Intro
Master text manipulation in VBA with these 5 expert ways to split text with a new line. Learn how to insert line breaks, split strings, and format text using VBA code. Discover the power of VBA text functions, including Split(), Mid(), and Chr() to streamline your workflow. Optimize your text processing with these actionable VBA tips.
In VBA, splitting text into multiple lines can be achieved through various methods. This functionality is crucial for tasks such as data manipulation, report generation, and user interface management. Here are five ways to split text with a new line in VBA, each serving different purposes and use cases.
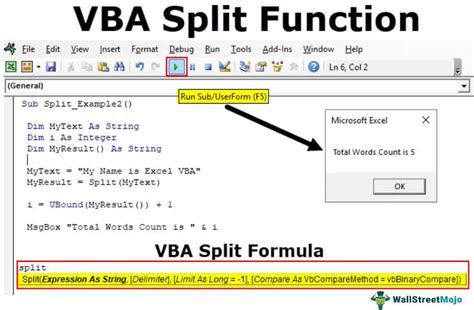
Using the VBA Chr Function
The Chr function returns the character represented by a specific ASCII code. The ASCII code for a line break is 10 (vbLf) for a line feed and 13 (vbCr) for a carriage return. You can use these to insert a new line into your text.
Example Using Chr Function
Dim OriginalText As String
OriginalText = "Hello World"
Dim ModifiedText As String
ModifiedText = OriginalText & Chr(13) & Chr(10) & "This is on a new line."
Debug.Print ModifiedText
Using VBA Constants vbLf and vbCrLf
VBA provides constants for line feed (vbLf) and carriage return line feed (vbCrLf), making it easier to insert new lines without having to remember the ASCII codes.
Example Using VBA Constants
Dim OriginalText As String
OriginalText = "Hello World"
Dim ModifiedText As String
ModifiedText = OriginalText & vbCrLf & "This is on a new line."
Debug.Print ModifiedText
Splitting Text into an Array
Sometimes, you might want to split a text into an array based on a specific delimiter, including new lines. The Split function in VBA can achieve this.
Example Splitting Text into an Array
Dim OriginalText As String
OriginalText = "Line 1" & vbCrLf & "Line 2" & vbCrLf & "Line 3"
Dim LinesArray() As String
LinesArray = Split(OriginalText, vbCrLf)
For i = LBound(LinesArray) To UBound(LinesArray)
Debug.Print LinesArray(i)
Next i
Using Replace Function
The Replace function can be used to insert new lines by replacing specific strings or characters with a line break.
Example Using Replace Function
Dim OriginalText As String
OriginalText = "Hello,World"
Dim ModifiedText As String
ModifiedText = Replace(OriginalText, ",", "," & vbCrLf)
Debug.Print ModifiedText
Reading Text Files with New Lines
When reading text files, VBA automatically handles line breaks. You can use the Line Input statement to read a file line by line.
Example Reading Text Files with New Lines
Open "C:\example.txt" For Input As #1
While Not EOF(1)
Line Input #1, LineText
Debug.Print LineText
Wend
Close #1
Split Text With New Line In VBA Image Gallery
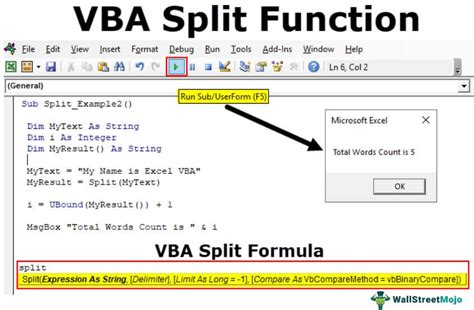
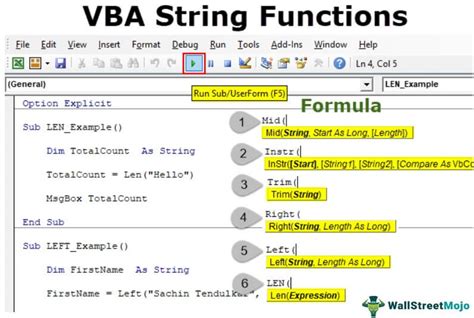
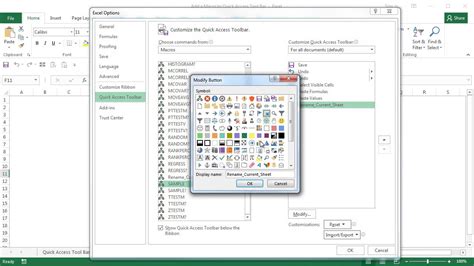
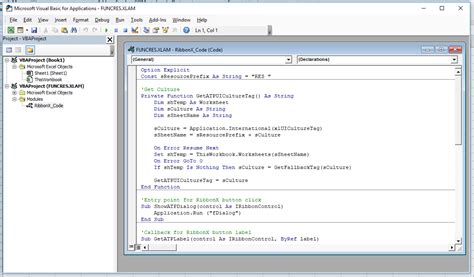
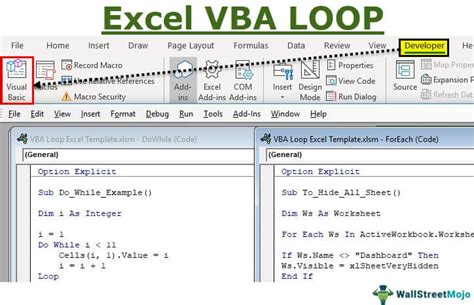
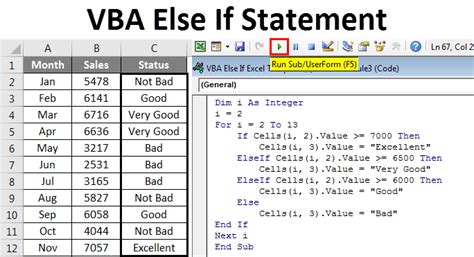
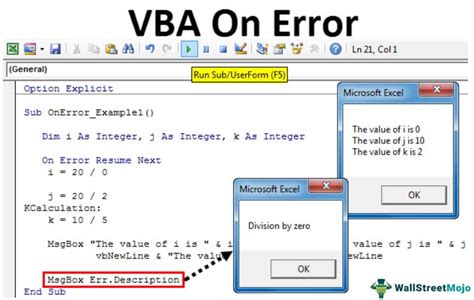
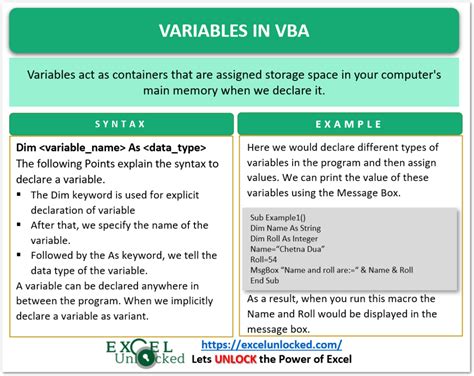
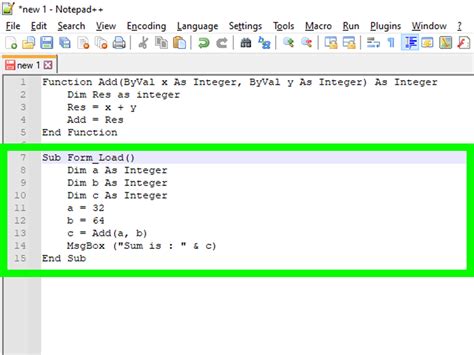
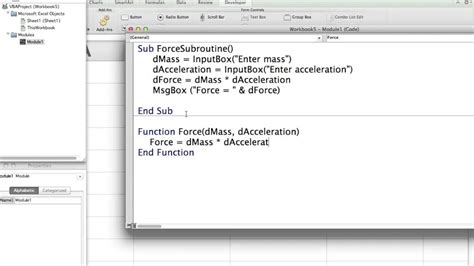
In conclusion, handling new lines in VBA text is crucial for various applications, from data manipulation to user interface design. By mastering the methods outlined above, you can efficiently manage text within your VBA projects, ensuring that your code is both readable and effective.