Intro
Unlock the power of VBA functions with array returns. Simplify your code and boost efficiency by mastering the art of returning arrays from VBA functions. Learn how to create, manipulate, and return arrays with ease, and discover tips for error handling and optimization in this comprehensive guide to VBA function return arrays.
VBA Function Return Array: Simplify Your Code
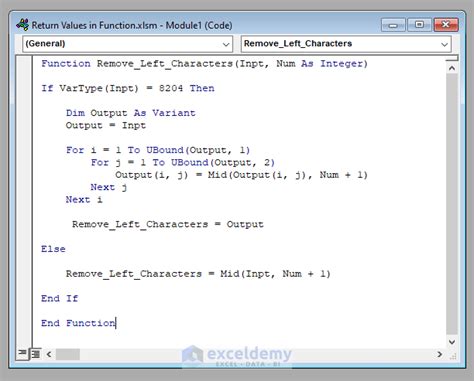
When working with VBA (Visual Basic for Applications), you may find yourself needing to return an array from a function. While VBA doesn't natively support returning arrays from functions, there are workarounds to achieve this. In this article, we'll explore how to return an array from a VBA function and simplify your code.
VBA functions are a powerful tool for automating tasks in Microsoft Office applications, such as Excel, Word, and Access. However, they have limitations, including the inability to directly return arrays. This can make it challenging to work with complex data sets or perform calculations that involve multiple values.
Why Can't VBA Functions Return Arrays?
VBA functions can only return a single value, which is determined by the function's data type. This means that if you try to return an array from a VBA function, you'll encounter a compile-time error. The reason for this limitation is largely historical and technical.
In the early days of VBA, the language was designed to be a simple, procedural programming language for automating Office applications. As a result, it didn't include support for more advanced data structures like arrays. While VBA has evolved over the years, this limitation remains.
Workarounds for Returning Arrays from VBA Functions
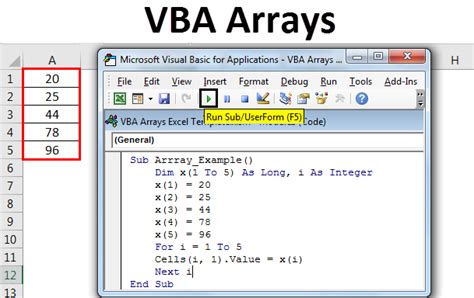
While VBA functions can't directly return arrays, there are several workarounds to achieve this:
1. Use a Variant Data Type
One way to return an array from a VBA function is to use a Variant data type. A Variant is a special data type in VBA that can hold any type of value, including arrays.
Function MyFunction() As Variant
Dim MyArray() As Integer
MyArray = Array(1, 2, 3, 4, 5)
MyFunction = MyArray
End Function
In this example, the MyFunction
function returns a Variant that contains an array of integers.
2. Use a Collection Object
Another way to return an array from a VBA function is to use a Collection object. A Collection is a built-in VBA object that can hold multiple values.
Function MyFunction() As Collection
Dim MyCollection As New Collection
MyCollection.Add 1
MyCollection.Add 2
MyCollection.Add 3
MyCollection.Add 4
MyCollection.Add 5
Set MyFunction = MyCollection
End Function
In this example, the MyFunction
function returns a Collection that contains five values.
3. Use a User-Defined Type (UDT)
A User-Defined Type (UDT) is a custom data type that you can create in VBA. You can use a UDT to return an array from a function.
Type MyType
MyArray() As Integer
End Type
Function MyFunction() As MyType
Dim MyVar As MyType
MyVar.MyArray = Array(1, 2, 3, 4, 5)
MyFunction = MyVar
End Function
In this example, the MyFunction
function returns a UDT that contains an array of integers.
Example Use Cases
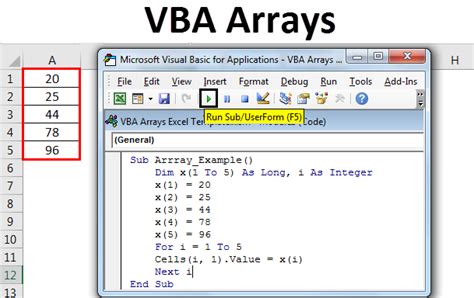
Here are some example use cases for returning arrays from VBA functions:
- Data Analysis: You can use VBA functions to perform complex data analysis tasks, such as calculating statistics or performing data transformations. By returning arrays from these functions, you can easily work with the results in your VBA code.
- Automating Tasks: VBA functions can be used to automate tasks in Office applications, such as Excel or Word. By returning arrays from these functions, you can easily work with the results and perform additional tasks.
- Interfacing with Other Applications: VBA functions can be used to interface with other applications, such as databases or web services. By returning arrays from these functions, you can easily work with the results and perform additional tasks.
Best Practices for Returning Arrays from VBA Functions
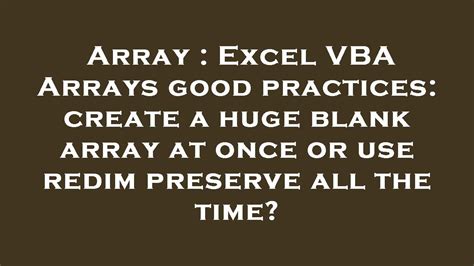
Here are some best practices to keep in mind when returning arrays from VBA functions:
- Use Descriptive Variable Names: Use descriptive variable names to make your code easier to understand. This is especially important when working with arrays, as it can be difficult to keep track of multiple values.
- Use Error Handling: Use error handling to catch any errors that may occur when returning arrays from VBA functions. This can help prevent crashes and make your code more robust.
- Use Type Declarations: Use type declarations to specify the data type of your variables. This can help prevent errors and make your code more maintainable.
Gallery of VBA Array Examples
VBA Array Examples
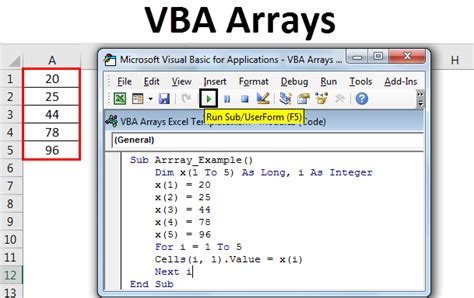
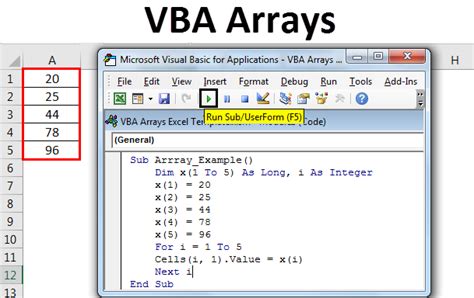
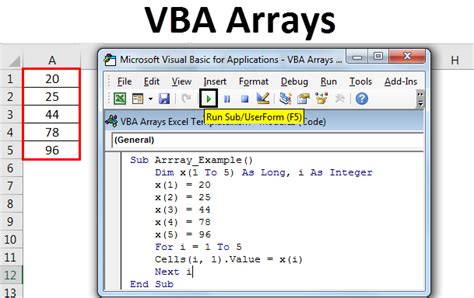
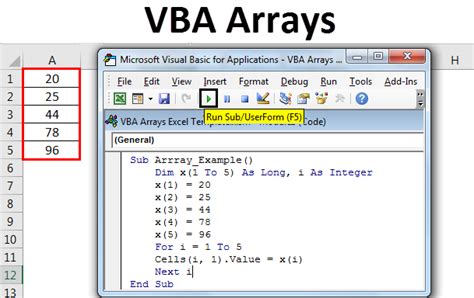
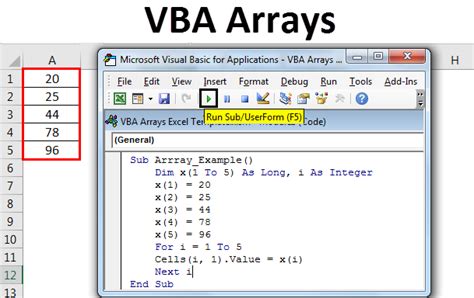
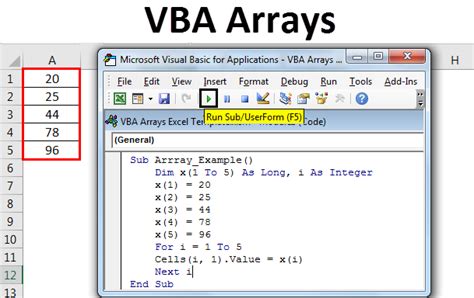
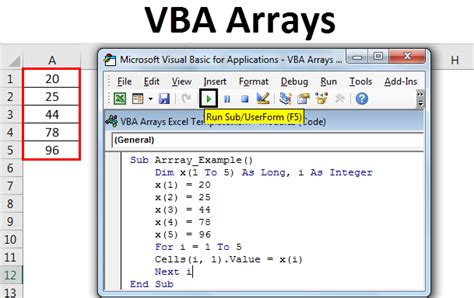
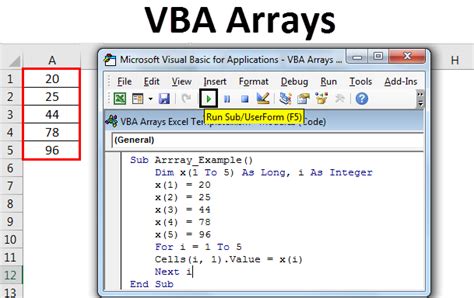
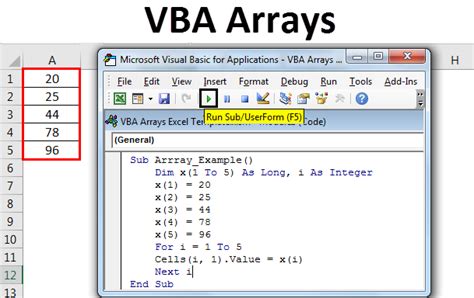
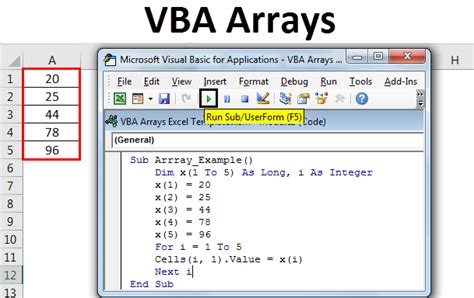
We hope this article has provided you with a comprehensive guide to returning arrays from VBA functions. By following the workarounds and best practices outlined in this article, you can simplify your code and achieve your goals.
Do you have any questions or comments about returning arrays from VBA functions? Please feel free to share them below!