Intro
Master VBA copy and paste range with ease. Learn how to efficiently transfer data between worksheets and workbooks using Visual Basic for Applications. Discover techniques for copying and pasting ranges, handling errors, and optimizing performance. Improve your Excel automation skills and streamline your workflow with this comprehensive guide.
VBA Copy and Paste Range Made Easy
Mastering VBA copy and paste range techniques is essential for any Excel user who wants to automate tasks and increase productivity. In this article, we will delve into the world of VBA copy and paste range, exploring the different methods, benefits, and best practices. Whether you're a beginner or an advanced user, this comprehensive guide will help you understand how to copy and paste ranges like a pro.
The Importance of VBA Copy and Paste Range
Copying and pasting ranges is a common task in Excel, and VBA provides a powerful way to automate this process. By using VBA, you can copy and paste ranges quickly and accurately, saving you time and reducing errors. Moreover, VBA allows you to perform complex tasks, such as copying multiple ranges, pasting data into different worksheets, and formatting cells.
Benefits of VBA Copy and Paste Range
- Increased Productivity: VBA copy and paste range techniques can automate repetitive tasks, freeing up your time to focus on more important tasks.
- Improved Accuracy: By using VBA, you can reduce errors caused by manual copying and pasting.
- Flexibility: VBA allows you to copy and paste ranges in different worksheets, workbooks, and even external applications.
- Customization: You can customize the copy and paste process to suit your specific needs, such as formatting cells or performing calculations.
How to Copy and Paste Range in VBA
To copy and paste a range in VBA, you need to use the Range
object and the Copy
and Paste
methods. Here's a basic example:
Sub CopyAndPasteRange()
' Define the range to copy
Dim sourceRange As Range
Set sourceRange = Range("A1:B2")
' Copy the range
sourceRange.Copy
' Define the destination range
Dim destinationRange As Range
Set destinationRange = Range("C1:D2")
' Paste the range
destinationRange.Paste
End Sub
In this example, we define the source range (A1:B2
) and copy it using the Copy
method. Then, we define the destination range (C1:D2
) and paste the copied range using the Paste
method.
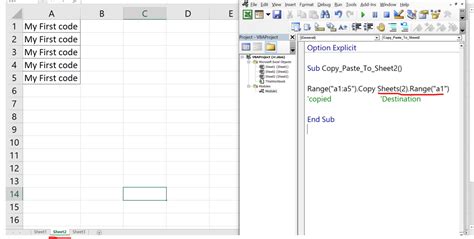
VBA Copy and Paste Range Methods
VBA provides several methods for copying and pasting ranges, including:
- Range.Copy: Copies the range to the clipboard.
- Range.Paste: Pastes the copied range into the destination range.
- Range.PasteSpecial: Pastes the copied range into the destination range with special formatting options.
- Range.ClearContents: Clears the contents of the range.
Best Practices for VBA Copy and Paste Range
- Use explicit references: Always use explicit references to ranges, worksheets, and workbooks to avoid confusion and errors.
- Use meaningful variable names: Use descriptive variable names to make your code more readable and maintainable.
- Avoid using
Select
: Avoid using theSelect
method, which can cause errors and slow down your code. - Test your code: Always test your code to ensure it works as expected.
Common Errors in VBA Copy and Paste Range
- Error 1004: "Method 'Range' of object '_Worksheet' failed." This error occurs when the range is not valid or does not exist.
- Error 91: "Object variable or With block variable not set." This error occurs when the range object is not set properly.
Troubleshooting VBA Copy and Paste Range Errors
To troubleshoot VBA copy and paste range errors, follow these steps:
- Check the range references: Ensure that the range references are correct and valid.
- Check the worksheet and workbook references: Ensure that the worksheet and workbook references are correct and valid.
- Use the debugger: Use the debugger to step through your code and identify the error.
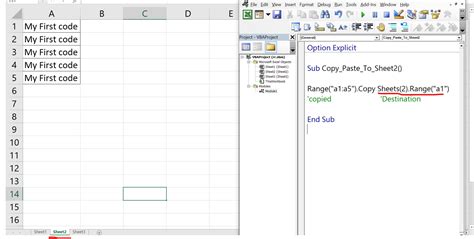
Advanced VBA Copy and Paste Range Techniques
- Copying multiple ranges: You can copy multiple ranges by using the
Range
object and theUnion
method. - Pasting data into different worksheets: You can paste data into different worksheets by using the
Worksheet
object and thePaste
method. - Formatting cells: You can format cells by using the
Range
object and theFont
andInterior
properties.
Conclusion
Mastering VBA copy and paste range techniques is essential for any Excel user who wants to automate tasks and increase productivity. By following the best practices and troubleshooting tips outlined in this article, you can become a pro at copying and pasting ranges in VBA.
We hope this article has been helpful in explaining the concepts of VBA copy and paste range. If you have any questions or need further clarification, please leave a comment below. Don't forget to share this article with your friends and colleagues who may benefit from it.
VBA Copy and Paste Range Gallery
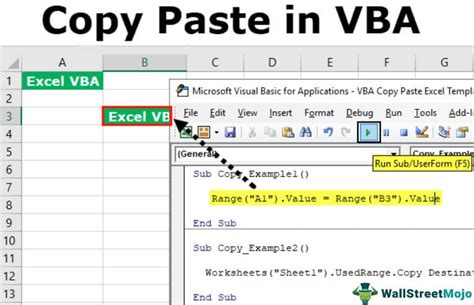
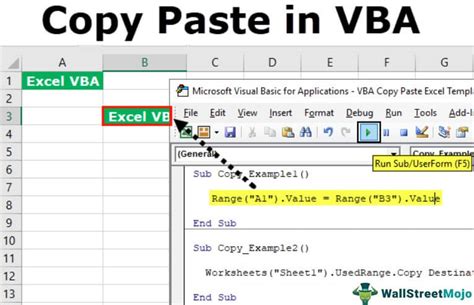
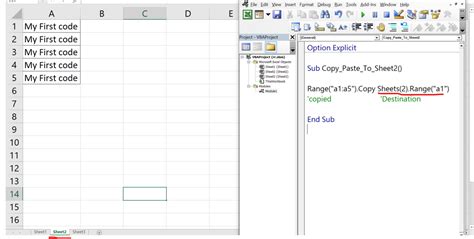
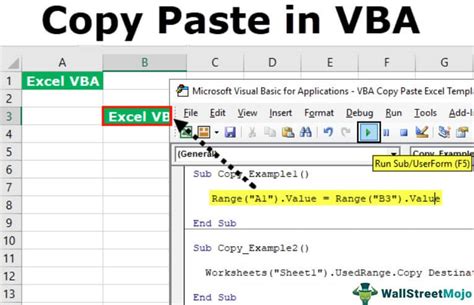
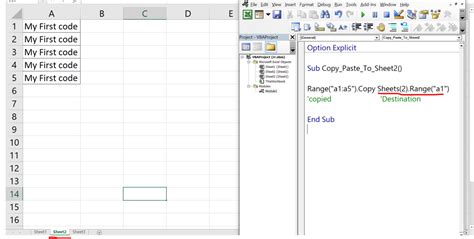
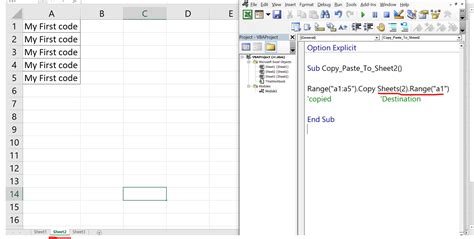
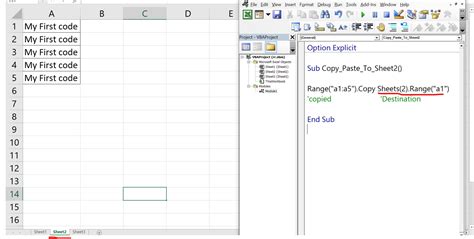
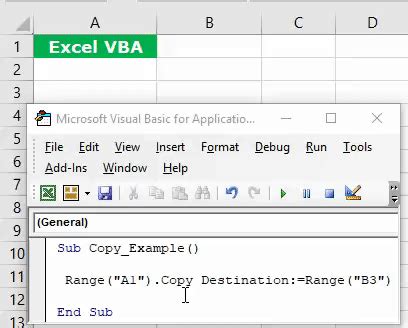
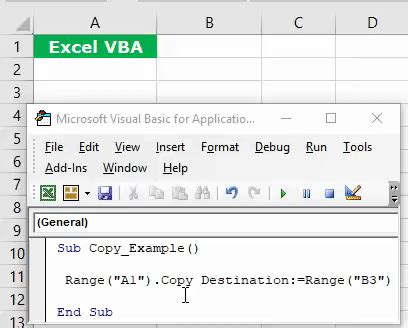
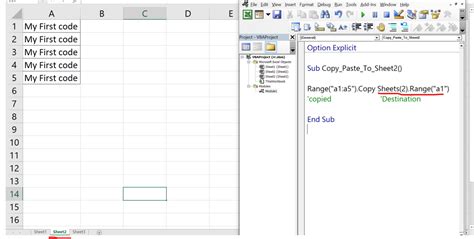