Intro
Master VBA array creation in minutes! Learn how to quickly create and manipulate arrays in Visual Basic for Applications, including declaring, populating, and resizing arrays. Discover techniques for working with array formulas, array functions, and array loops. Boost your Excel productivity with efficient VBA array programming.
Working with arrays in VBA can be a powerful tool for storing and manipulating data. In this article, we will explore the basics of creating arrays in VBA and provide practical examples to help you get started.
Why Use Arrays in VBA?
Before we dive into creating arrays, let's briefly discuss why you might want to use them in your VBA projects. Arrays offer several advantages, including:
- Efficient data storage: Arrays allow you to store multiple values in a single variable, making it easier to manage and manipulate large datasets.
- Improved performance: By storing data in an array, you can reduce the number of times you need to access external data sources, resulting in faster execution times.
- Simplified code: Arrays can simplify your code by reducing the need for multiple variables and making it easier to loop through data.
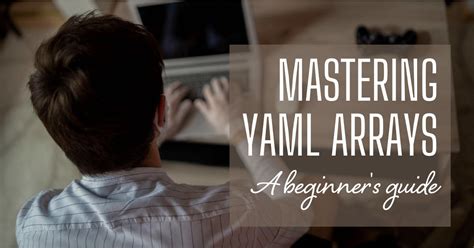
Declaring Arrays in VBA
To create an array in VBA, you need to declare it using the Dim
statement. The basic syntax for declaring an array is as follows:
Dim MyArray() As DataType
In this example, MyArray
is the name of the array, and DataType
is the data type of the values you want to store in the array. The parentheses ()
indicate that MyArray
is an array.
Dynamic vs. Fixed-Size Arrays
VBA supports two types of arrays: dynamic and fixed-size. Dynamic arrays can be resized at runtime, while fixed-size arrays have a fixed size that cannot be changed.
To declare a fixed-size array, you specify the size in the parentheses:
Dim MyArray(10) As Integer
In this example, MyArray
is a fixed-size array that can store 11 integers (remember that array indices start at 0).
To declare a dynamic array, you omit the size:
Dim MyArray() As Integer
You can then use the ReDim
statement to resize the array at runtime:
ReDim MyArray(10)
Initializing Arrays
Once you've declared an array, you can initialize it using the =
operator. For example:
Dim MyArray(10) As Integer
MyArray = Array(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11)
This code initializes MyArray
with the values 1 through 11.
Working with Arrays
Arrays support various operations, including indexing, looping, and sorting. Here are a few examples:
-
Indexing: You can access individual elements of an array using the index operator
()
. For example:MyArray(0) = 10
-
Looping: You can loop through an array using a
For
loop or aFor Each
loop. For example:
For i = 0 To UBound(MyArray) Debug.Print MyArray(i) Next i
* Sorting: You can sort an array using the `Sort` function from the `Excel` library or a custom sorting algorithm.
**Best Practices for Working with Arrays**
Here are some best practices to keep in mind when working with arrays in VBA:
* Use meaningful variable names: Choose names that clearly indicate the purpose and contents of the array.
* Declare arrays with the correct data type: Make sure the data type matches the type of data you're storing in the array.
* Use `ReDim` instead of `Redim`: The `ReDim` statement is more efficient and flexible than the `Redim` statement.
* Avoid using `Variant` arrays: While `Variant` arrays can store multiple data types, they can lead to errors and performance issues.
**Common Errors When Working with Arrays**
Here are some common errors to watch out for when working with arrays in VBA:
* Out-of-bounds errors: Make sure you're not trying to access an index that's outside the bounds of the array.
* Type mismatch errors: Ensure that the data type of the values you're storing in the array matches the declared data type.
* Null or empty arrays: Check that the array has been initialized and populated before trying to access its elements.
**Conclusion**
In this article, we've covered the basics of creating arrays in VBA, including declaring arrays, initializing arrays, and working with arrays. By following best practices and avoiding common errors, you can harness the power of arrays to simplify your code and improve performance.
**FAQs**
* What is the difference between a dynamic and fixed-size array in VBA?
* A dynamic array can be resized at runtime, while a fixed-size array has a fixed size that cannot be changed.
* How do I declare an array in VBA?
* Use the `Dim` statement, followed by the array name and data type.
* What is the purpose of the `ReDim` statement in VBA?
* The `ReDim` statement is used to resize a dynamic array at runtime.
Array Operations Image Gallery
If you have any questions or need further clarification on any of the topics covered in this article, please don't hesitate to ask.