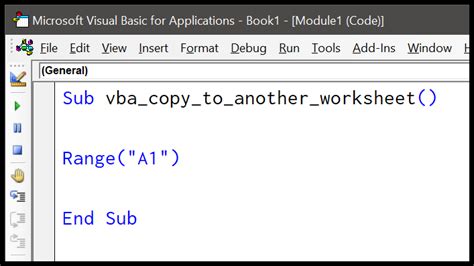
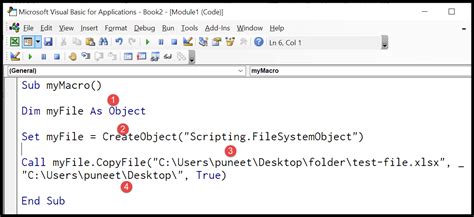