Intro
Discover how to delete rows in Excel with VBA effortlessly. Learn VBA coding techniques to automate row deletion based on specific conditions, errors, or duplicates. Master VBA macro examples, formulas, and shortcuts to streamline your workflow, boost productivity, and manage large datasets with ease. Get step-by-step guides and expert tips inside.
The art of deleting rows in Excel with VBA! It's a crucial skill for any Excel power user or developer. In this article, we'll dive into the world of VBA programming and explore the various ways to delete rows in Excel with ease.
Why Delete Rows in Excel?
Before we dive into the VBA code, let's quickly discuss why you might need to delete rows in Excel. There are several scenarios where deleting rows is necessary:
- Removing duplicate or redundant data
- Deleting empty rows or columns
- Cleaning up data by removing unnecessary rows
- Preparing data for analysis or reporting
Basic VBA Syntax
Before we explore the VBA code, let's cover the basic syntax. To delete rows in Excel using VBA, you'll use the Rows
object and the Delete
method. The basic syntax is as follows:
Rows(row_number).Delete
Where row_number
is the number of the row you want to delete.
Deleting a Single Row
Let's start with a simple example: deleting a single row. Suppose you want to delete row 5. You can use the following VBA code:
Sub DeleteRow()
Rows(5).Delete
End Sub
Deleting Multiple Rows
What if you want to delete multiple rows? You can specify a range of rows using the Rows
object. For example, to delete rows 5 to 10, you can use the following VBA code:
Sub DeleteRows()
Rows("5:10").Delete
End Sub
Deleting Rows Based on Conditions
In many cases, you'll want to delete rows based on specific conditions. For example, you might want to delete rows where the value in column A is blank. You can use the If
statement and the Cells
object to achieve this:
Sub DeleteRowsBasedOnCondition()
Dim i As Long
For i = 1 To 100
If Cells(i, 1).Value = "" Then
Rows(i).Delete
End If
Next i
End Sub
Deleting Rows Using a Loop
Another common scenario is deleting rows using a loop. For example, you might want to delete rows where the value in column A is less than 10. You can use a For
loop and the Cells
object to achieve this:
Sub DeleteRowsUsingLoop()
Dim i As Long
For i = 1 To 100
If Cells(i, 1).Value < 10 Then
Rows(i).Delete
End If
Next i
End Sub
Using the AutoFilter
Method
The AutoFilter
method is another powerful way to delete rows in Excel. You can use the AutoFilter
method to filter the data based on specific conditions and then delete the filtered rows:
Sub DeleteRowsUsingAutoFilter()
Range("A1:A100").AutoFilter Field:=1, Criteria1:="<10"
Range("A1:A100").SpecialCells(xlCellTypeVisible).Delete
End Sub
Gallery of Deleting Rows in Excel
Deleting Rows in Excel VBA Gallery
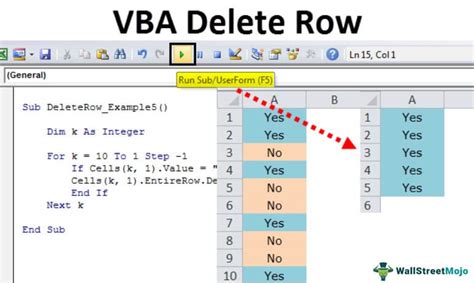
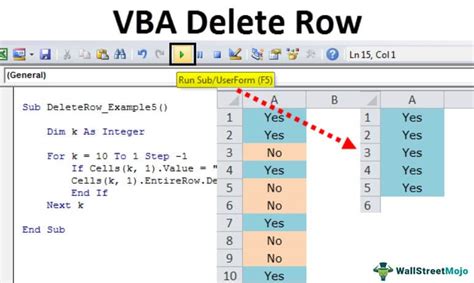
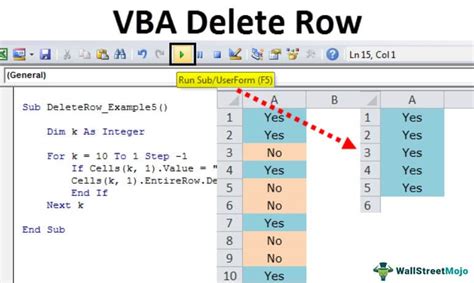
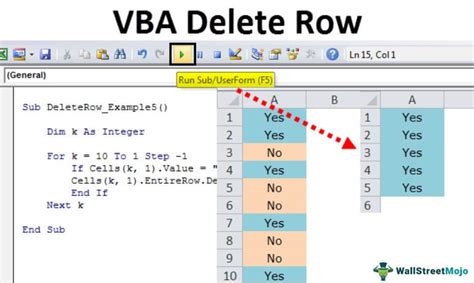
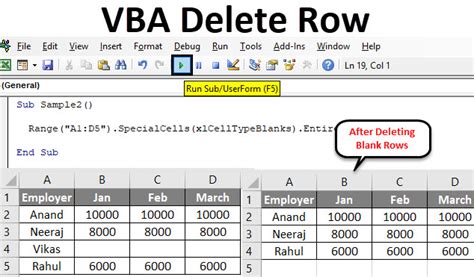
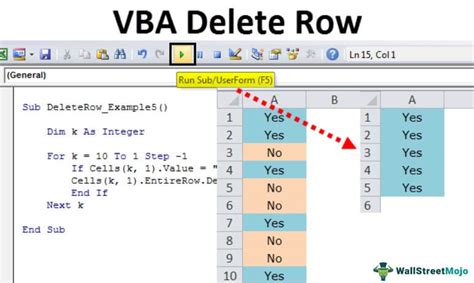
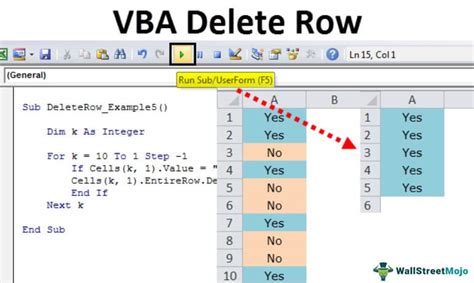
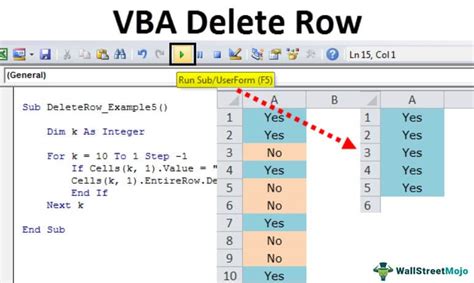
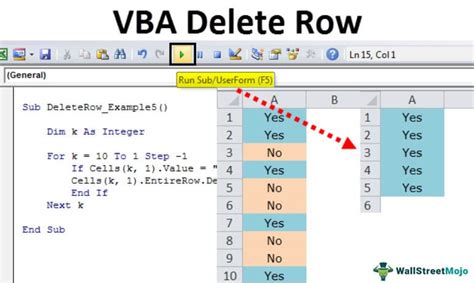
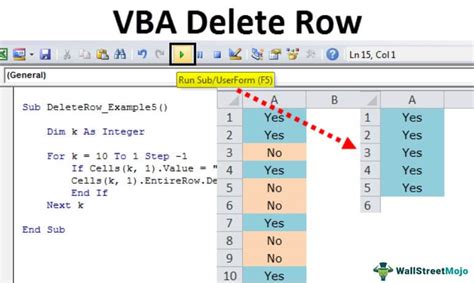
Conclusion
Deleting rows in Excel using VBA is a powerful skill that can save you time and effort. Whether you're a beginner or an advanced user, mastering the art of deleting rows in Excel will take your data manipulation skills to the next level. Remember to use the Rows
object, Delete
method, and AutoFilter
method to achieve your goals. Happy coding!