Get File Names From Folder With Excel VBA
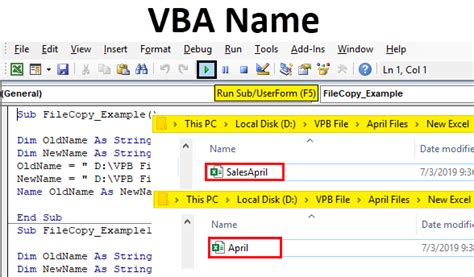
Excel VBA is a powerful tool that allows users to automate tasks and interact with the operating system. One common task is to retrieve a list of file names from a specific folder. This can be useful for various applications, such as data import, file management, or automation of tasks.
In this article, we will explore how to get file names from a folder using Excel VBA. We will cover the different methods available, including using the Dir
function, the FileSystemObject
, and the Shell
object.
Method 1: Using the Dir Function
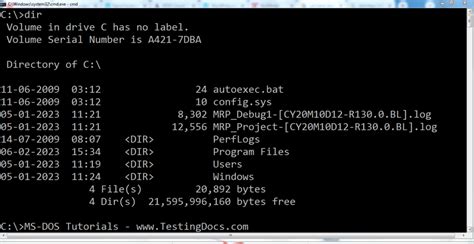
The Dir
function is a built-in VBA function that returns the name of a file or directory. We can use this function to retrieve a list of file names from a specific folder.
Here is an example code snippet:
Sub GetFileNames()
Dim folderPath As String
Dim fileName As String
folderPath = "C:\Example\Folder"
fileName = Dir(folderPath & "\*.*")
While fileName <> ""
Debug.Print fileName
fileName = Dir
Wend
End Sub
This code sets the folderPath
variable to the desired folder and uses the Dir
function to retrieve the first file name. The While
loop continues to retrieve file names until there are no more files in the folder.
Advantages and Disadvantages of Using the Dir Function
Advantages:
- Easy to use and implement
- Fast execution time
Disadvantages:
- Limited functionality (only returns file names, not other file attributes)
- Not suitable for large folders or complex file structures
Method 2: Using the FileSystemObject
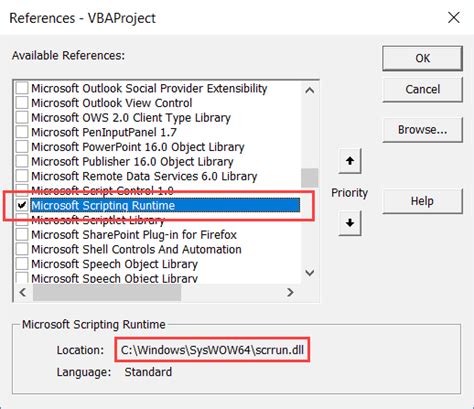
The FileSystemObject
is a more powerful and flexible way to interact with the file system. We can use this object to retrieve a list of file names from a specific folder.
Here is an example code snippet:
Sub GetFileNames()
Dim fso As Object
Dim folder As Object
Dim file As Object
Set fso = CreateObject("Scripting.FileSystemObject")
Set folder = fso.GetFolder("C:\Example\Folder")
For Each file In folder.Files
Debug.Print file.Name
Next file
End Sub
This code creates a FileSystemObject
instance and uses the GetFolder
method to retrieve a reference to the desired folder. The For Each
loop iterates over the files in the folder and prints the file name.
Advantages and Disadvantages of Using the FileSystemObject
Advantages:
- More flexible and powerful than the
Dir
function - Can retrieve other file attributes (e.g., file size, last modified date)
Disadvantages:
- Requires the
Scripting.FileSystemObject
library to be installed and registered - Slower execution time compared to the
Dir
function
Method 3: Using the Shell Object
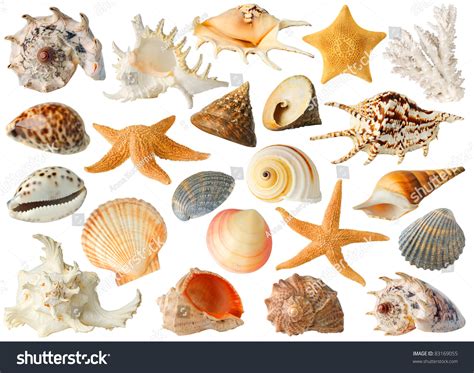
The Shell
object is another way to interact with the file system. We can use this object to retrieve a list of file names from a specific folder.
Here is an example code snippet:
Sub GetFileNames()
Dim shell As Object
Dim folder As Object
Dim file As Object
Set shell = CreateObject("Shell.Application")
Set folder = shell.Namespace("C:\Example\Folder")
For Each file In folder.Items
Debug.Print file.Name
Next file
End Sub
This code creates a Shell
object instance and uses the Namespace
method to retrieve a reference to the desired folder. The For Each
loop iterates over the files in the folder and prints the file name.
Advantages and Disadvantages of Using the Shell Object
Advantages:
- Fast execution time
- Can retrieve other file attributes (e.g., file size, last modified date)
Disadvantages:
- Requires the
Shell.Application
library to be installed and registered - Limited functionality compared to the
FileSystemObject
Get File Names From Folder With Excel VBA Image Gallery
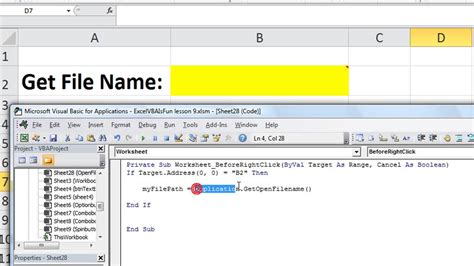
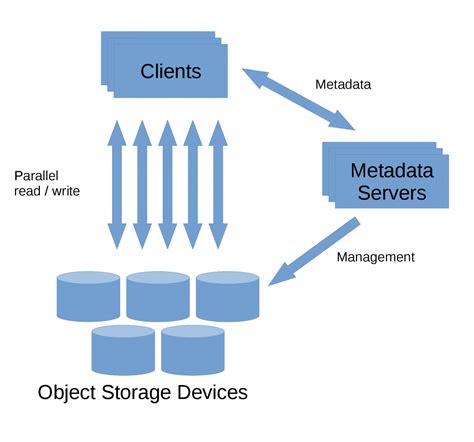
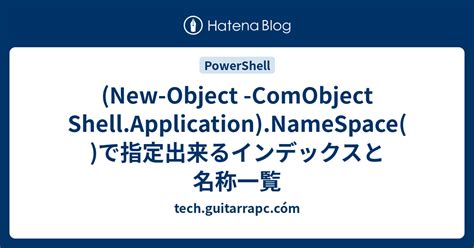
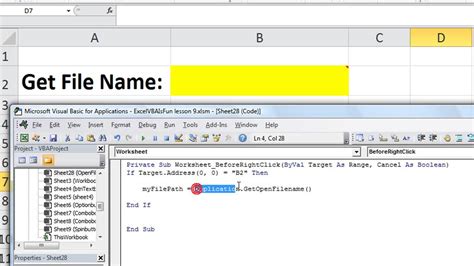
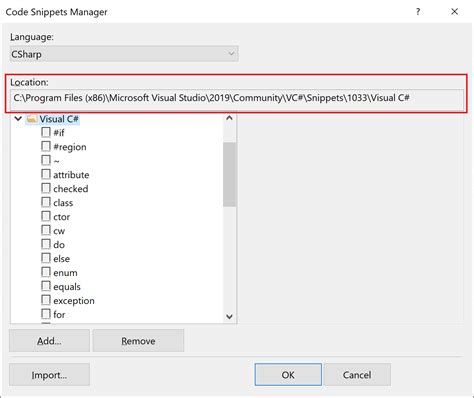
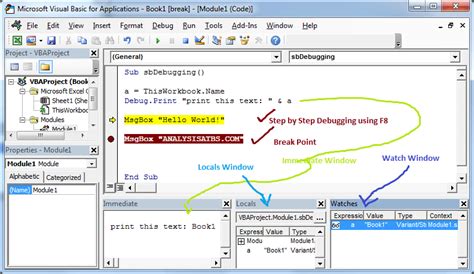
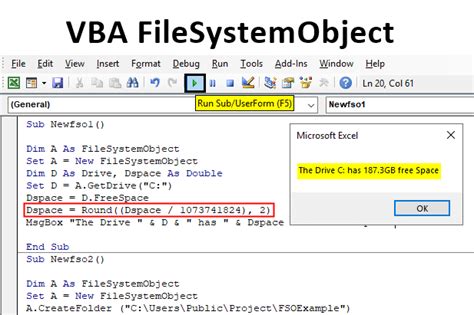
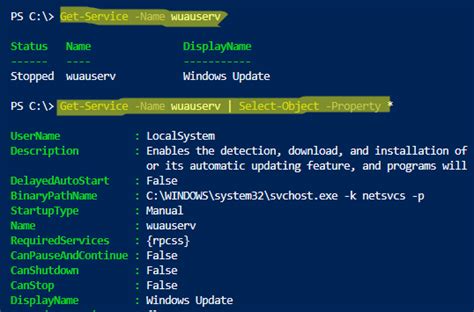
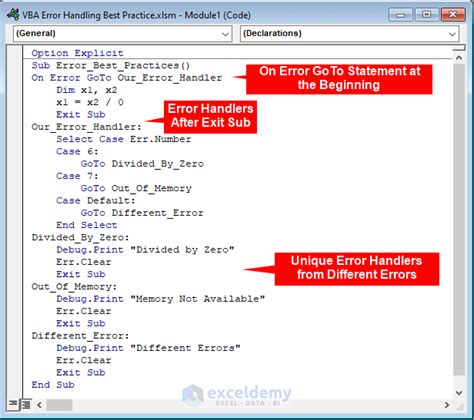
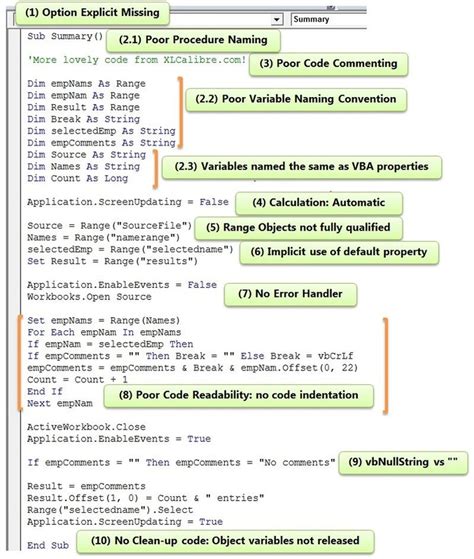
In conclusion, there are three methods to get file names from a folder using Excel VBA: the Dir
function, the FileSystemObject
, and the Shell
object. Each method has its advantages and disadvantages, and the choice of method depends on the specific requirements of the project.
We hope this article has been informative and helpful in your Excel VBA development journey. If you have any questions or need further assistance, please feel free to ask in the comments section below.