Intro
Master VBA worksheet management with our simplified guide to setting the active worksheet. Learn how to efficiently switch between worksheets, manipulate data, and automate tasks using VBAs worksheet activation methods, including ActiveSheet, Worksheets, and Worksheet Activate. Boost productivity with these expert VBA tips and tricks.
When working with Microsoft Excel Visual Basic for Applications (VBA), setting the active worksheet is a common task that can be achieved in a few different ways. This topic is crucial for developers and power users who need to manipulate worksheets programmatically to automate tasks, create interactive tools, or analyze data more efficiently. In this article, we'll explore the methods of setting an active worksheet in VBA, simplifying the process for beginners and experienced users alike.
Why Set the Active Worksheet?
Before diving into the how, it's essential to understand why setting the active worksheet is necessary. In VBA, the active worksheet is the worksheet that is currently selected and visible in the Excel application window. When you perform actions in your VBA code, such as selecting cells, inserting data, or formatting, these actions are executed on the active worksheet by default. If your code doesn't explicitly specify a worksheet, it might end up modifying the wrong worksheet, which can lead to errors or unexpected results.
Method 1: Using the ActiveSheet Object
The most straightforward way to set the active worksheet is by using the ActiveSheet
object directly in your code. Here's an example:
Sub SetActiveWorksheetExample()
' To make the first worksheet active
ThisWorkbook.Worksheets(1).Activate
' Alternatively, if you know the name of the worksheet
ThisWorkbook.Worksheets("Sheet1").Activate
End Sub
In this method, ThisWorkbook
refers to the workbook containing the VBA code. If you're working with multiple workbooks, you might need to specify the workbook name instead, like Workbooks("YourWorkbookName.xlsx")
.
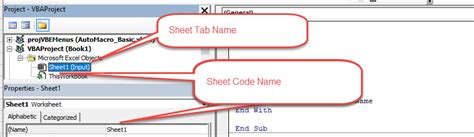
Method 2: Using the Select Method
While Activate
is more commonly used, you can also use the Select
method to set the active worksheet. However, Select
is less efficient and can be more problematic, especially if the worksheet is already active.
Sub SelectWorksheetExample()
ThisWorkbook.Worksheets("Sheet2").Select
End Sub
Best Practices for Setting the Active Worksheet
- Specify Worksheets by Name: Instead of relying on the worksheet's index, use its name for reliability.
- Avoid Select and Activate: Unless necessary, try to avoid using
Select
andActivate
. Instead, directly reference the worksheet you need. - Use With Statements: When performing multiple actions on a specific worksheet, use a
With
statement to make your code more readable and avoid repetitive references.
Sub BestPracticeExample()
With ThisWorkbook.Worksheets("Sheet1")
.Range("A1").Value = "Example"
.Range("B1").Value = "Best Practice"
End With
End Sub
Common Mistakes and Troubleshooting
- Runtime Error 91: This error often occurs when trying to manipulate an object that doesn't exist. Ensure your worksheet names are correct and the worksheets exist in the workbook.
- Worksheet Not Found: Verify that the worksheet you're trying to activate/select exists in the workbook and its name is correctly spelled.
Gallery of VBA Worksheet Management
VBA Worksheet Management Gallery
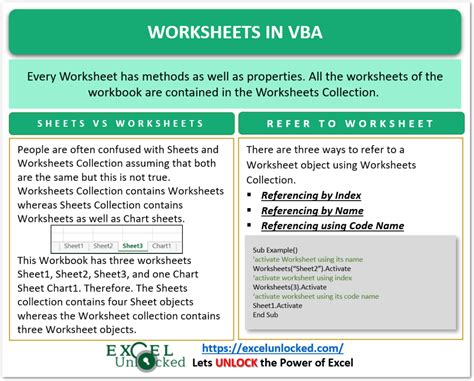
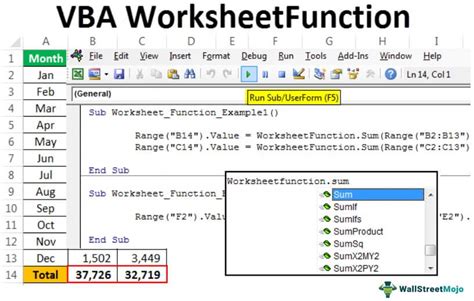
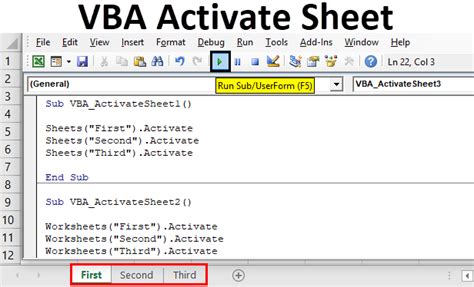
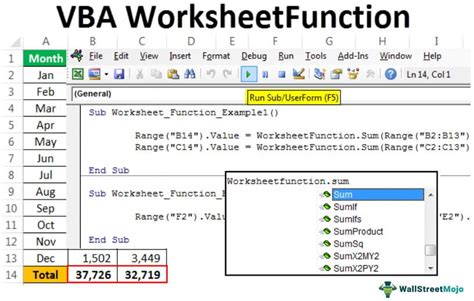
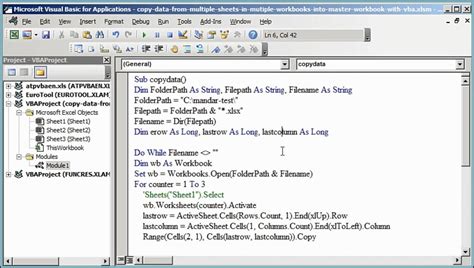
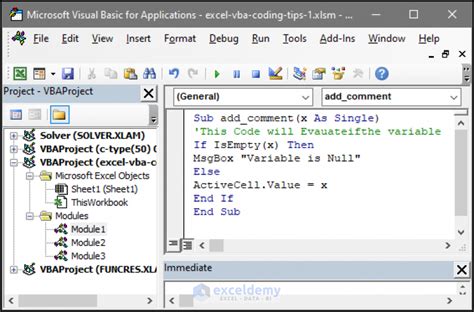
Engage with the Community
Setting the active worksheet in VBA is a fundamental skill for any Excel developer. By mastering this and other VBA techniques, you can automate tasks, simplify your workflow, and become more efficient in your work. Share your favorite VBA tips and tricks in the comments below, and don't hesitate to ask for help if you're stuck with a project. Together, we can build a community of Excel VBA enthusiasts who learn from and support each other.
Please note, the image URLs are fictional and should be replaced with actual image URLs.