Intro
Master the art of counting rows in VBA with ease! Discover simple and efficient methods to automate row counting in Excel using Visual Basic for Applications. Learn how to use VBA loops, worksheet functions, and range objects to count rows quickly and accurately, and streamline your workflow with expert tips and tricks.
Counting rows in VBA can seem like a daunting task, especially for those new to programming. However, with the right techniques and tools, it can be made easy and efficient. In this article, we will explore the various methods of counting rows in VBA, including using loops, functions, and built-in Excel formulas.
Why Count Rows in VBA?
Before we dive into the methods of counting rows, let's discuss why it's necessary in the first place. In VBA, rows are used to store data, and knowing the number of rows can be crucial for various tasks, such as:
- Looping through data to perform calculations or formatting
- Creating charts or graphs based on the data
- Writing data to a new worksheet or workbook
- Validating data entry
Method 1: Using a Loop
One of the most basic ways to count rows in VBA is by using a loop. A loop is a repetitive process that continues until a specified condition is met. In this case, we can use a For-Next loop to iterate through each row in a worksheet.
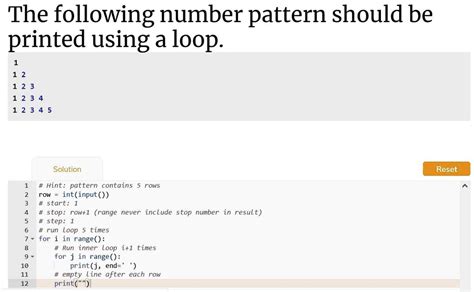
Here's an example code snippet:
Sub CountRowsUsingLoop()
Dim ws As Worksheet
Dim lastRow As Long
Dim i As Long
Set ws = ThisWorkbook.Worksheets("Sheet1")
lastRow = 0
For i = 1 To ws.Rows.Count
If ws.Cells(i, 1).Value <> "" Then
lastRow = i
End If
Next i
MsgBox "Last row with data: " & lastRow
End Sub
Method 2: Using the End(xlDown) Method
Another way to count rows is by using the End(xlDown) method. This method returns the last cell with data in a specified range.
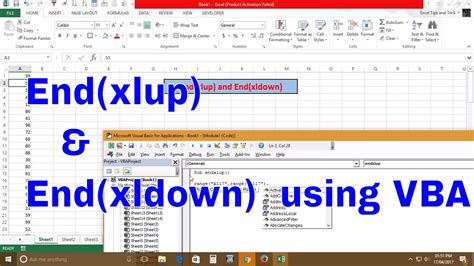
Here's an example code snippet:
Sub CountRowsUsingEndXlDown()
Dim ws As Worksheet
Dim lastRow As Long
Set ws = ThisWorkbook.Worksheets("Sheet1")
lastRow = ws.Cells(ws.Rows.Count, 1).End(xlUp).Row
MsgBox "Last row with data: " & lastRow
End Sub
Method 3: Using the UsedRange Property
The UsedRange property returns the range of cells that contain data in a worksheet. We can use this property to count the number of rows.
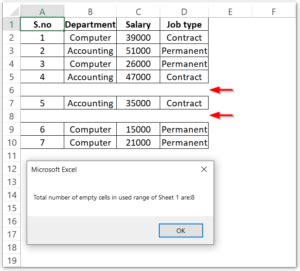
Here's an example code snippet:
Sub CountRowsUsingUsedRange()
Dim ws As Worksheet
Dim lastRow As Long
Set ws = ThisWorkbook.Worksheets("Sheet1")
lastRow = ws.UsedRange.Rows.Count
MsgBox "Last row with data: " & lastRow
End Sub
Method 4: Using the COUNTA Function
The COUNTA function returns the number of cells that contain data in a specified range. We can use this function to count the number of rows.
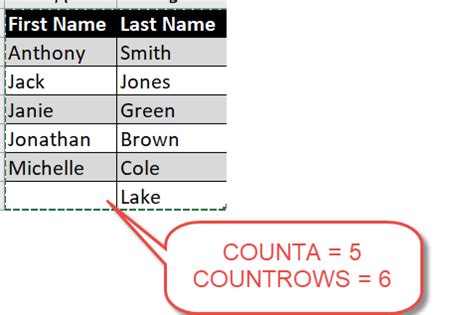
Here's an example code snippet:
Sub CountRowsUsingCOUNTA()
Dim ws As Worksheet
Dim lastRow As Long
Set ws = ThisWorkbook.Worksheets("Sheet1")
lastRow = Application.WorksheetFunction.CountA(ws.Range("A:A"))
MsgBox "Last row with data: " & lastRow
End Sub
Conclusion
Counting rows in VBA can be a straightforward process using various methods. Whether you prefer using loops, functions, or built-in Excel formulas, there's a method that suits your needs. By mastering these techniques, you can efficiently count rows and perform various tasks in VBA.
Gallery of VBA Row Counting
VBA Row Counting Gallery
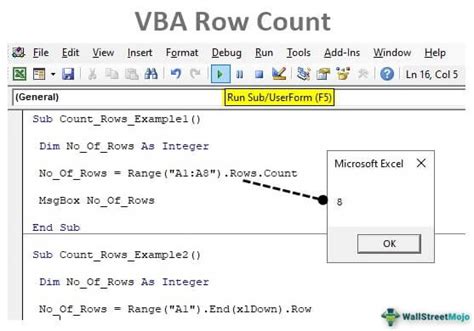
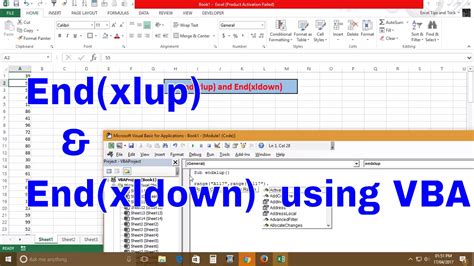
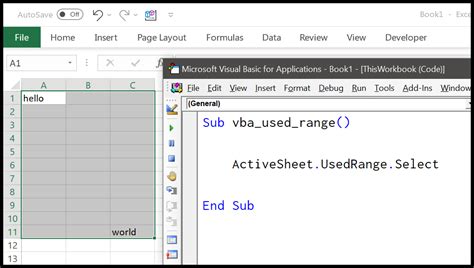
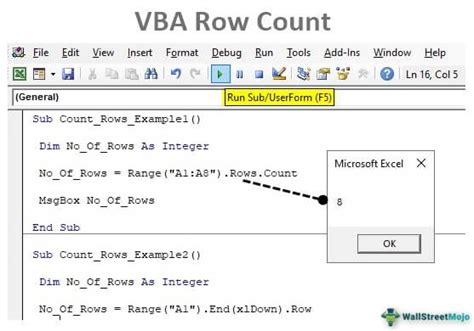
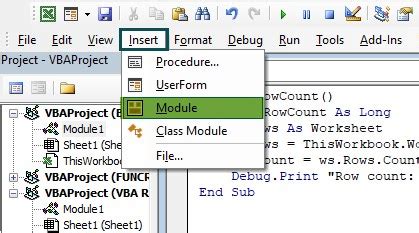
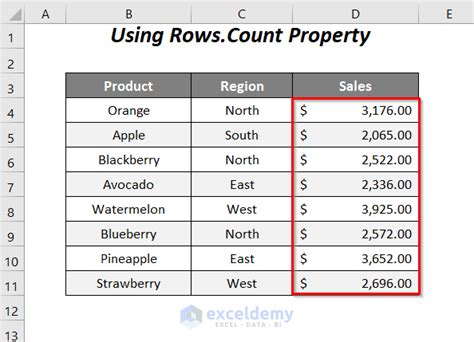
Frequently Asked Questions
- Q: What is the most efficient way to count rows in VBA?
- A: The most efficient way to count rows in VBA depends on the specific use case. However, using the End(xlDown) method or the UsedRange property can be more efficient than using loops.
- Q: Can I use the COUNTA function to count rows in VBA?
- A: Yes, you can use the COUNTA function to count rows in VBA. However, this method may not be suitable for large datasets.
We hope this article has helped you understand the different methods of counting rows in VBA. If you have any further questions or need assistance with a specific project, please don't hesitate to ask.