Intro
Discover how to conceal worksheets in Excel using VBA with our expert guide. Learn 5 ways to hide worksheets, including using VBA code, hiding worksheets from users, and protecting your workbook. Master VBA worksheet hiding techniques, including worksheet visibility, worksheet protection, and Excel VBA macros to enhance your spreadsheet security and organization.
In Microsoft Excel, hiding worksheets can be a useful feature, especially when dealing with sensitive data or complex spreadsheets. While Excel provides a built-in option to hide worksheets, using VBA (Visual Basic for Applications) can offer more flexibility and control over the process. In this article, we'll explore five different ways to hide worksheets in Excel VBA.
The Importance of Hiding Worksheets
Before we dive into the methods, let's quickly discuss why hiding worksheets is important. By hiding worksheets, you can:
- Protect sensitive data from unauthorized access
- Simplify complex spreadsheets by hiding unnecessary sheets
- Prevent accidental modifications to critical data
- Enhance spreadsheet organization and reduce clutter
Method 1: Hiding a Single Worksheet
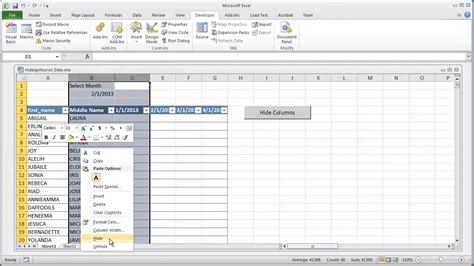
To hide a single worksheet using VBA, you can use the following code:
Sub HideWorksheet()
Worksheets("Sheet1").Visible = False
End Sub
In this example, replace "Sheet1" with the name of the worksheet you want to hide. The Visible
property is set to False
to hide the worksheet.
Method 2: Hiding Multiple Worksheets
To hide multiple worksheets, you can use a loop to iterate through a list of worksheet names. Here's an example:
Sub HideMultipleWorksheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
If ws.Name = "Sheet1" Or ws.Name = "Sheet2" Or ws.Name = "Sheet3" Then
ws.Visible = False
End If
Next ws
End Sub
In this example, replace "Sheet1", "Sheet2", and "Sheet3" with the names of the worksheets you want to hide.
Method 3: Hiding All Worksheets Except One
Sometimes, you may want to hide all worksheets except one. You can use the following code to achieve this:
Sub HideAllWorksheetsExceptOne()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
If ws.Name <> "Sheet1" Then
ws.Visible = False
End If
Next ws
End Sub
In this example, replace "Sheet1" with the name of the worksheet you want to keep visible.
Method 4: Hiding Worksheets Based on a Condition
You can also hide worksheets based on a condition, such as a specific value in a cell. Here's an example:
Sub HideWorksheetsBasedOnCondition()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
If ws.Range("A1").Value = "Hide" Then
ws.Visible = False
End If
Next ws
End Sub
In this example, the worksheet will be hidden if the value in cell A1 is "Hide".
Method 5: Hiding Worksheets Using a User Form
You can also create a user form to hide worksheets interactively. Here's an example:
Sub HideWorksheetsUsingUserForm()
Dim ws As Worksheet
Dim frm As UserForm
Set frm = New UserForm1
frm.Show
If frm.HideWorksheet Then
For Each ws In ThisWorkbook.Worksheets
If ws.Name = frm.WorksheetName Then
ws.Visible = False
End If
Next ws
End If
Unload frm
End Sub
In this example, create a user form with a text box to input the worksheet name and a button to hide the worksheet.
Gallery of Excel VBA Worksheet Hiding
Excel VBA Worksheet Hiding Gallery
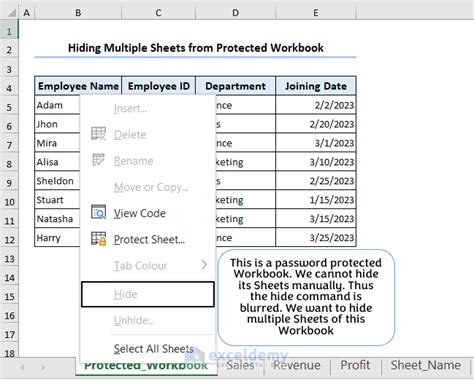
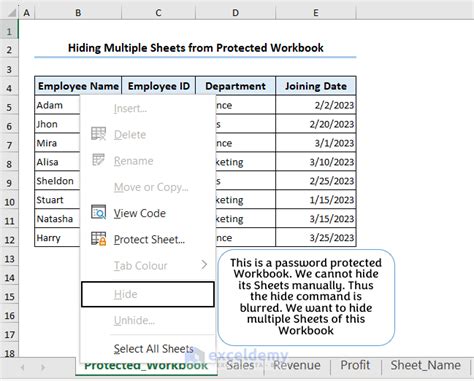
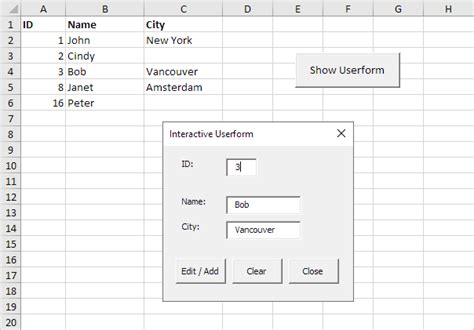
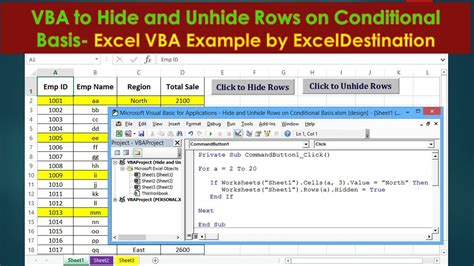
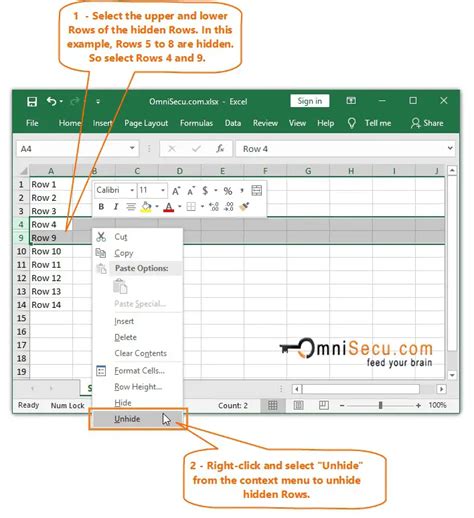
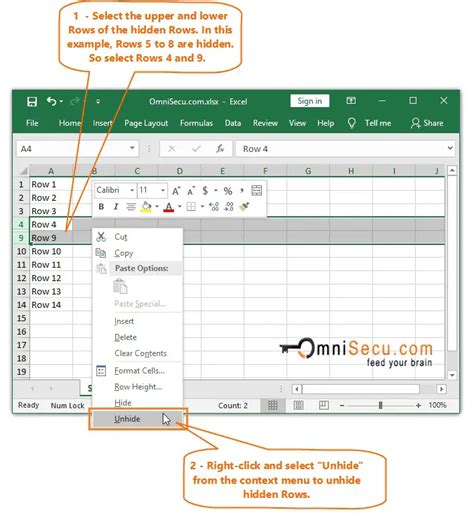
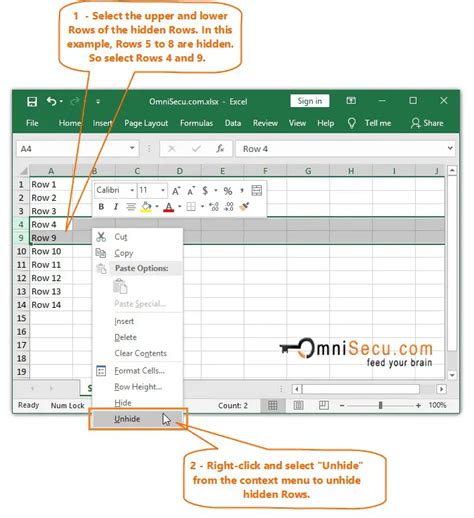
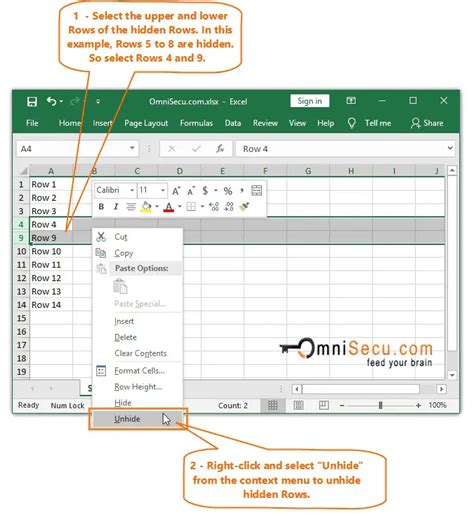
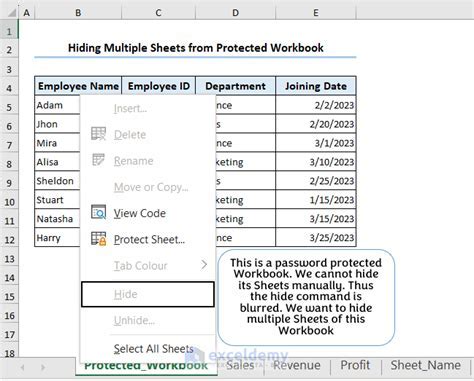
Conclusion
Hiding worksheets in Excel VBA can be a useful feature to protect sensitive data, simplify complex spreadsheets, and enhance spreadsheet organization. By using the methods outlined in this article, you can hide worksheets in various ways, including hiding a single worksheet, multiple worksheets, all worksheets except one, hiding worksheets based on a condition, and hiding worksheets using a user form.