Intro
Master Excel VBA with our expert guide on adding rows dynamically. Learn 5 efficient ways to insert rows in Excel using VBA, including looping, range offset, and worksheet events. Boost productivity and automate tasks with these practical VBA row addition methods.
Adding rows in Excel VBA is a fundamental task that can be accomplished in several ways, depending on the specific requirements of your project. Whether you're dealing with a small dataset or a large, complex spreadsheet, mastering the art of adding rows programmatically can save you a significant amount of time and effort. In this article, we will explore five different methods for adding rows in Excel VBA, including their benefits and potential pitfalls.
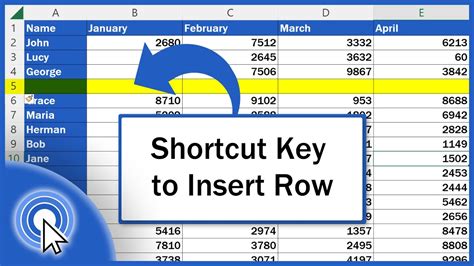
Understanding the Basics
Before diving into the different methods, it's essential to understand the basic syntax and structure of Excel VBA. VBA (Visual Basic for Applications) is a programming language developed by Microsoft that allows you to create and automate tasks in Excel. To access the VBA editor, press Alt + F11
or navigate to the Developer tab in the ribbon.
Method 1: Using the Range.Insert
Method
The Range.Insert
method is one of the most straightforward ways to add rows in Excel VBA. This method inserts a new row above the specified range and shifts the existing cells down.
Sub AddRowUsingInsertMethod()
Dim rng As Range
Set rng = Range("A1")
rng.Insert Shift:=xlDown
End Sub
In this example, we create a range object rng
and set it to cell A1
. We then use the Insert
method to add a new row above the range, shifting the existing cells down.
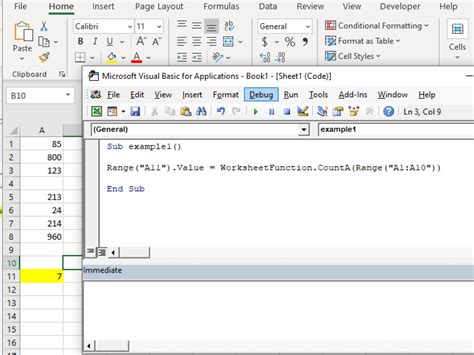
Method 2: Using the Range.EntireRow.Insert
Method
Another way to add rows is by using the Range.EntireRow.Insert
method. This method inserts a new row above the specified range and shifts the existing rows down.
Sub AddRowUsingEntireRowInsertMethod()
Dim rng As Range
Set rng = Range("A1")
rng.EntireRow.Insert
End Sub
In this example, we create a range object rng
and set it to cell A1
. We then use the EntireRow.Insert
method to add a new row above the range, shifting the existing rows down.
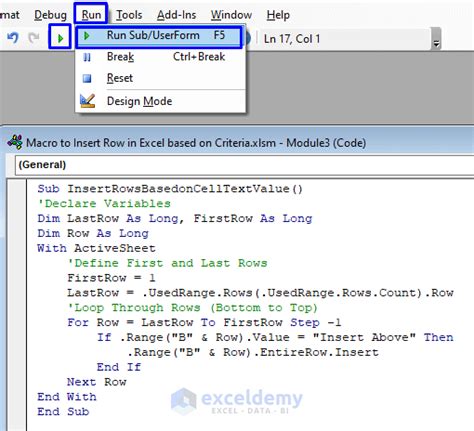
Method 3: Using the Rows.Insert
Method
The Rows.Insert
method is another way to add rows in Excel VBA. This method inserts a new row above the specified row and shifts the existing rows down.
Sub AddRowUsingRowsInsertMethod()
Rows(1).Insert
End Sub
In this example, we use the Rows.Insert
method to add a new row above row 1, shifting the existing rows down.
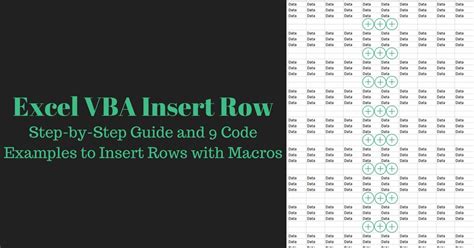
Method 4: Using the Cells.Insert
Method
The Cells.Insert
method is another way to add rows in Excel VBA. This method inserts a new row above the specified cell and shifts the existing cells down.
Sub AddRowUsingCellsInsertMethod()
Cells(1, 1).Insert Shift:=xlDown
End Sub
In this example, we use the Cells.Insert
method to add a new row above cell A1
, shifting the existing cells down.
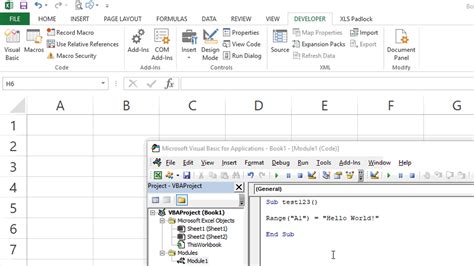
Method 5: Using the Worksheet.Rows.Add
Method
The Worksheet.Rows.Add
method is another way to add rows in Excel VBA. This method inserts a new row above the specified row and shifts the existing rows down.
Sub AddRowUsingWorksheetRowsAddMethod()
ThisWorkbook.Worksheets("Sheet1").Rows.Add
End Sub
In this example, we use the Worksheet.Rows.Add
method to add a new row above the last row in the worksheet, shifting the existing rows down.
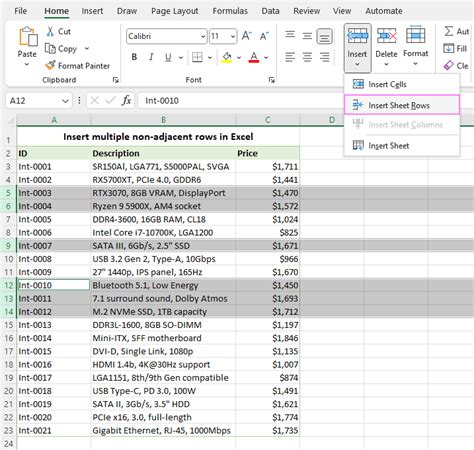
Conclusion
Adding rows in Excel VBA is a straightforward task that can be accomplished using various methods. By understanding the benefits and potential pitfalls of each method, you can choose the best approach for your specific project. Whether you're working with small datasets or complex spreadsheets, mastering the art of adding rows programmatically can save you a significant amount of time and effort.
Gallery of Excel VBA Images
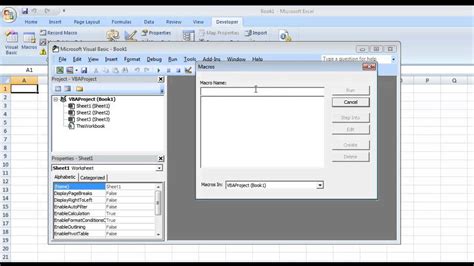
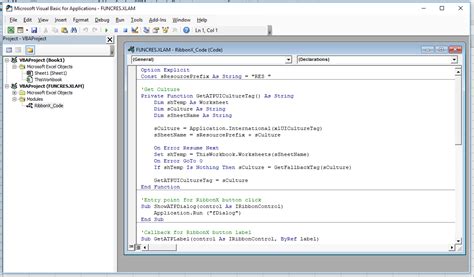
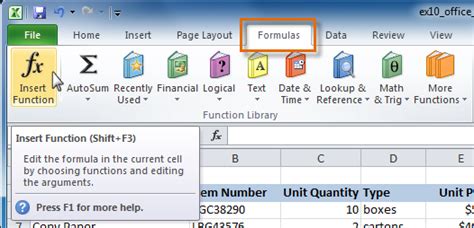
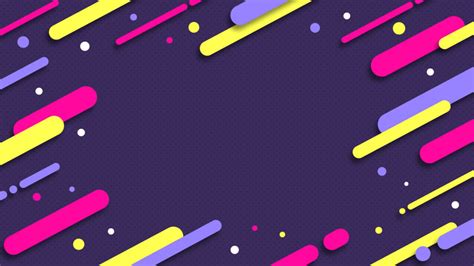
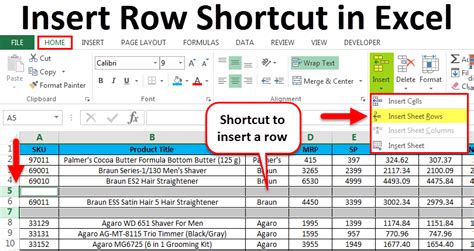
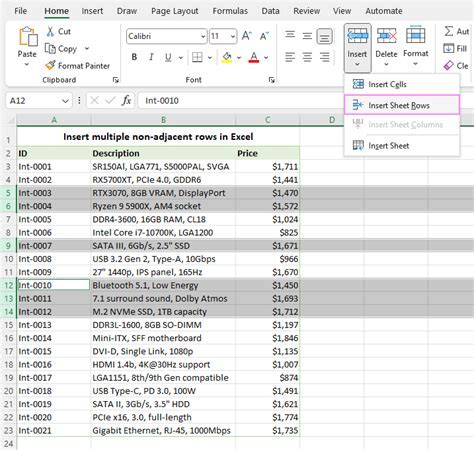
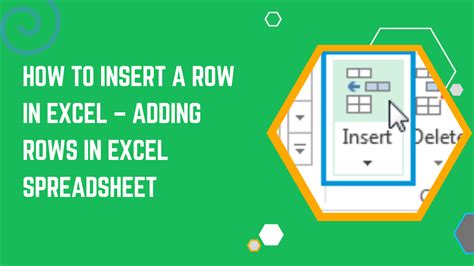
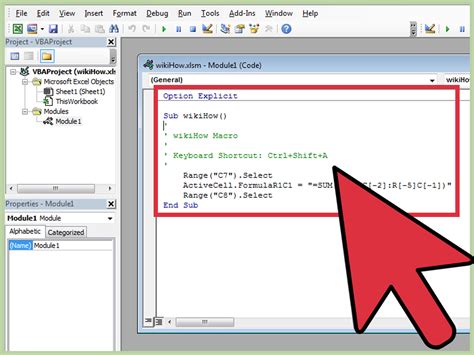
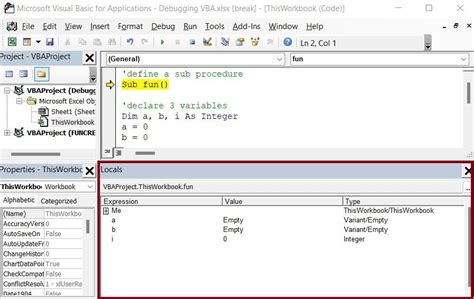
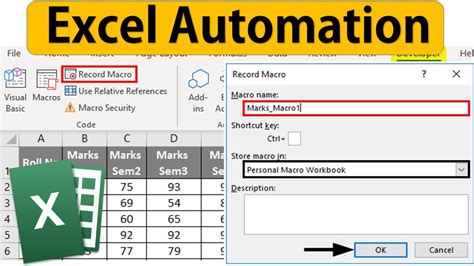
We hope this article has provided you with a comprehensive understanding of how to add rows in Excel VBA. Whether you're a beginner or an experienced programmer, mastering the art of adding rows programmatically can save you a significant amount of time and effort. If you have any questions or need further assistance, please don't hesitate to comment below.