Intro
Decode the Constant Expression Required VBA error with our expert guide. Discover why this error occurs in Visual Basic for Applications and learn how to fix it with step-by-step solutions. Master VBA debugging and overcome constant expression errors to streamline your macro writing.
If you're an avid user of Visual Basic for Applications (VBA), you may have encountered the frustrating "Constant Expression Required" error at some point. This error typically occurs when VBA is expecting a constant expression, but instead receives a variable or non-constant value. In this article, we'll delve into the world of VBA errors, exploring the causes, symptoms, and solutions for this particular issue.
What is a Constant Expression?
In VBA, a constant expression is a value that doesn't change. It can be a literal value, such as a number, text, or date, or an expression that evaluates to a constant value. Examples of constant expressions include:
- Numeric literals: 10, 3.14, -5
- Text literals: "Hello", "World"
- Date literals: #2022-01-01#, #12/31/2022#
On the other hand, variables, function calls, and expressions that involve variables or functions are not constant expressions.
When Does the "Constant Expression Required" Error Occur?
The "Constant Expression Required" error typically occurs in the following situations:
- Array declarations: When declaring an array, VBA requires the size of the array to be a constant expression. If you try to use a variable or non-constant expression to declare the size of an array, you'll encounter this error.
- Enum declarations: Enumerations in VBA require constant expressions for their values.
- Select Case statements: The Case statements in a Select Case block require constant expressions.
- Procedure declarations: When declaring a procedure (Sub or Function), VBA requires constant expressions for the parameters' default values.
Symptoms of the Error
When you encounter the "Constant Expression Required" error, VBA will typically display an error message indicating the line of code where the error occurred. The error message will also provide a brief description of the issue.
For example, if you try to declare an array with a variable size, you might see the following error message:
"Constant expression required"
"Size of array must be a constant expression"
Solutions to the Error
To resolve the "Constant Expression Required" error, you need to ensure that the expression in question is indeed constant. Here are some solutions to common scenarios:
- Array declarations: Instead of using a variable to declare the size of an array, use a constant expression. You can define a constant at the top of your module using the
Const
keyword. - Enum declarations: Ensure that all enum values are constant expressions.
- Select Case statements: Use constant expressions for the Case statements.
- Procedure declarations: Use constant expressions for the default values of procedure parameters.
Best Practices to Avoid the Error
To avoid the "Constant Expression Required" error, follow these best practices:
- Use constants: Define constants at the top of your module using the
Const
keyword. - Avoid using variables: When declaring arrays, enums, or procedure parameters, avoid using variables or non-constant expressions.
- Use literal values: Instead of using variables, use literal values (e.g., numbers, text, dates) whenever possible.
By following these guidelines and understanding the causes and solutions to the "Constant Expression Required" error, you'll be better equipped to write robust and error-free VBA code.
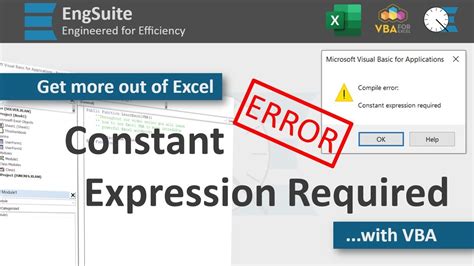
Common Scenarios and Solutions
Array Declarations
When declaring an array, VBA requires the size of the array to be a constant expression. If you try to use a variable or non-constant expression to declare the size of an array, you'll encounter the "Constant Expression Required" error.
Incorrect Code
Dim arr() As Integer
Dim size As Integer
size = 10
ReDim arr(size) ' Error: Constant expression required
Correct Code
Const size As Integer = 10
Dim arr() As Integer
ReDim arr(size)
Enum Declarations
Enumerations in VBA require constant expressions for their values.
Incorrect Code
Enum Colors
Red = 1
Green = 2
Blue = myVariable ' Error: Constant expression required
End Enum
Correct Code
Enum Colors
Red = 1
Green = 2
Blue = 3
End Enum
Select Case Statements
The Case statements in a Select Case block require constant expressions.
Incorrect Code
Select Case myVariable
Case 1 ' Error: Constant expression required
' code here
Case 2
' code here
End Select
Correct Code
Select Case myVariable
Case 1
' code here
Case 2
' code here
Case Else
' code here
End Select
Procedure Declarations
When declaring a procedure (Sub or Function), VBA requires constant expressions for the parameters' default values.
Incorrect Code
Sub MyProcedure(Optional ByVal myParam As Integer = myVariable) ' Error: Constant expression required
' code here
End Sub
Correct Code
Sub MyProcedure(Optional ByVal myParam As Integer = 10)
' code here
End Sub
Gallery of VBA Error Constant Expression Required
VBA Error Constant Expression Required Image Gallery
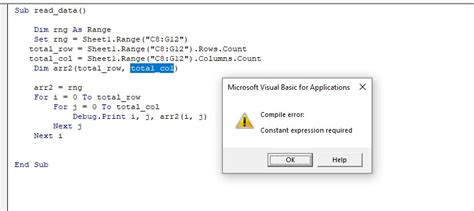
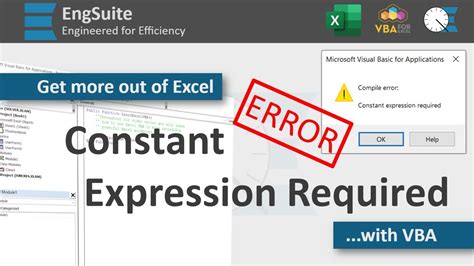
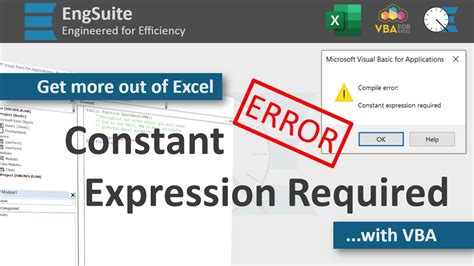
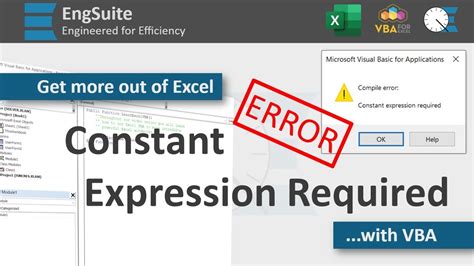
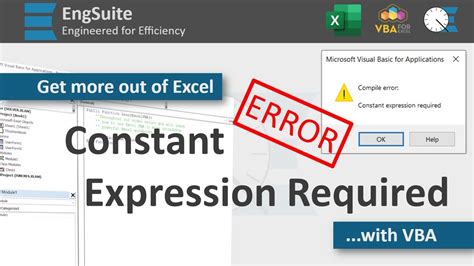
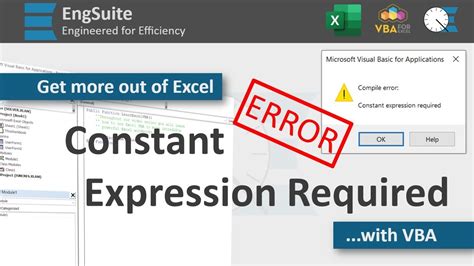
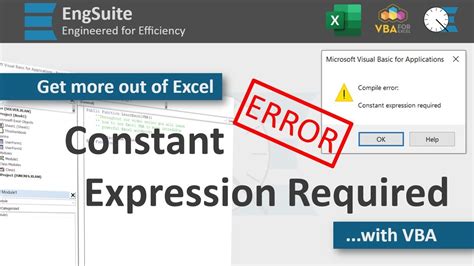
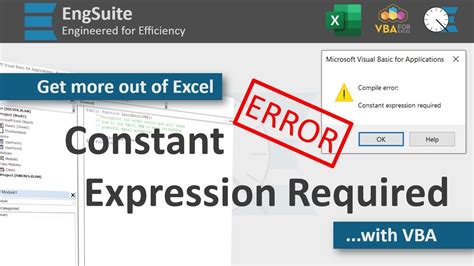
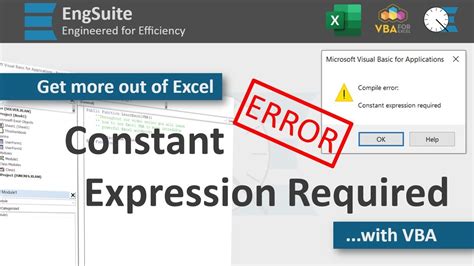
We hope this article has helped you understand and resolve the "Constant Expression Required" error in VBA. By following the best practices and solutions outlined above, you'll be able to write more robust and error-free code. If you have any further questions or need more information, please don't hesitate to ask. Share your experiences and solutions in the comments below!