Intro
Master the art of finding the last row in VBA with ease. Discover how to efficiently identify the last row in Excel using VBA, making data analysis and automation a breeze. Learn expert tips and tricks for finding the last row with VBA, including range-based methods and end-of-data detection, to boost your productivity.
Finding the last row in a spreadsheet can be a daunting task, especially for those new to VBA programming. However, with the right techniques and tools, it can be made easy. In this article, we will explore the different methods for finding the last row in VBA, providing you with a comprehensive guide to make your coding experience more efficient.
Why is Finding the Last Row Important?
Finding the last row in a spreadsheet is crucial for various VBA applications, such as data manipulation, automation, and reporting. It allows you to determine the range of data that needs to be processed, ensuring that your code works accurately and efficiently. Whether you're dealing with a small dataset or a large one, finding the last row is an essential step in your VBA coding journey.
Method 1: Using the Range.Find
Method
One of the most common methods for finding the last row is by using the Range.Find
method. This method searches for a specific value or pattern in a range of cells and returns the range of the first cell that matches the search criteria.
Dim lastRow As Long
lastRow = Cells.Find("*", SearchOrder:=xlByRows, SearchDirection:=xlPrevious).Row
In this example, the Range.Find
method searches for any value ("*"
) in the active worksheet, starting from the top row and moving downwards (xlByRows
). The SearchDirection:=xlPrevious
argument ensures that the search starts from the bottom row and moves upwards, returning the last row with data.
Method 2: Using the Cells.Rows.Count
Property
Another method for finding the last row is by using the Cells.Rows.Count
property. This property returns the total number of rows in the worksheet.
Dim lastRow As Long
lastRow = Cells.Rows.Count
However, this method has a significant drawback: it returns the total number of rows in the worksheet, not the last row with data. To overcome this limitation, you can use the Cells.Rows.Count
property in conjunction with a loop that checks for blank rows.
Dim lastRow As Long
For lastRow = Cells.Rows.Count To 1 Step -1
If Not IsEmpty(Cells(lastRow, 1).Value) Then
Exit For
End If
Next lastRow
In this example, the loop starts from the bottom row and moves upwards, checking for blank rows. When it finds a non-blank row, it exits the loop, returning the last row with data.
Method 3: Using the Worksheet.UsedRange
Property
The Worksheet.UsedRange
property returns the range of cells that contain data in the worksheet.
Dim lastRow As Long
lastRow = ActiveSheet.UsedRange.Rows.Count
This method is more efficient than the previous ones, as it directly returns the range of cells with data. However, it has some limitations, such as not working correctly with hidden rows or columns.
Method 4: Using the Range.CurrentRegion
Property
The Range.CurrentRegion
property returns the range of cells that surround the active cell, including any adjacent data.
Dim lastRow As Long
lastRow = ActiveCell.CurrentRegion.Rows.Count
This method is similar to the Worksheet.UsedRange
property, but it's more flexible, as it allows you to specify a range of cells.
Best Practices for Finding the Last Row
When finding the last row in VBA, it's essential to follow some best practices to ensure accuracy and efficiency:
- Always specify the worksheet or range of cells to avoid errors.
- Use the
Range.Find
method orCells.Rows.Count
property with caution, as they may return incorrect results if the data is not uniform. - Consider using the
Worksheet.UsedRange
property orRange.CurrentRegion
property for more accurate results. - Avoid using hard-coded row numbers, as they may change over time.
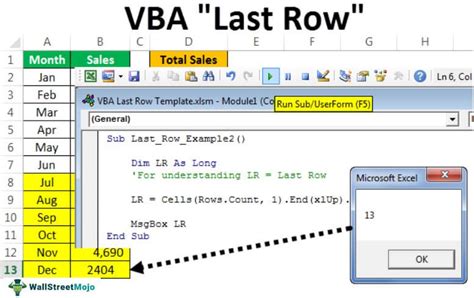
Conclusion
Finding the last row in VBA is a crucial step in various applications, and with the right techniques, it can be made easy. By understanding the different methods and best practices, you can ensure accuracy and efficiency in your coding journey. Remember to always specify the worksheet or range of cells, use caution with certain methods, and consider using the Worksheet.UsedRange
property or Range.CurrentRegion
property for more accurate results.
Gallery of Finding Last Row in VBA
Finding Last Row in VBA Image Gallery
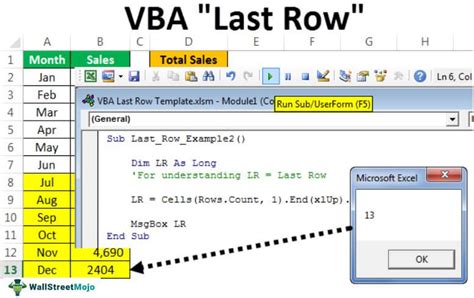
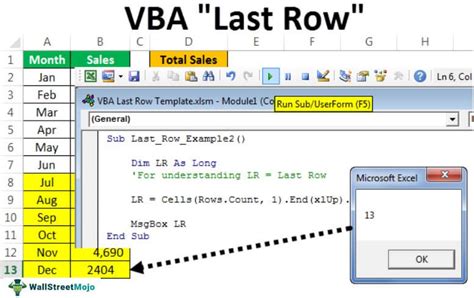
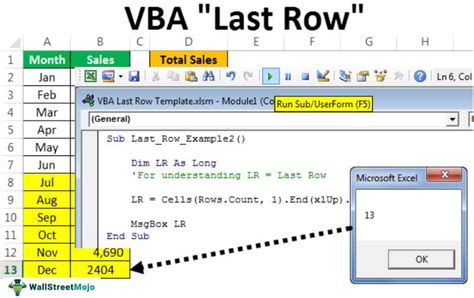
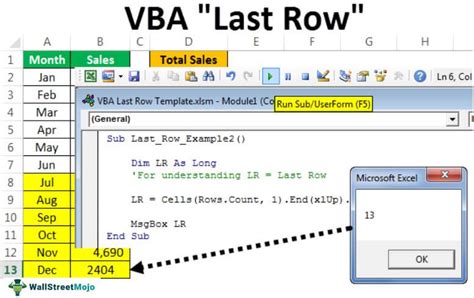
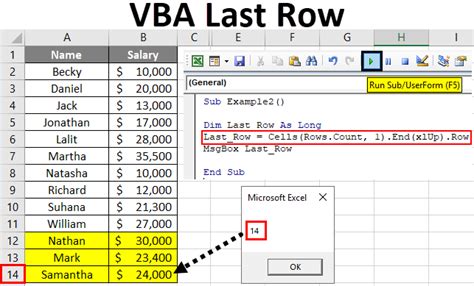
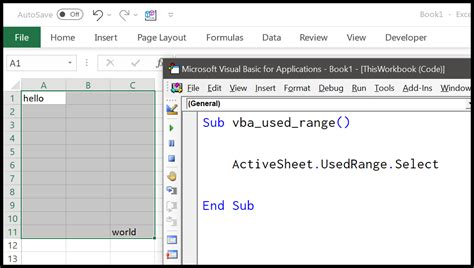
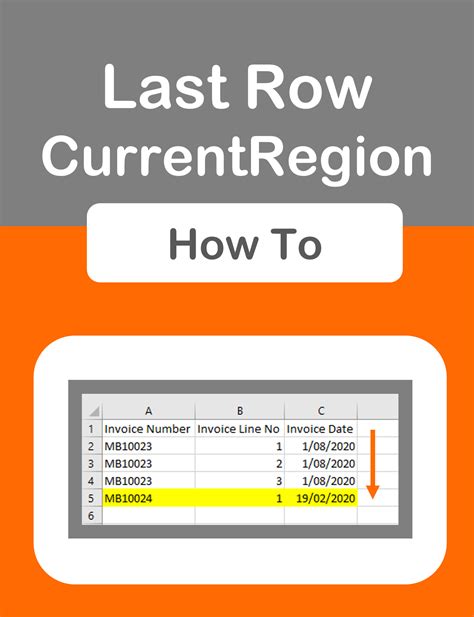
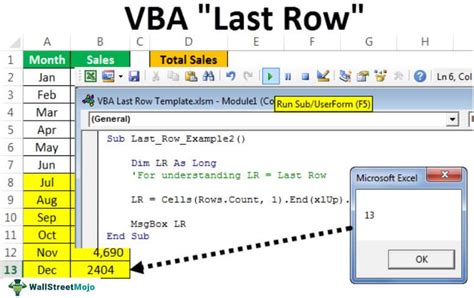
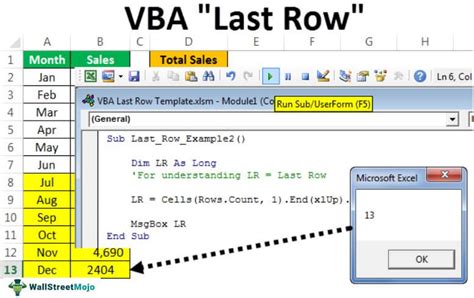
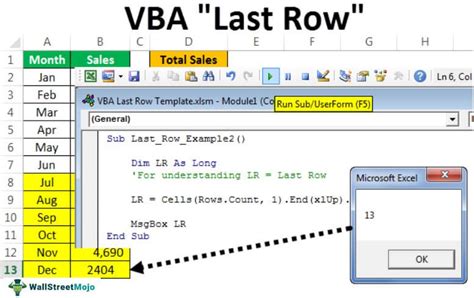
Frequently Asked Questions
- What is the most efficient method for finding the last row in VBA?
- How can I avoid errors when finding the last row in VBA?
- What is the difference between the
Range.Find
method andCells.Rows.Count
property?
We hope this article has provided you with a comprehensive guide to finding the last row in VBA. If you have any further questions or need more clarification, please don't hesitate to ask.