Intro
Master error handling in VBA with ease using the On Error Goto statement. Learn how to efficiently manage runtime errors, handle exceptions, and debug your code with precision. Discover best practices for implementing error handling routines and streamline your VBA development process with this comprehensive guide.
Error handling is a crucial aspect of programming, and in VBA (Visual Basic for Applications), it's no exception. When it comes to managing errors in VBA, one of the most popular and effective methods is using the "On Error Goto" statement. In this article, we'll explore the ins and outs of error handling in VBA, and how "On Error Goto" can make your life easier.
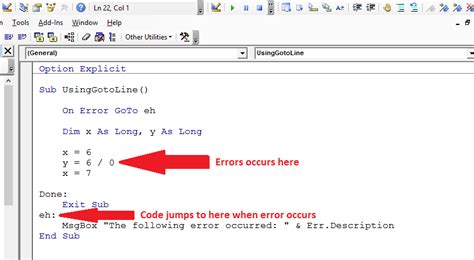
What is Error Handling in VBA?
Error handling in VBA refers to the process of anticipating and managing errors that may occur during the execution of your code. Errors can arise from various sources, such as invalid user input, runtime errors, or bugs in your code. Without proper error handling, your application may crash, or worse, produce incorrect results.
Why is Error Handling Important in VBA?
Error handling is essential in VBA for several reasons:
- Prevents Application Crashes: Error handling helps prevent your application from crashing due to runtime errors, ensuring a better user experience.
- Provides Useful Error Messages: By handling errors, you can provide informative error messages that help users understand what went wrong and how to fix it.
- Ensures Data Integrity: Error handling helps maintain data integrity by preventing incorrect data from being written to databases or files.
What is On Error Goto in VBA?
"On Error Goto" is a VBA statement that allows you to specify a specific line of code to execute when an error occurs. This statement is used in conjunction with a label or a line number, which indicates the starting point of the error-handling code.
Syntax:
On Error GoTo Label
Example:
Sub MyProcedure()
On Error GoTo ErrorHandler
' Code that may raise an error
Exit Sub
ErrorHandler:
MsgBox "An error occurred: " & Err.Description
End Sub
How Does On Error Goto Work?
When an error occurs, the VBA runtime environment checks if an "On Error Goto" statement is present in the current procedure. If it is, the execution jumps to the specified label or line number, and the error-handling code is executed.
Here's a step-by-step breakdown of the process:
- Error Occurs: An error occurs during the execution of your code.
- VBA Checks for On Error Goto: The VBA runtime environment checks if an "On Error Goto" statement is present in the current procedure.
- Execution Jumps to Error Handler: If an "On Error Goto" statement is found, the execution jumps to the specified label or line number.
- Error-Handling Code Executes: The error-handling code is executed, which can include error messages, logging, or recovery actions.
Best Practices for Using On Error Goto
Here are some best practices to keep in mind when using "On Error Goto":
- Use Meaningful Labels: Use descriptive labels that indicate the purpose of the error-handling code.
- Keep Error Handlers Separate: Keep error-handling code separate from the main code to improve readability and maintainability.
- Use Exit Sub/Function: Use "Exit Sub" or "Exit Function" statements to ensure that the error-handling code is executed only when an error occurs.
Benefits of Using On Error Goto
Using "On Error Goto" in VBA offers several benefits, including:
- Improved Error Handling: "On Error Goto" allows you to handle errors in a more structured and centralized way, making it easier to manage and maintain your code.
- Reduced Code Duplication: By using a single error-handling routine, you can reduce code duplication and improve code reusability.
- Better Error Messages: "On Error Goto" enables you to provide more informative error messages, which can help users understand what went wrong and how to fix it.
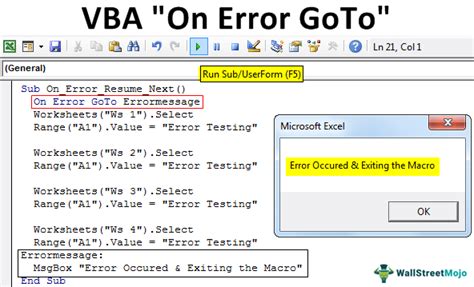
Common Errors in VBA
Here are some common errors that can occur in VBA:
- Type Mismatch: Occurs when you try to assign a value of the wrong data type to a variable.
- Overflow: Occurs when a calculation exceeds the maximum value that can be stored in a variable.
- Out of Range: Occurs when you try to access an array or collection element that is outside the valid range.
Tips for Debugging VBA Code
Here are some tips for debugging VBA code:
- Use the Immediate Window: The Immediate window allows you to execute VBA code and inspect variables in real-time.
- Set Breakpoints: Set breakpoints to pause execution at specific points in your code, making it easier to inspect variables and debug.
- Use Debug.Print: Use "Debug.Print" statements to output values to the Immediate window, helping you understand the flow of your code.
Conclusion
Error handling is a critical aspect of VBA programming, and "On Error Goto" is a powerful tool for managing errors in your code. By using "On Error Goto," you can improve error handling, reduce code duplication, and provide better error messages. Remember to use meaningful labels, keep error handlers separate, and use "Exit Sub/Function" statements to ensure that your error-handling code is executed correctly.
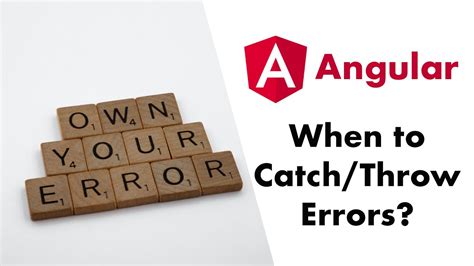
Gallery of Error Handling in VBA
Error Handling in VBA Image Gallery
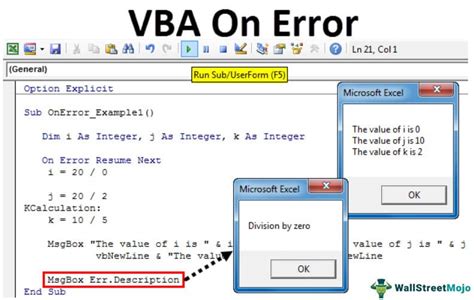
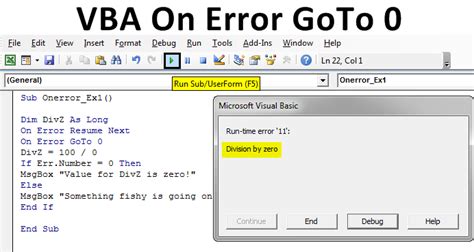
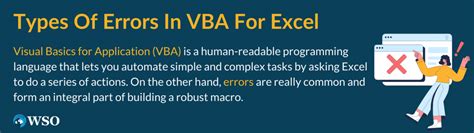
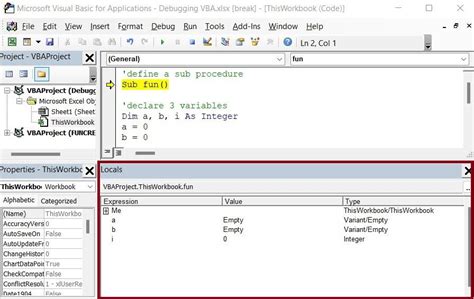
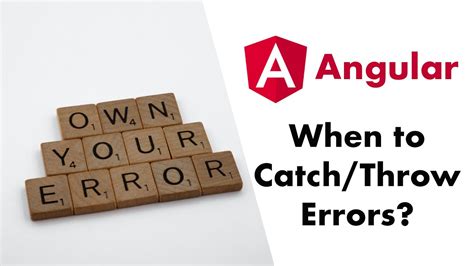
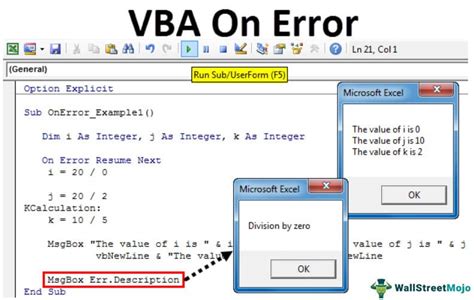

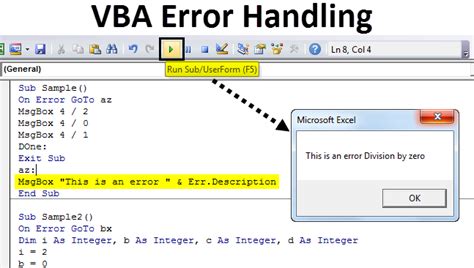
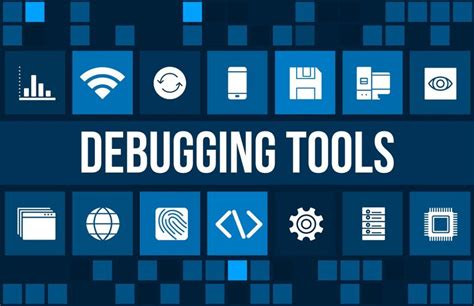
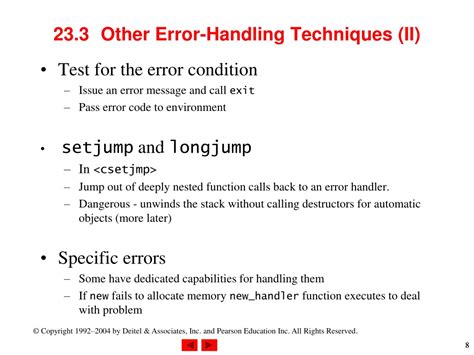
Share your thoughts on error handling in VBA and how you use "On Error Goto" in your code. Do you have any favorite error-handling techniques or best practices? Let's discuss in the comments below!