Intro
Master copying and pasting in VBA with precision! Learn 5 efficient ways to paste without formatting in VBA, ensuring seamless data transfer. Discover how to avoid formatting issues, preserve original content, and streamline your coding workflow using VBAs Range, PasteSpecial, and other advanced techniques for accurate data pasting.
When working with VBA, it's common to need to paste data without formatting, especially when dealing with large datasets or when the formatting might interfere with further processing. The ability to paste values only or text without any extraneous formatting is crucial for maintaining data integrity and ensuring that your macros run smoothly. Here are five ways to achieve this in VBA, catering to different scenarios and needs.
Understanding the Basics
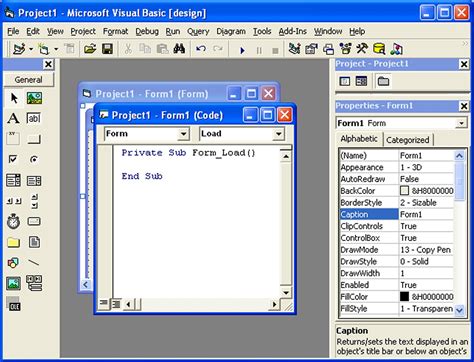
Before diving into the methods, it's essential to understand the basics of VBA and Excel. VBA (Visual Basic for Applications) is a programming language used for creating and automating tasks in Microsoft Office applications like Excel. Excel, being a powerful spreadsheet software, allows for a wide range of formatting options, which can sometimes get in the way of data manipulation.
Method 1: Using the PasteSpecial Method
One of the most straightforward methods to paste without formatting is by using the PasteSpecial
method in VBA. This method allows you to specify what you want to paste, including values, formats, or even just comments.
Sub PasteValuesOnly()
'Select the range you want to copy
Range("A1").Copy
'Select where you want to paste
Range("B1").Select
'Paste values only
Selection.PasteSpecial Paste:=xlPasteValues, Operation:=xlNone, SkipBlanks:=False, Transpose:=False
Application.CutCopyMode = False
End Sub
Method 2: Assigning Values Directly
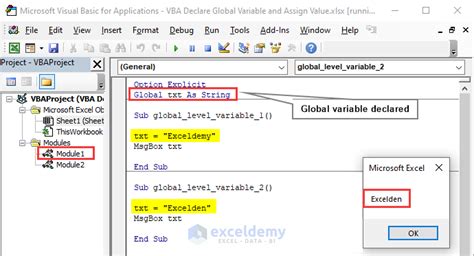
Another efficient way to paste without formatting is by directly assigning values from one range to another. This method bypasses the clipboard, making it faster and more efficient, especially for large datasets.
Sub AssignValuesDirectly()
'Assign values from one range to another
Range("B1").Value = Range("A1").Value
End Sub
Method 3: Using the Value Property
The Value
property in VBA can be used to set or get the value of a range without its formatting. This method is particularly useful when you need to paste data into a range that already has formatting, as it will overwrite the existing formatting with the new values.
Sub SetValueProperty()
'Set the value property of a range
Range("B1").Value = Range("A1").Value
'Alternatively, you can directly set the value
Range("B1").Value = "New Value"
End Sub
Method 4: Utilizing the TextToColumns Method
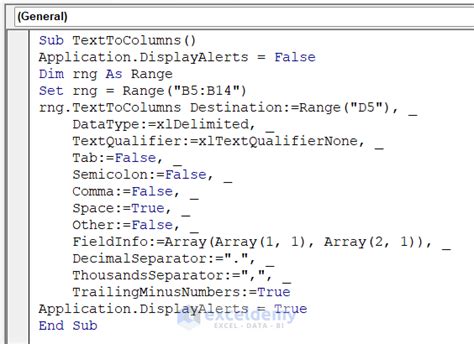
If you're dealing with text data that needs to be split into separate columns, the TextToColumns
method can be a powerful tool. It allows you to specify delimiters and parsing options, giving you fine-grained control over the import process.
Sub TextToColumnsExample()
'Text to columns example
Range("A1").TextToColumns Destination:=Range("B1"), DataType:=xlDelimited, TextQualifier:=xlDoubleQuote, ConsecutiveDelimiter:=False, Tab:=False, Semicolon:=False, Comma:=False, Space:=False, Other:=False, OtherChar:=getti _
ng, FieldInfo:=Array(Array(1, 1)), TrailingMinusNumbers:=True
End Sub
Method 5: Creating a Custom Function
For scenarios that require more flexibility or when the above methods don't fit your needs, creating a custom function can be the way to go. This approach allows you to define your own logic for pasting without formatting, giving you the utmost control over the process.
Function PasteWithoutFormatting(source As Range, destination As Range) As Boolean
'Custom function logic here
'Return True if successful, False otherwise
End Function
Gallery of VBA Paste Without Formatting
VBA Paste Without Formatting Image Gallery
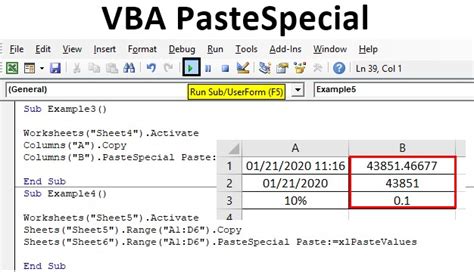
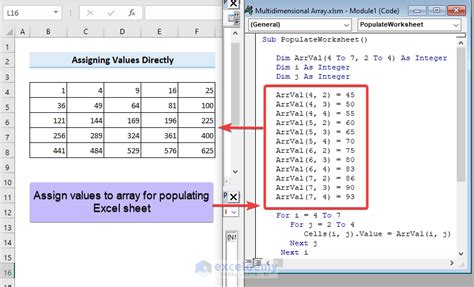
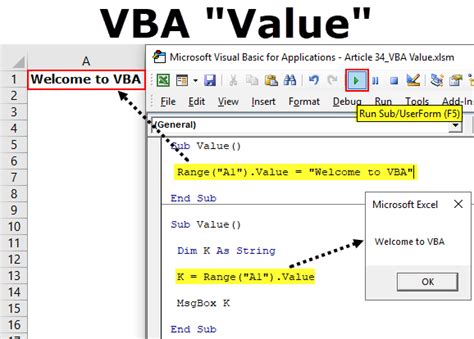
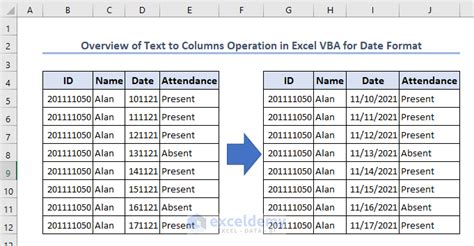
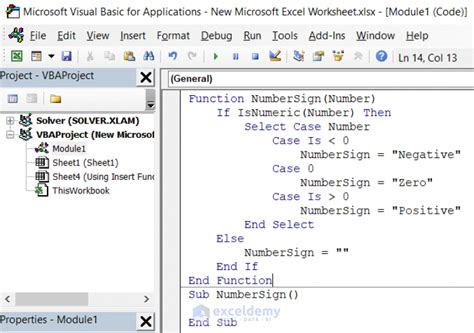
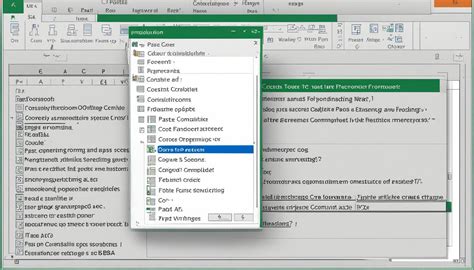
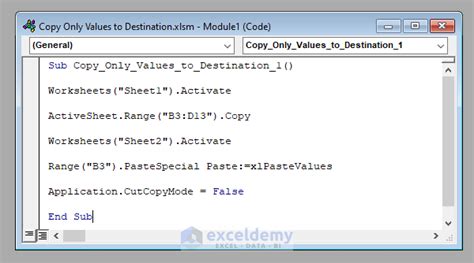
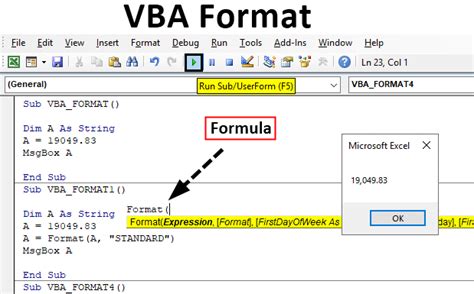
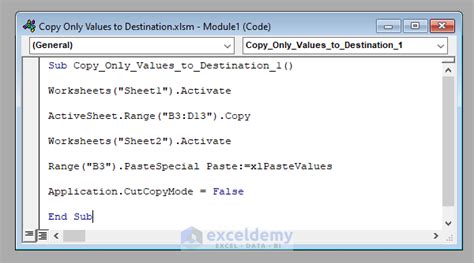
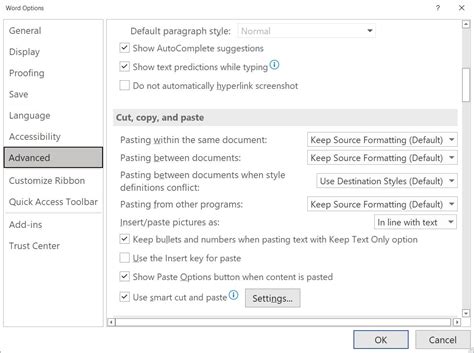
Conclusion and Further Reading
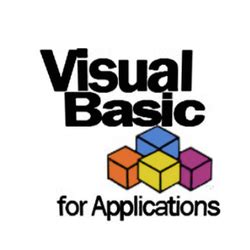
Pasting without formatting is a fundamental skill in VBA that can significantly improve your workflow efficiency. By mastering these five methods, you'll be well-equipped to handle a variety of data manipulation tasks in Excel. For further learning, consider exploring official Microsoft documentation, VBA forums, and Excel-specific blogs. Don't hesitate to experiment with different approaches to find what works best for your projects.
We'd love to hear from you! Share your favorite VBA tips or ask questions about pasting without formatting in the comments below. Don't forget to share this article with fellow Excel enthusiasts to spread the knowledge.